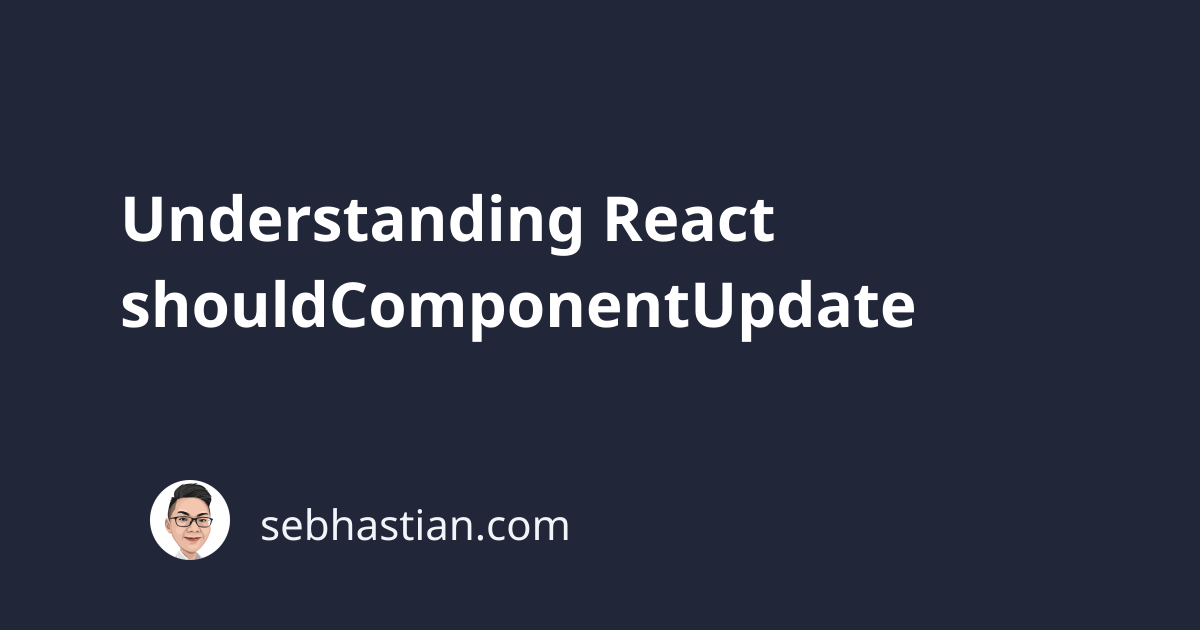
During React component’s lifecycle, there’s a set of specific methods that gets executed during a certain moment. These methods are called lifecycle methods, and shouldComponentUpdate
is a part of this method group.
This method is used to let your component exit the update lifecycle and prevent re-rendering of the component if there’s no meaningful change. The method will receive two arguments: the next props and state that are being received. You can then compare these data to the current data.
Here’s an implementation example:
shouldComponentUpdate(nextProps, nextState) {
if (this.props.color !== nextProps.color) {
return true;
}
if (this.state.count !== nextState.count) {
return true;
}
return false;
}
The method is run right after React internal state is updated but before the component re-renders and the update is reflected in the browser:
By default, this method is not implemented into React, and the most optimum implementation of this method can be found in React’s PureComponent API.
Should you use shouldComponentUpdate
Through my experience in creating React applications, I rarely encounter a case where the use of componentShouldUpdate
is justified. The reason is that React rendering execution is already fast by default. If you check on React’s performance. Most component render would take about 1 to 2 milliseconds. On a very complicated component with lots of computation, it might take React 10 milliseconds.
Let’s say you implemented shouldComponentUpdate
manually and you reduced React’s render time from 10 milliseconds to 1 milliseconds. You just created a 10x improvement, sure. But I bet you won’t feel any different at all. That’s because we’re humans, and we won’t be able to discern a significant improvement in load speed between 10ms and 1 ms.
In general, this method shouldn’t be used at all. Or if you really need it, you should implement Pure Component API so React can maintain it for you.