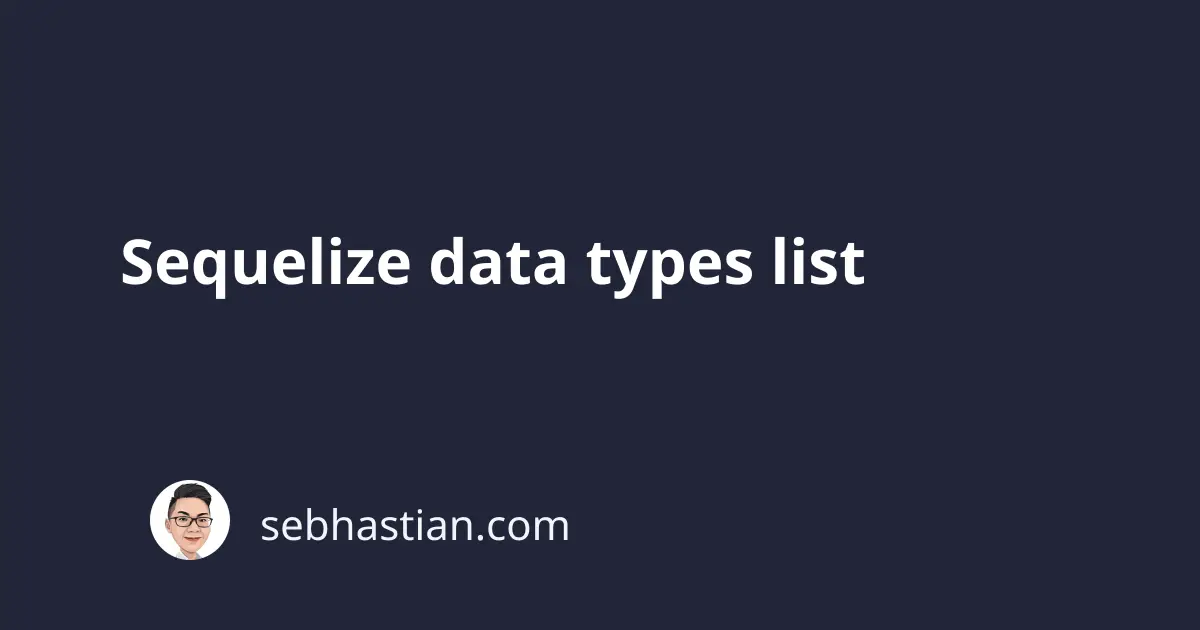
Sequelize provides support for most data types that you can find in SQL-based databases.
When defining types, you can use both Sequelize
class and DataTypes
class interchangeably as shown below:
const User = sequelize.define(
"User",
{
firstName: DataTypes.STRING,
lastName: Sequelize.STRING,
},
{
timestamps: false,
}
);
Some of the data types supported by Sequelize are as follows:
Sequelize.STRING // VARCHAR(255)
Sequelize.STRING.BINARY // VARCHAR BINARY
Sequelize.TEXT // TEXT
Sequelize.TEXT('tiny') // TINYTEXT
Sequelize.BOOLEAN // TINYINT(1)
Sequelize.INTEGER // INTEGER
Sequelize.BIGINT // BIGINT
Sequelize.BIGINT(11) // BIGINT(11)
Sequelize.FLOAT // FLOAT
Sequelize.FLOAT(11) // FLOAT(11)
Sequelize.FLOAT(11, 10) // FLOAT(11,10)
Sequelize.DOUBLE // DOUBLE
Sequelize.DOUBLE(11) // DOUBLE(11)
Sequelize.DOUBLE(11, 10) // DOUBLE(11,10)
Sequelize.DECIMAL // DECIMAL
Sequelize.DECIMAL(10, 2) // DECIMAL(10,2)
Sequelize also supports many types that are available only for specific vendors.
For MySQL and MariaDB, you can define unsigned or zero fill integers as shown below:
Sequelize.INTEGER.UNSIGNED
Sequelize.INTEGER.ZEROFILL
Sequelize.INTEGER.UNSIGNED.ZEROFILL // both unsigned and zero fill
You can use the unsigned or zero fill options for INTEGER
, BIGINT
, FLOAT
, and DOUBLE
types.
For PostgreSQL, the REAL
and RANGE
types are also supported:
Sequelize.REAL // REAL
Sequelize.REAL(11) // REAL(11)
Sequelize.REAL(11, 12) // REAL(11,12)
Sequelize.RANGE(Sequelize.INTEGER) // Defines int4range range.
Sequelize.RANGE(Sequelize.BIGINT) // Defined int8range range.
Sequelize.RANGE(Sequelize.DATE) // Defines tstzrange range.
Sequelize.RANGE(Sequelize.DATEONLY) // Defines daterange range.
Sequelize.RANGE(Sequelize.DECIMAL) // Defines numrange range.
Sequelize also has support for the date types.
Sequelize.DATE
is used for DATETIME
column, while Sequelize.DATEONLY
is used for DATE
column (no timestamp):
Sequelize.DATE // DATETIME for MySQL and SQLite, TIMESTAMP for Postgres
Sequelize.DATEONLY // DATE without time.
The TIMESTAMP
type will be used when you use the Sequelize.DATE
type with Postgres database.
If you want to use the TIMESTAMP
type for other databases, you need to manually specify the type of your Model attribute as a TIMESTAMP
.
In the following model definition, the registerDate
attribute is defined as a TIMESTAMP
type:
const User = sequelize.define(
"User",
{
firstName: Sequelize.STRING,
registerDate: {
type: "TIMESTAMP",
defaultValue: sequelize.literal("CURRENT_TIMESTAMP"),
},
},
{
timestamps: false,
}
);
You can learn more about the TIMESTAMP
type here:
Sequelize timestamps option and format explained
For the full list of available data types in Sequelize, you can check the Sequelize data types documentation.
Please note that any type you can call from the DataTypes
is also available from the Sequelize
class.
It’s more convenient to use the Sequelize
class because you already imported the class for creating a new Sequelize
instance anyway.