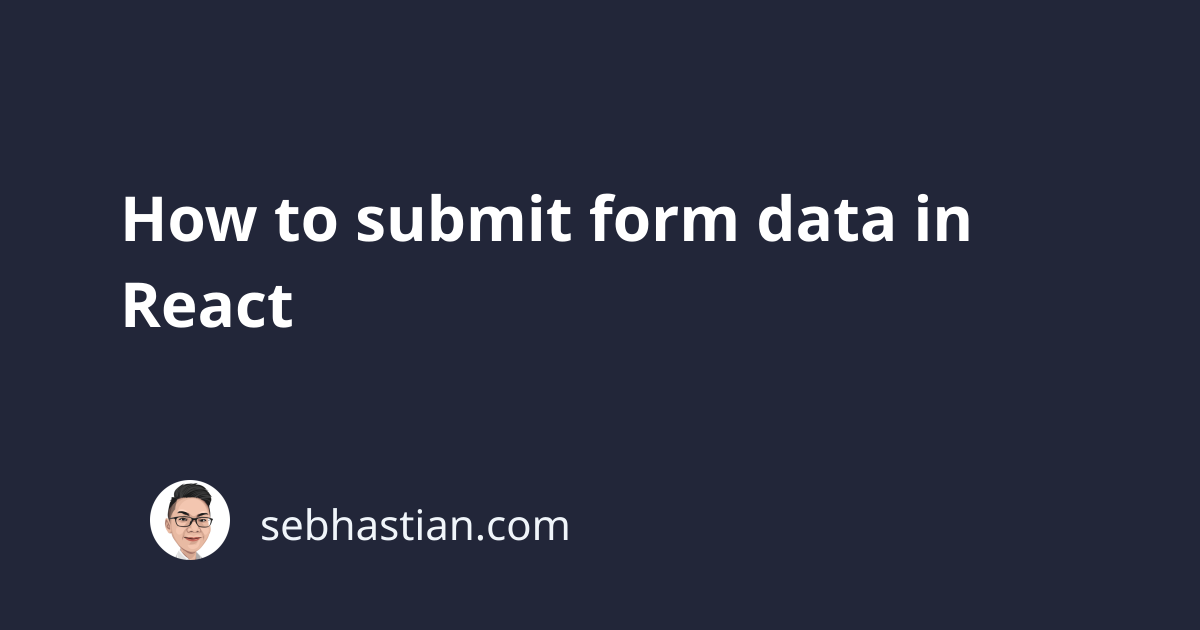
Unlike other JavaScript libraries, React doesn’t have any special way of handling a form submission. All you need to do is specify a custom function that gets called when user clicks on the submit button or press the Enter key. You need to pass the function into the <form>
element’s onSubmit
prop:
<form onSubmit={ /* your function here */ }>
What do you do when the user submits the form? It all depends on where you store your form data.
Generally, React recommends you to sync your form data with the component’s internal state through Controlled Components. When the user submits the form, you take those values and send it to the back-end service that validates and stores the data.
Here’s a complete example of a basic login form in React. Notice how the handleSubmit
function is passed to the onSubmit
prop:
function BasicForm(){
const [email, setEmail] = useState('')
const handleEmailChange = event => {
setEmail(event.target.value)
};
const handleSubmit = event => {
event.preventDefault();
alert(`Your state values: \n
email: ${email} \n
You can replace this alert with your process`);
};
return (
<form onSubmit={handleSubmit}>
<div>
<label>Email address</label>
<input
type="email"
name="email"
placeholder="Enter email"
onChange={handleEmailChange}
value={email}
/>
</div>
<button type="submit">
Submit
</button>
</form>
)
}
You can play the live demo in Code Sandbox.
Essentially, you need to cancel the form submission default behavior with preventDefault()
function and then describe the process you want React to do with your form.
If you keep the form data in state, then you can send the state data into the service that accepts it using an AJAX call. Put your form data in the body
of the request as a JSON string:
const handleSubmit = event => {
event.preventDefault();
const url = 'https://jsonplaceholder.typicode.com/posts'
const requestOptions = {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ email, password })
};
fetch(url, requestOptions)
.then(response => console.log('Submitted successfully'))
.catch(error => console.log('Form submit error', error))
};
You may even validate the data using an interactive form method before submitting it.