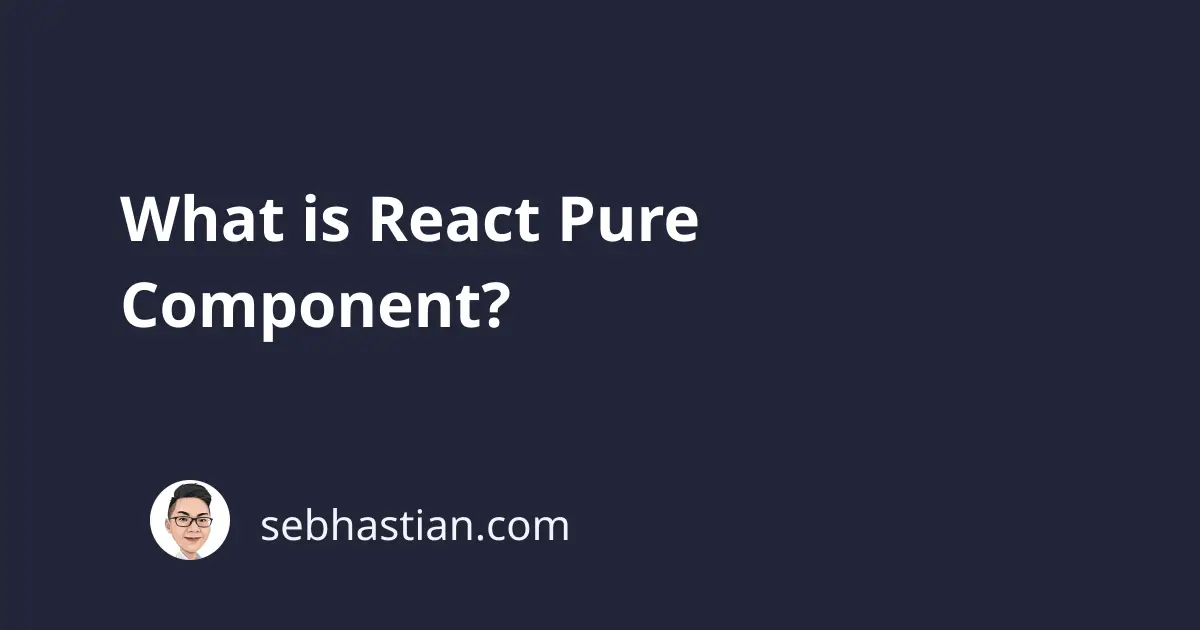
By default, React will always re-render the component each time there is a change in state or props value. A Pure Component is a component that performs a check on the value of React props before deciding whether to re-render the component or not. Let me show you by an example.
Class-based PureComponent
Imagine you have an application that displays a movie title and release status:
export default class App extends React.Component {
constructor(props) {
super(props);
this.state = {
title: "Titanic",
isReleased: true
};
}
toogleRelease = () => {
console.log("toogleRelease");
this.setState((prevState) => ({
isReleased: !prevState.isReleased
}));
};
render() {
const { title, isReleased } = this.state;
return (
<>
<MovieTitle title={title} />
<h1>Movie is released: {isReleased ? "yes" : "no"} </h1>
<button onClick={this.toogleRelease}>Toogle Release</button>
</>
);
}
}
Next, you have a MovieTitle
component with the following content:
class MovieTitle extends React.Component {
render() {
console.log("MovieTitle is rendered");
return <h1>Movie title {this.props.title} </h1>;
}
}
Here’s a running demo.
When you change the isReleased
value by click on the button, you’ll find that MovieTitle
gets re-rendered because React will print the log to the console. But actually, MovieTitle
component doesn’t need to re-render because even if the value of isReleased
is changed, its output remains the same.
To prevent the re-rendering and optimize your React app performance, you can extends
the PureComponent
class instead of Component
:
class MovieTitle extends React.PureComponent {
}
Now when you click on toogle release, you won’t see React printing the log because MovieTitle
will check the props and determine whether to render or not.
function-based PureComponent
You can do the same with function-based components, but instead of extending the PureComponent
class, you need to wrap the function component with React.memo()
, which memoize (cache) the output of the component.
The function will return a memoized component that you can call from other components:
function MovieTitle(){
}
const MemoizedMovie = React.memo(MovieTitle)
Now you just need to call the memoized MovieTitle
inside App
component:
render() {
return (
<MemoizedMovie title={title} />
);
}
React’s “pure component” is a performance optimization method which implements the shouldComponentUpdate
method underneath, but you should only use it sparingly, or even none at all.
This is because the performance optimization gained from using pure components are very trivial. React already re-render components very fast (as in less than 100 milliseconds fast, mostly around 20ms in small applications)
When you use pure components, comparing states and props will consume memory storage.
There will be a little delay because React has to compare the states and props before deciding whether to render or not. When React doesn’t re-render the component, you will gain a few milliseconds. When React does re-render, you will be slowed by a few milliseconds. Most people won’t be able to tell the difference anyway.