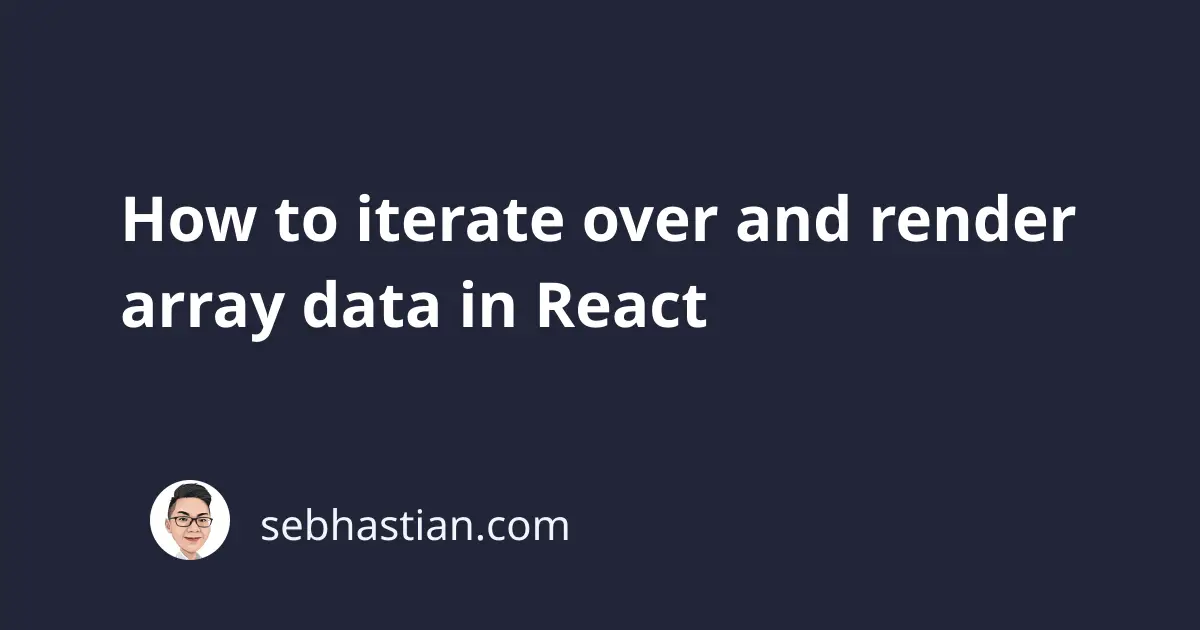
In order to iterate over an array of data using React, you need to learn about JavaScript’s map()
function first.
Understanding JavaScript’s map function
The map()
function is a special JavaScript array function that iterate over an array, allowing you to operate on each element of the array using a callback function.
Here is an example of the map function in action:
const numbers = [1,2,3]
const newNumbers = numbers.map(function(number){
return number+1
});
//newNumbers value is [2,3,4]
In the example, the map function returns a new array with values populated from the function result. You are free to define the callback function that map will call on each element of the array. Map will always pass on three parameters into the function:
- currentValue: the value of the current element under map operation
- index: the index of the current element under map operation
- array: the array under map operation, or the one you call map on
Since we have an array with 3 elements, the callback function will be called 3 times, once for each element:
const numbers = [1,2,3]
const newNumbers = numbers.map(function(currentValue, index, array){
// currentValue will return 1, then 2, then 3
// index will return 0, then 1, then 2
// array will always return [1,2,3]
return currentValue+1
});
Now that you know how a map function works, let’s get back to how you can iterate over an array in React.
Using map function inside React JSX
Inside a React component, you can use the map function to iterate over an array of data and return a JSX element that renders that will be rendered by React.
Let’s say you have an array of users that you’d like to render:
const users = [
{ id: 1, name: 'Nathan', role: 'Web Developer' },
{ id: 2, name: 'John', role: 'Web Designer' },
{ id: 3, name: 'Jane', role: 'Team Leader' },
]
Here is how you can do it inside your React component:
import React from "react"
function App() {
const users = [
{ id: 1, name: 'Nathan', role: 'Web Developer' },
{ id: 2, name: 'John', role: 'Web Designer' },
{ id: 3, name: 'Jane', role: 'Team Leader' },
]
return (
<>
<h1>Application</h1>
<p>The currently active users list:</p>
<ul>
{
users.map(function(user){
// returns Nathan, then John, then Jane
return <li> {user.name} as the {user.role} </li>
})
}
</ul>
</>
)
}
export default App
Inside React, you don’t need to store the return value of the map function in a variable. The example above will return a list element for each array value into the component.
While the above code is already complete, React will trigger a warning that you need to put “key” prop in each child of a list (the element that you return from map function):
The “key” prop is a special prop that React will use to determine which child element have been changed, added, or removed from the list. you won’t use it actively in any part of your array rendering code, but since React needs the props, then let’s give it.
It is recommended that you put the unique identifier of your data as the key value. In the example above, you can put the id
of each user as the key of each <li>
element:
return <li key={user.id}> {user.name} as the {user.role} </li>
When you don’t have any unique identifiers for your list, you may use the array index as the last resort:
{
users.map(function(user, index){
return <li key={index}> {user.name} as the {user.role} </li>
})
}
And that’s how you iterate over an array in React 🤓
What about for loops?
Although you can do for loops inside React components body, you can’t do a for loop in JSX because JSX only allows you to embed JavaScript expressions, while for
is a JavaSript statement.
I’ve written about using for loops with React here. So you can consider the differences.