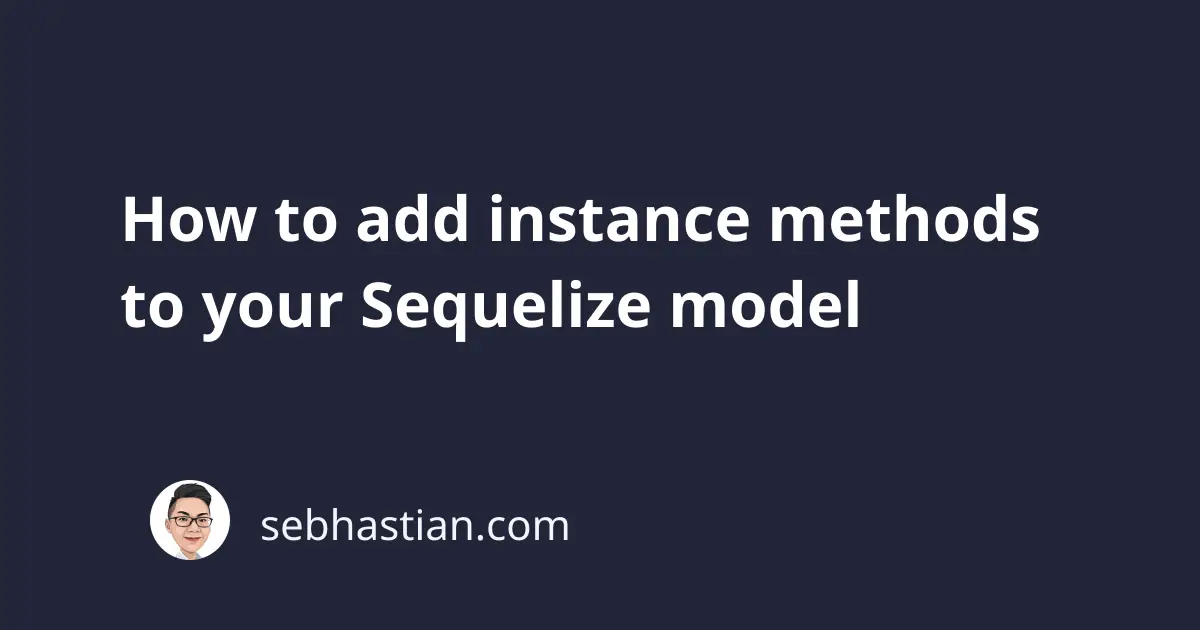
Sequelize allows you to define instance methods for your model.
Any instance method you define in the model can be called from the object returned by Sequelize query methods.
There are two ways you can define instance methods with Sequelize:
- Adding the function to the
prototype
object - Adding the function to the model created using ES6
class
This tutorial will help you learn both ways, starting with adding a prototype
function
Adding Sequelize instance method from the prototype object
To define an instance method without using ES6 classes, you need to add a new function to the model’s prototype
object.
The following example adds an instance method named testMethod()
to the User
model:
const User = sequelize.define("User", {
firstName: {
type: Sequelize.STRING,
},
isActive: {
type: Sequelize.BOOLEAN,
defaultValue: true,
},
},
{
timestamps: false,
});
User.prototype.testMethod = function () {
console.log("This is an instance method log");
};
The testMethod()
can then be called from the object returned by Sequelize query methods.
Below shows how the user
object returned from findOne()
method calls the testMethod()
:
const user = await User.findOne({ where: { firstName: "Nathan" } });
user.testMethod(); // This is an instance method log
Please note that when the findOne()
function doesn’t return any data row, then the call to instance method will throw a TypeError
as shown below:
user.testMethod();
^
TypeError: Cannot read properties of null (reading 'testMethod')
This is because the user
variable becomes null
when there is no row that matches the defined condition.
You can prevent this error by adding an if
statement as follows:
const user = await User.findOne({ where: { firstName: "Nathan" } });
if (user) user.testMethod();
This way, the call to testMethod()
will only be executed when the user
variable is not null
.
Sequelize instance method using ES6 class
Alternatively, you can also add a Sequelize instance method from an ES6 class-based model as shown below:
class User extends Model {
instanceMethod() {
console.log("This is an instance method log");
}
}
User.init(
{
firstname: Sequelize.STRING,
isActive: {
type: Sequelize.BOOLEAN,
defaultValue: false,
},
},
{ sequelize, timestamps: false }
);
Once you initialize the User
model with init()
call, you can call query methods from the model:
const user = await User.findOne({ where: { firstName: "Nathan" } });
if (user) {
user.instanceMethod(); // This is an instance method log
}
Now you’ve learned how to add instance methods to your Sequelize model. Nice work! 👍
Aside from instance methods, Sequelize also allows you to create class methods that you can call from the model directly.
Learn more about Sequelize class methods here.