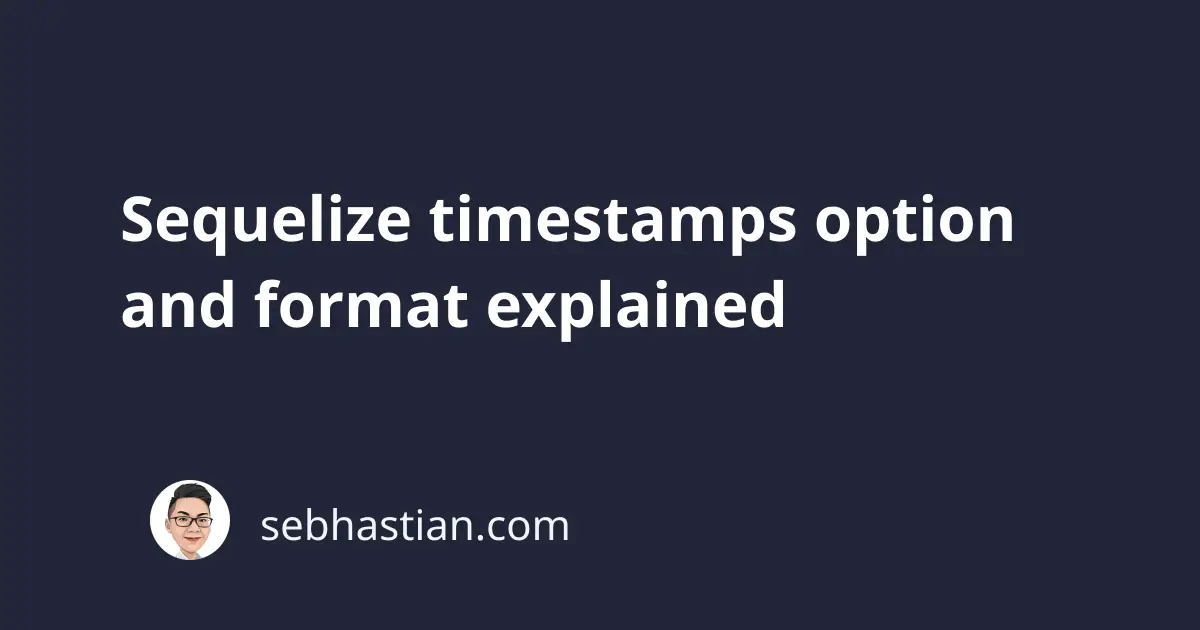
The timestamps
option in Sequelize models allows you to add two time-related attributes to the model.
The two attributes added to your model are createdAt
and updatedAt
attributes.
Consider the following User
model:
const User = sequelize.define(
"User",
{ firstName: Sequelize.STRING }
);
When you sync()
the above model, the generated CREATE TABLE
statement would be as shown below:
CREATE TABLE IF NOT EXISTS `Users` (
`id` integer NOT NULL AUTO_INCREMENT,
`firstName` varchar(255),
`createdAt` DATETIME NOT NULL,
`updatedAt` DATETIME NOT NULL,
PRIMARY KEY (`id`)
) ENGINE = InnoDB;
Notice how the table has two extra columns: createdAt
and updatedAt
columns of DATETIME
value.
The createdAt
column will store the timestamp of when a new row was inserted, while the updatedAt
column will store a timestamp of when the row was last updated.
The two columns are added to your model by the timestamps
option which defaults to true
.
If you don’t want the columns, you need to set the timestamps
option as false
when you define your model:
const User = sequelize.define(
"User",
{ firstName: Sequelize.STRING },
{ timestamps: false }
);
With timestamps: false
, the generated model will omit the createdAt
and updatedAt
attributes.
You can also opt to include only the timestamp attribute you need as shown below:
const User = sequelize.define(
"User",
{ firstName: Sequelize.STRING },
{
timestamps: true,
createdAt: false, // don't add createdAt attribute
updatedAt: true,
}
);
When you have timestamp columns in your SQL table with different names, you can add the column names as aliases to the createdAt
and updatedAt
attributes as shown below:
const User = sequelize.define(
"User",
{ firstName: Sequelize.STRING },
{
timestamps: true,
createdAt: "created_date", // alias createdAt as created_date
updatedAt: "updated_at", // alias updatedAt as updated_at
}
);
You may have already noted from the CREATE TABLE
statement that the createdAt
and updatedAt
columns created by Sequelize don’t have the DEFAULT
and ON UPDATE
options added.
This means that the columns are populated by values sent from Sequelize instead of the server calculating the values itself.
If you use the timestamps
option of Sequelize models, then you need to keep this in mind when you remove or switch Sequelize with another ORM library in the future.
Using TIMESTAMP instead of DATETIME type
Sequelize uses the DATETIME
type for the time-related attributes.
If you want to use the TIMESTAMP
type, then you need to manually specify the attributes as TIMESTAMP
types in your model as shown below:
const User = sequelize.define(
"User",
{
firstName: Sequelize.STRING,
createdAt: {
type: "TIMESTAMP",
defaultValue: sequelize.literal("CURRENT_TIMESTAMP"),
allowNull: false,
},
updatedAt: {
type: "TIMESTAMP",
defaultValue: sequelize.literal(
"CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP"
),
allowNull: false,
},
},
{
timestamps: false,
}
);
Syncing the above model will generate the following CREATE TABLE
statement:
CREATE TABLE IF NOT EXISTS `Users` (
`id` integer NOT NULL AUTO_INCREMENT,
`firstName` varchar(255),
`createdAt` timestamp NOT NULL
DEFAULT CURRENT_TIMESTAMP,
`updatedAt` timestamp NOT NULL
DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP,
PRIMARY KEY (`id`)
) ENGINE = InnoDB;
The defaultValue
option specified above will also add the DEFAULT
and ON UPDATE
options for the created columns.
Now you’ve learned how the timestamps
option works in Sequelize, as well as how to change the type from DATETIME
to TIMESTAMP
. Good work! 👍