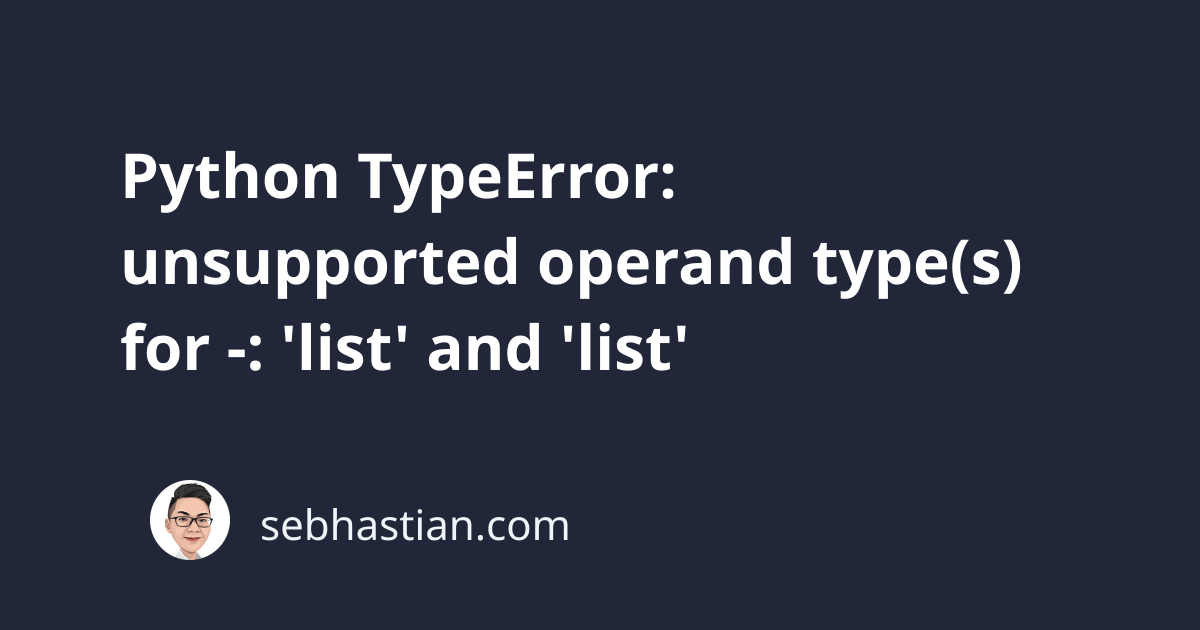
The Python TypeError: unsupported operand type(s) for -: 'list' and 'list'
message is triggered when you perform a subtraction operation on two lists.
The example code that triggers this error:
list1 = [2, 3]
list2 = [3, 2]
result = list1 - list2 # ❌
The code above produces the following error:
Traceback (most recent call last):
File ...
result = list1 - list2
TypeError: unsupported operand type(s) for -: 'list' and 'list'
In Python, the subtraction operator (-
) is not defined for lists, so subtracting one list from another will raise an error.
If you want to perform a subtraction, you can use a NumPy array instead:
import numpy as np
array1 = np.array([2, 3])
array2 = np.array([3, 2])
result = array1 - array2
print(result) # [-1 1]
If you want to get elements in list1
that are not present in list2
, you can use the list comprehension syntax.
Add an if
statement to filter out the elements that are in both lists as shown below:
list1 = [1, 2, 3]
list2 = [2, 3, 4]
result = [x for x in list1 if x not in list2]
print(result) # [1]
In the example above, the list comprehension iterates through list1
and filters out the elements that are also in list2
.
This way, the result
list will contain only elements from list1
that are not in list2
.
If you want to get elements that are in list2
and not in list1
, you need to switch the input lists:
list1 = [1, 2, 3]
list2 = [2, 3, 4]
result = [x for x in list2 if x not in list1]
print(result) # [4]
Finally, you can also use the set
type to get unique elements from one of the lists as follows:
list1 = [1, 2, 3]
list2 = [2, 3, 4]
result = set(list1) - set(list2)
# convert the result back to list
result = list(result)
print(result) # [1]
To conclude, the Python TypeError: unsupported operand type(s) for -: 'list' and 'list'
occurs because the subtraction operator can’t be used on two lists.
To fix this error, you can use the NumPy array type to perform subtraction between two arrays.
If you want to get unique elements from the list, use a list comprehension with an if
statement, or the set
type.
Good work solving this error! 👍