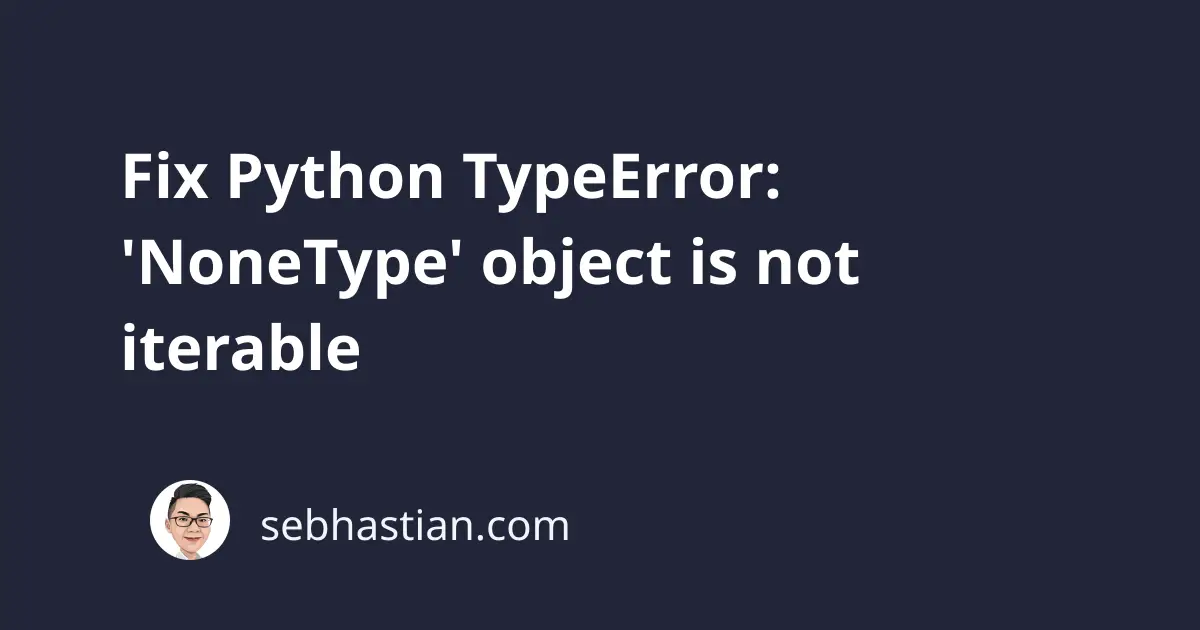
Python shows TypeError: 'NoneType' object is not iterable
message when you iterate over a None
value using a for
loop.
To fix this error, you need to make sure you do not put a None
object in your for
statement.
In Python, an iterable is an object capable of returning its members one at a time, rather than returning the object itself.
Examples of iterable objects include lists, tuples, and strings:
# List is an iterable ✅
items = [1, 2, 3]
for item in items:
print(item)
# Tuple is an iterable ✅
items = (1, 2, 3)
for item in items:
print(item)
# String is an iterable ✅
items = "abc"
for item in items:
print(item)
These examples show how you can use a for
loop to iterate over the members of a list, tuple, or string.
On the other hand, the NoneType
is a type that represents the absence of a value, and it is not iterable.
When you run the following code:
items = None
# ❌ TypeError: 'NoneType' object is not iterable
for item in items:
print(item)
Python responds with the following error:
To avoid this error, you need to adjust your code so that you don’t pass a None
value to the for
statement.
You can use an if
statement to check if the variable value is None
as shown below:
items = None
if items is None:
print('Error: items is not iterable')
else:
for item in items:
print(item)
The code above checks if the items
variable is None
and it only executes the for
loop when the variable is not None
.
Alternatively, you can use the try .. except
block to handle the TypeError when it occurs as shown below:
items = [1, 2, 3]
try:
for item in items:
print(item)
except TypeError:
print("Error: 'items' must be an iterable.")
The example above is recommended because the None
value is not the only object that’s not iterable.
This allows you to handle ‘int’ object is not iterable error as well.
You can also use the iter()
function to check whether an object is iterable or not in Python.
The iter()
function returns an iterator object from a value, but it will raise a TypeError when the value is not iterable:
items = [1, 2, 3]
try:
iterator = iter(items)
for item in iterator:
print(item)
except TypeError:
print("Error: 'items' must be an iterable.")
Although the try .. except
block works the same, calling the iter()
function ensures that the error happens exactly because the variable is not iterable.
Conclusion
To conclude, the Python TypeError: 'NoneType' object is not iterable
error happens when you try to iterate over a None
value.
To fix this error, adjust your code so that you don’t pass a None
value to the for
statement.
This can be done by using an if
statement to check for a None
value. You can also use a try .. except
block to catch the TypeError.