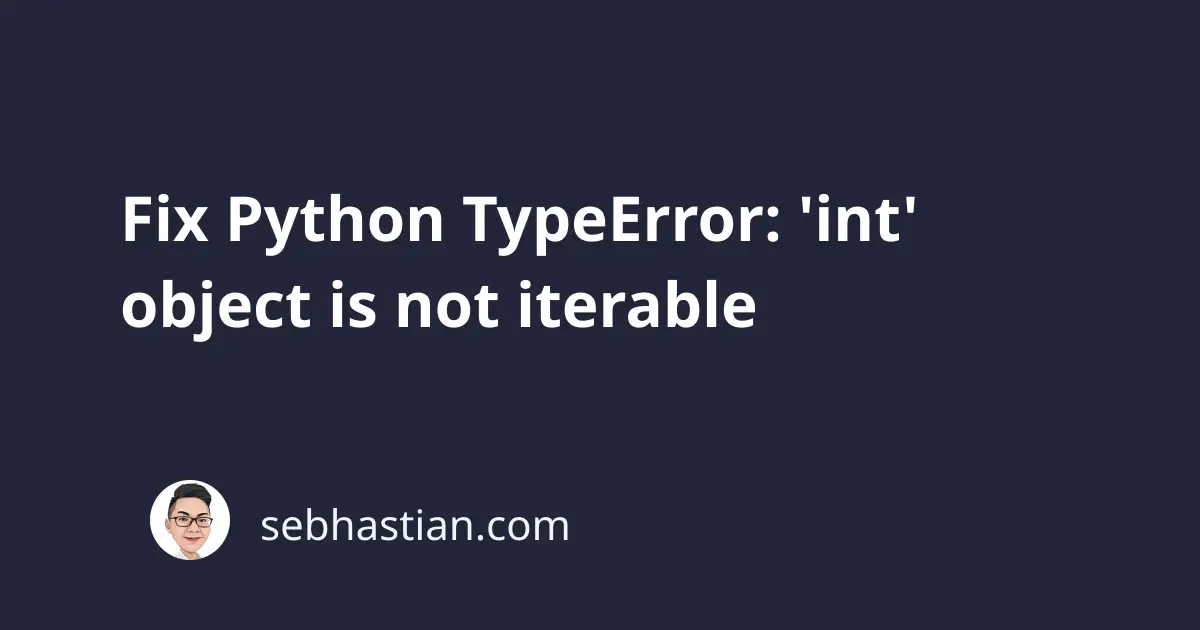
The Python TypeError: 'int' object is not iterable
error occurs when you try to use an iterable function or construct, such as a for
loop or a list comprehension, on an object of type int
.
To fix this error, add an if
statement to ensure that you’re not passing an int
when an iterable object is expected. This article will show you how.
In Python, an iterable is an object capable of returning its members one at a time, rather than returning the object itself.
Examples of iterable objects include lists, tuples, and strings.
However, integers are not iterable, and attempting to iterate over them will raise a TypeError.
Here’s an example of code that would trigger this error:
number = 7
# ❌ TypeError: 'int' object is not iterable
for item in number:
print(item)
Running the code above will give the following response:
The same error also occurs when you iterate using the list comprehension syntax:
number = 5000
# ❌ TypeError: 'int' object is not iterable
new_list = [digit for digit in number]
To fix this TypeError, you need to add a conditional if
statement and run a for
statement only when the variable is not an int
.
The following code should work:
number = [1, 2, 3]
if isinstance(number, int):
print("Can't iterate over an integer")
else:
for item in number:
print(item)
The code above checks if the number
variable is an instance of the int
class.
The isinstance()
function returns a boolean value that indicates whether the variable is an instance of the type you passed as its second argument.
The for
loop is defined inside the else
block, so it runs only when the variable is not an int
.
Alternatively, you can also use a try .. except
block to catch the TypeError that occurs when the variable is not an iterable object.
Consider the code below:
numbers = 50000
try:
for item in numbers:
print(item)
except TypeError:
print("Error: 'numbers' must be an iterable.")
The code above runs the for
loop inside a try
block. When the code causes a TypeError, Python will run the except
block and print a message to the console.
Using a try .. except
block is recommended because the int
type is not the only non-iterable type in Python. The None
value is also not iterable.
Conclusion
In summary, Python responds with TypeError: 'int' object is not iterable
when you try to iterate over an int
value using a for
loop or list comprehension syntax.
To fix this error, you need to adjust your code with an if
statement or a try .. catch
block so that the iterable function or construct doesn’t run on an int
value.