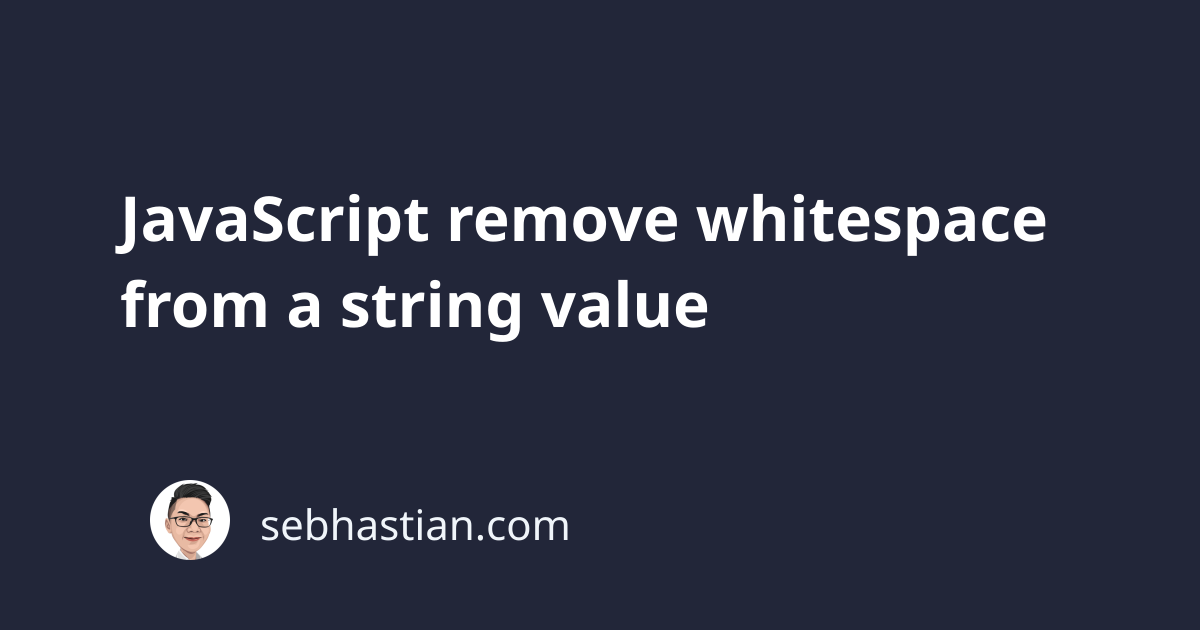
When you need to remove all whitespace from a JavaScript string
, you can use the trim()
method.
Here’s an example of trim()
in action:
let str = " Hello World! ";
let trimStr = str.trim();
console.log(trimStr); // "Hello World!"
The trim()
method doesn’t accept any parameter. It will remove all whitespace characters on either side of the original string.
The method returns a new string, so the original string won’t be affected.
Also, keep in mind that trim()
won’t remove whitespace in the middle of your string.
Consider the following example:
let str = " Hello Wo rld! ";
let trimStr = str.trim();
console.log(trimStr); // "Hello Wo rld!"
The whitespace characters between Wo
and rld!
are not removed in the above example.
If you want to remove whitespaces from between the characters too, you need to the combination of the split()
and join()
methods to your string as follows:
let str = " Hello Wo rld! ";
let trimStr = str.split(' ').join('');
console.log(trimStr); // "HelloWorld!"
Or you can also use the replace()
method and pass a regular expression to search whitespace characters and replace them with an empty string(''
).
Here’s how you do it:
let str = " Hello Wo rld! ";
let trimStr = str.replace(/\s+/g, '');
console.log(trimStr); // "HelloWorld!"
The /\s+/g
regex pattern will match all whitespace characters, and the replace()
method will do the removal.
And now you’ve learned how to remove whitespace characters using JavaScript trim()
and replace()
methods. Nice work!
The replace()
method is one of the most useful methods of the string object. It can also be used to format number digits with commas for easier reading.
I hope this tutorial has been useful for you. 🙏