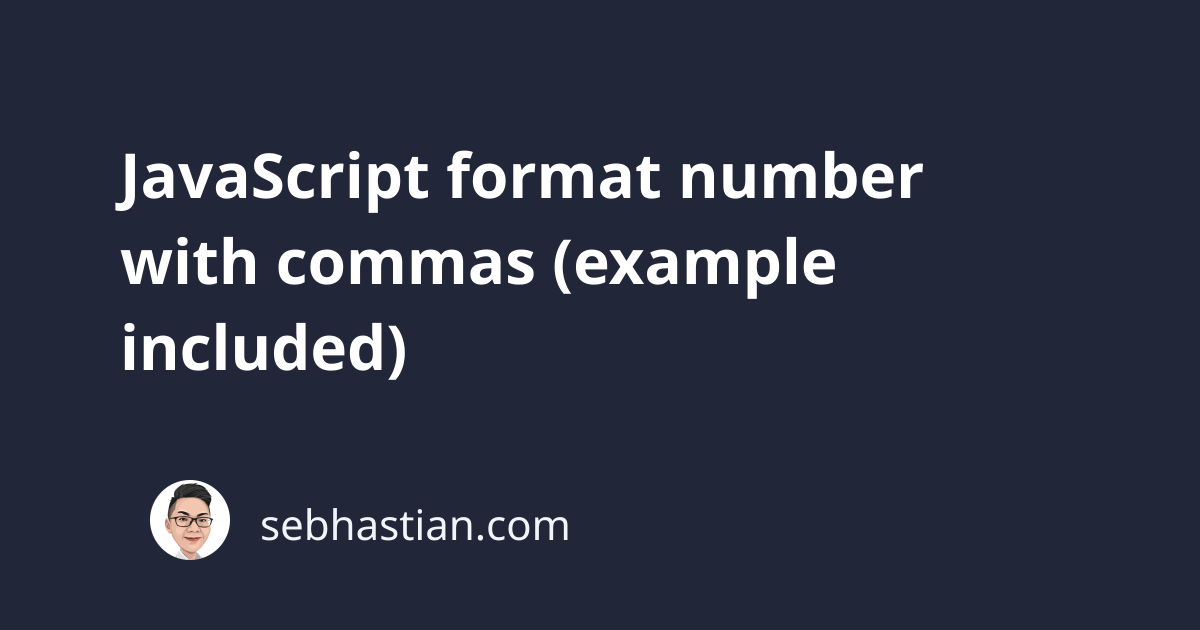
Sometimes, you may need to format a number value with commas in your HTML pages to make it easier to read.
You can transform a number value into a comma-separated string by using JavaScript. Here are two ways to do that:
Let’s learn both ways to format numbers next.
Using toLocaleString() method
The Number.toLocaleString()
method is a built-in method of the Number
object that returns a locale-sensitive string representing the number.
You can pass "en-US"
as the parameter so that it will always create a thousand separator for your number.
Here’s an example:
let n = 234234234;
let str = n.toLocaleString("en-US");
console.log(str); // "234,234,234"
The toLocaleString()
also works with floating numbers as follows:
let n = 234234.555;
let str = n.toLocaleString("en-US");
console.log(str); // "234,234.555"
But if you don’t want to use toLocaleString()
method, there’s also another way to format numbers with commas.
You can use the String.replace()
method and regex, which you will learn next.
Format number with commas using regular expressions
A Regular expression (regex) is a sequence of characters that specifies a search term.
By using regular expressions, you will be able to find and replace values in your string and format them in a way that you require.
For example, consider the following regex pattern:
/\B(?=(\d{3})+(?!\d))/g
The regex pattern above will search your string and put a marker when it finds 3 consecutive digits.
You can use the regex pattern in combination with String.replace()
to replace the markers with commas.
The following example shows how it works:
function numberWithCommas(num) {
return num.toString().replace(/\B(?=(\d{3})+(?!\d))/g, ',');
}
let n = numberWithCommas(234234.555);
console.log(n); // "234,234.555"
Conclusion
And those are two ways you can format a number with commas using JavaScript.
Formatting a number using regex and replace()
method is always faster than using toLocaleString()
because toLocaleString()
method needs to check out the localization (country) selected by your computer or JavaScript engine first before formatting the number.
When you’re handling a huge request to format numbers with commas, it’s probably better to use regex pattern and String.replace()
method instead of toLocaleString()
method.