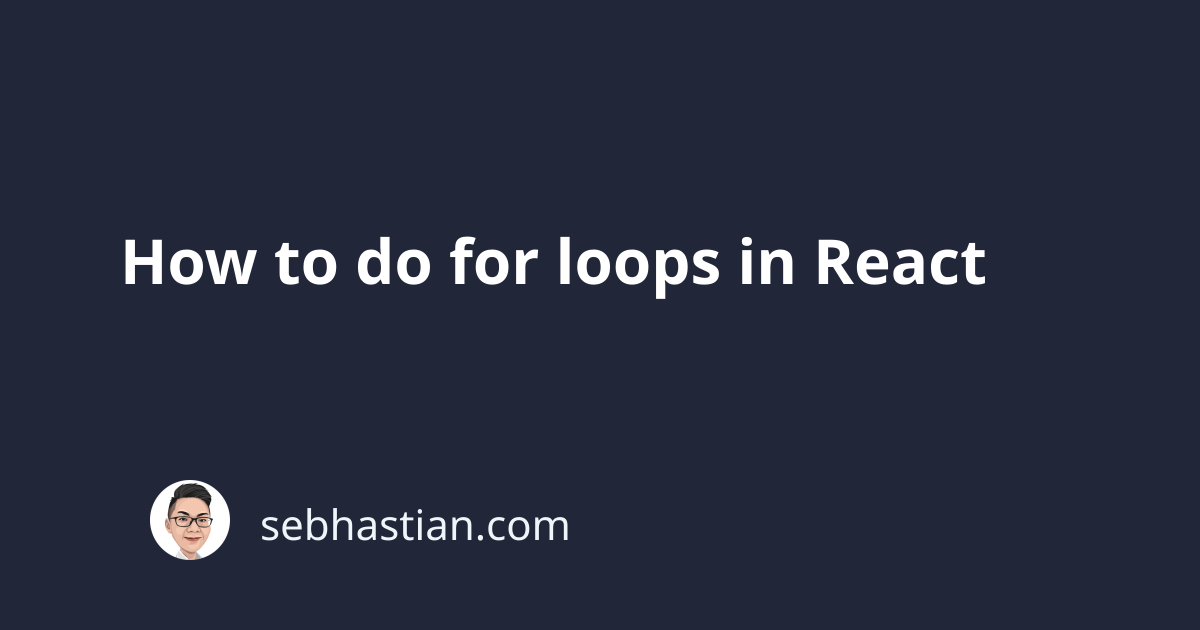
When you have multiple items that you want to render inside your React component, you can use a for
statement inside your function component to loop over the array. Inside the statement, you need to store each value inside a <li>
element, which you store in a new array.
Here’s an example:
function App() {
const names = ["Jessy", "James", "Jack"];
const items = [];
for (let i = 0; i < names.length; i++) {
items.push(<li>{names[i]}</li>);
}
return <ul>{items}</ul>;
}
JSX is smart enough to render the value of the array without you telling it to. You can also use other for loops like for...in
or for...of
in React components:
function App() {
const names = ["Jessy", "James", "Jack"];
const items = [];
for (const value of names) {
items.push(<li>{value}</li>);
}
return <ul>{items}</ul>;
}
But there is an easier, and also considered the correct way to loop over arrays or objects in React, and that is by using the map()
function.
Learn how you can use JavaScript’s map function with React here.