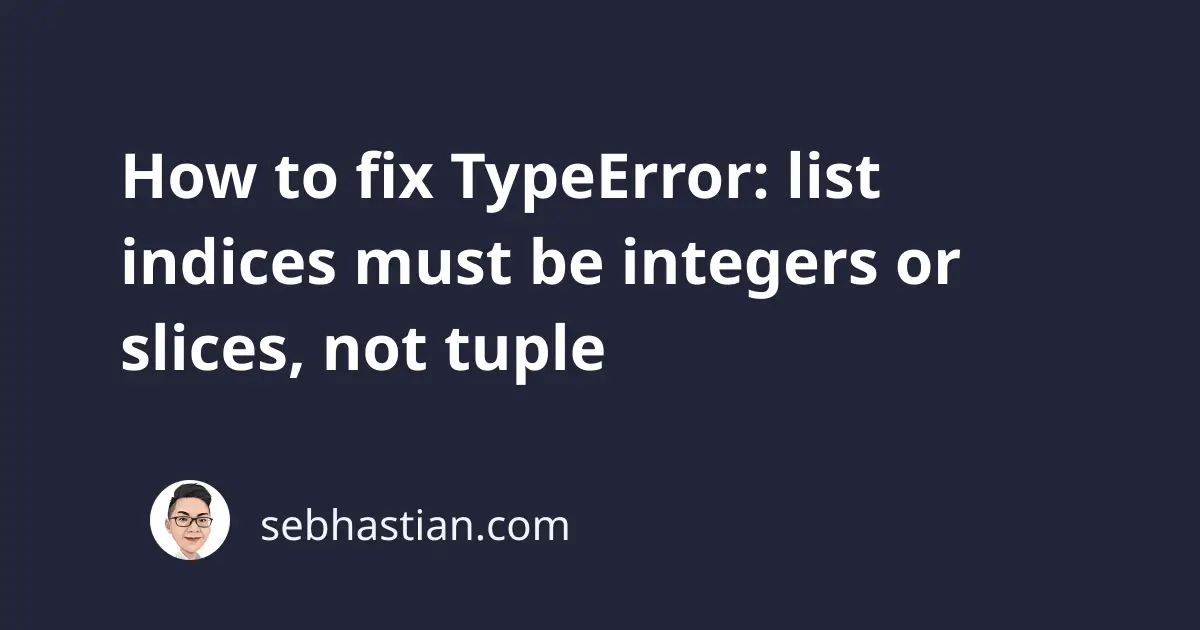
When you’re working with a list in Python, you might get the following error:
TypeError: list indices must be integers or slices, not tuple
This error occurs when you have an incorrect syntax in declaring or accessing a list. Python mistakenly thinks you’re trying to access a list using a tuple as the index position.
There are two possible scenarios that cause this error:
- You forget to separate nested lists with commas
- You access a list with a tuple
The tutorial below shows how to fix this error in each scenario.
1. You forget to separate nested lists with commas
Suppose you have a Python list of random numbers as follows:
my_list = [
[3, 4]
[5, 7]
[8, 9]
]
The my_list
above contains three pairs of nested lists, but the lists are not separated using a comma.
As a result, you get this error when you run the code:
Traceback (most recent call last):
File "main.py", line 2, in <module>
[3, 4]
TypeError: list indices must be integers or slices, not tuple
The error message might seem confusing because we’re not accessing any list, but it actually makes sense because of how Python works.
Python allows you to access a specific element from a list by specifying another square brackets next to the list declaration. Consider the code below:
x = ['Jane', 'Max'][1]
print(x) # Max
The subscript notation [1]
next to the list declaration allows you to access the list as if it was a variable name.
The following example is identical to the one above:
names = ['Jane', 'Max']
x = names[1]
print(x) # Max
A list indice (position index) can only be integers or slices. When you put more than one integer, Python thinks you’re passing a tuple, which causes the error.
How to fix this error
To resolve this error is very straightforward. You just need to separate the nested lists using a comma as follows:
my_list = [
[3, 4],
[5, 7],
[8, 9]
]
By adding the commas, Python knows that it’s a declaration of multiple child lists inside a list, so the error is now resolved.
2. You access a list with a tuple
The same error occurs when you try to access a list using a tuple. See the code example below:
names = ['Jane', 'Max', 'Amy']
x = names[0,2]
You’re putting two integers separated by a comma in the square brackets, so Python thinks you’re passing a tuple as the list index.
If you want to use a slice, you need to separate the numbers using a colon :
as shown below:
names = ['Jane', 'Max', 'Amy']
x = names[0:2]
print(x) # ['Jane', 'Max']
Notice that the slice now works and the error is resolved.
More resources
The following tutorials explain how you can fix other common errors when working with a list in Python:
TypeError: unsupported operand type(s) for -: ’list’ and ’list’
TypeError: list object cannot be interpreted as an integer
I hope these tutorials are helpful. Happy coding! 🙌