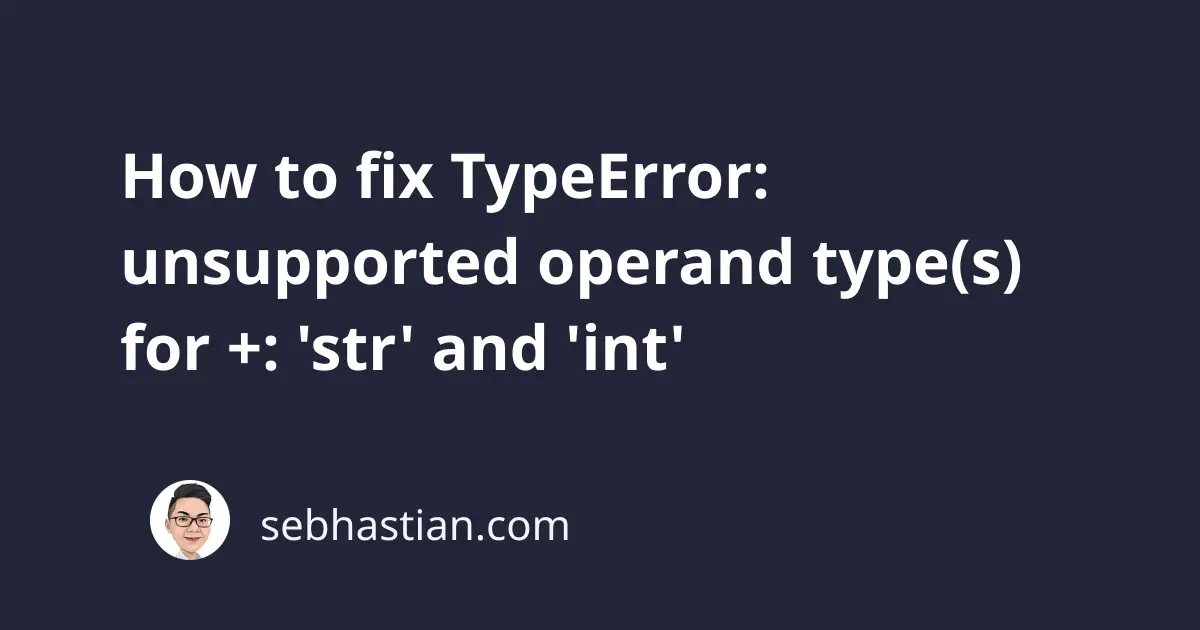
One error that you might encounter when programming with Python is:
TypeError: unsupported operand type(s) for +: 'str' and 'int'
This error occurs when you attempt to do an addition operation between strings and integers.
In Python, you can’t add string values to integer values and vice versa.
This tutorial will explain an example that causes this error and how you can fix it in practice.
How to reproduce this error
Suppose you use the input()
function to prompt the user for a specific number as follows:
x = input("Specify a number: ")
Next, you try to add that input number with another number as follows:
result = x + 3
print("The number added by 3:", result)
When you run the code above, you get this error:
Traceback (most recent call last):
File "main.py", line 3, in <module>
result = x + 3
TypeError: unsupported operand type(s) for +: 'str' and 'int'
This error occurs because the input()
function always returns the user input as a string.
Even when you prompt for a number, that number will be stored in the x
variable as a string.
Because Python adopts a strong typing system that allows operations only between the same type values, it raises this error.
How to fix this error
To resolve this error, you need to perform an addition operation between number types like integers and floats.
When you use the input()
function to ask for a number, you need to use the int()
function to convert the returned value to an integer:
x = int(input("Specify a number: ")) # ✅
The code above will assign an integer for the variable x
, allowing you to perform an addition operation to the variable.
Note that there are a number of similar unsupported operand type(s) errors in Python that points to the same problem.
For example, if you try to find the remainder of a string and an integer with the modulo %
operator:
TypeError: unsupported operand type(s) for %: 'int' and 'str'
Or when you try to subtract a string with an integer:
TypeError: unsupported operand type(s) for -: 'str' and 'int'
The solution to this error is to convert the values to integer type so you can do math operations with them. You can use the int()
function to do so.
Sometimes, you also get the unsupported operand type error when you use types that inherently don’t support the operation, such as using -
in strings and lists.
In this case, you need to learn what operations are supported for that specific types.
Conclusion
The Python TypeError: unsupported operand type(s) for +: 'str' and 'int'
occurs when you attempt to add a string with an integer together.
To resolve this error, you need to convert the string to an integer using the int()
function before performing the addition.
I hope this tutorial is helpful. Happy coding!