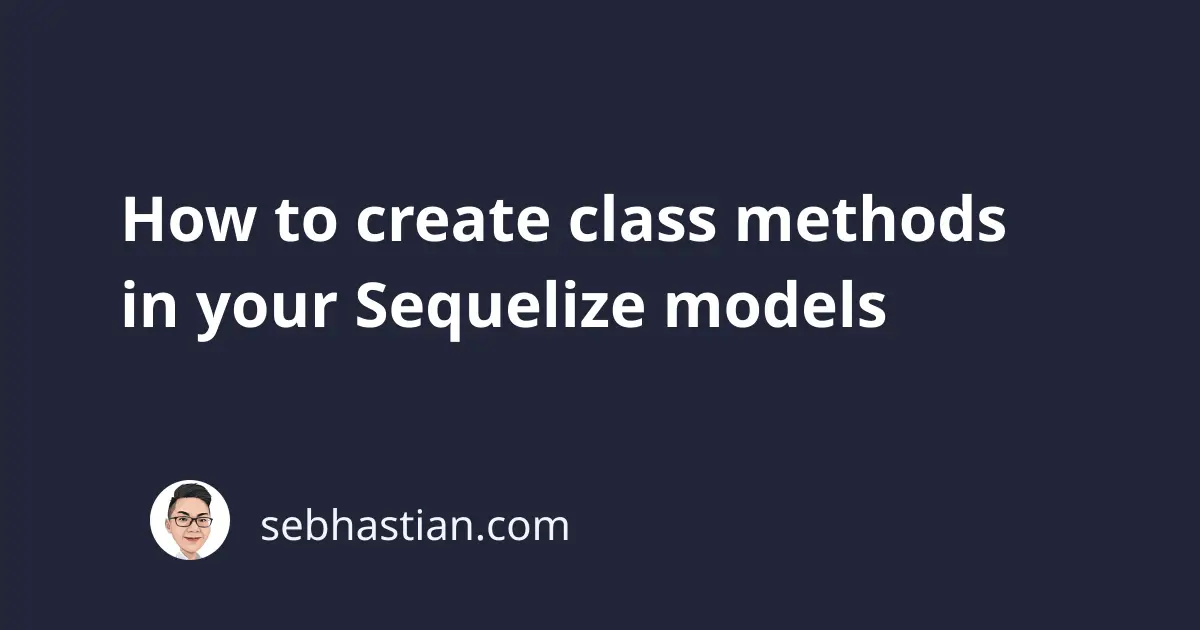
Sequelize allows you to add class-level methods to any defined models in your JavaScript code.
Usually, class-level methods are reserved for code that modifies the table, while instance methods are used for code that modifies your table rows.
For example, you can add association between different models as a class method as shown below:
const User = sequelize.define("User", {
firstName: {
type: Sequelize.STRING,
},
isActive: {
type: Sequelize.BOOLEAN,
defaultValue: false,
},
},
{
timestamps: false,
});
User.associate = function (models) {
User.hasMany(models.Task);
};
The above example creates a class method named associate()
in the User
model.
When called, the associate()
method will call the hasMany()
associate function, which will add the primary key of the User
model to the Task
model.
Once defined, you can call the method from the Model
object directly as shown below:
User.associate = function (models) {
User.hasMany(models.Task);
};
User.associate();
You are free to define the name and the body of your class method as you need.
To test a class method, you can add the following dummy function:
User.classMethod = function () {
console.log("Output from classMethod");
};
User.classMethod(); // Output from classMethod
Sequelize class methods in ES6 class models
If you use ES6 classes for defining your models, a class method can be created by defining a static
function inside your class.
The following example creates a static
function named classMethod()
that you can call from the initialized model directly:
class User extends Model {
static classMethod() {
console.log("This is a class level method");
}
}
User.init(
{
firstname: Sequelize.STRING,
isActive: {
type: Sequelize.BOOLEAN,
defaultValue: false,
},
},
{ sequelize, timestamps: false }
);
User.classMethod(); // This is a class level method
Before Sequelize v4, you can add both instanceMethods
and classMethods
options to your Sequelize model.
But since these options have been deprecated, the two new ways to create class methods are:
- Adding a function property to the model created from
sequelize.define()
- Adding a
static
function to aclass
that extends theModel
class
Now you’ve learned how to create class methods inside your Sequelize models. Great job! 👍