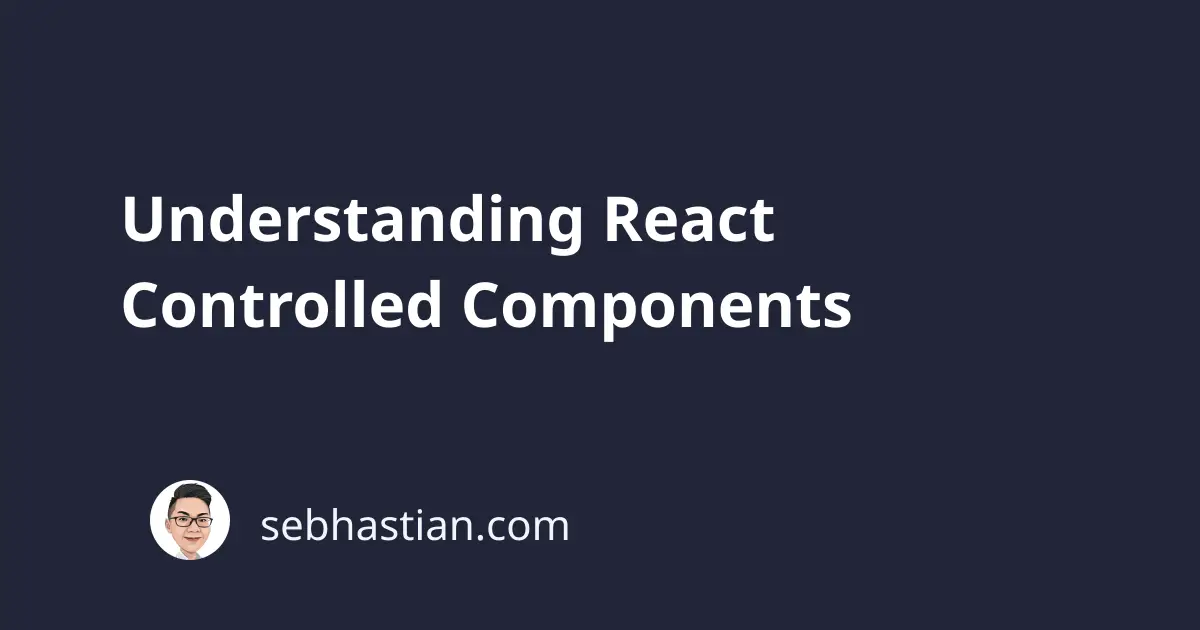
In React, controlled and uncontrolled component refers to the way JSX input elements store and get its form elements values.
A controlled component set and get its value from the state property. As you can see in the following component:
import React, { useState } from "react"
function App(props) {
const [name, setName] = useState('')
function handleChange(e) {
setName(e.target.value);
}
return (
<input
type="text"
name="name"
onChange={ handleChange }
value={name} />
)
}
In the example above, the <input>
element set its value from the name
state. When user changes the value of the input, the onChange
handler will be triggered and update the name
state with the target element’s value.
By letting React keep track of input values, you’re making the state as the “single source of truth” for your form data.
Now that you know what a controlled component is, understanding uncontrolled component is very easy. It refers to any component that doesn’t use React state to keep its value.
For example, you could still get JSX input values by using the browser’s Document selector:
import React, { useState } from "react"
function App() {
function getFirstNameValue() {
const nameValue = document.getElementById("name").value
console.log("Name input value:", nameValue)
}
return (
<>
<input type="text" id="name" />
<button onClick={getFirstNameValue}>
get input value
</button>
</>
)
}
Because React’s JSX elements are treated just regular HTML elements by the browser, you can simply query the value by using the same old getElementById
selector.
Making every single one of your input elements a controlled component can be very annoying. It requires you to write event handlers for every way your input value can change. After that, you also need to update your internal state to reflect the data changes.
React allows you to write uncontrolled components as an alternative to controlled components. Since uncontrolled component allows the DOM to be the source of truth, you can write forms in React with less code (quicker but looks dirtier).
React recommends you to always build forms using controlled components. But if you build your forms using other libraries and trying to integrate it with React, you might prefer using uncontrolled components.
You can then refactor your forms later to controlled components if you want to.