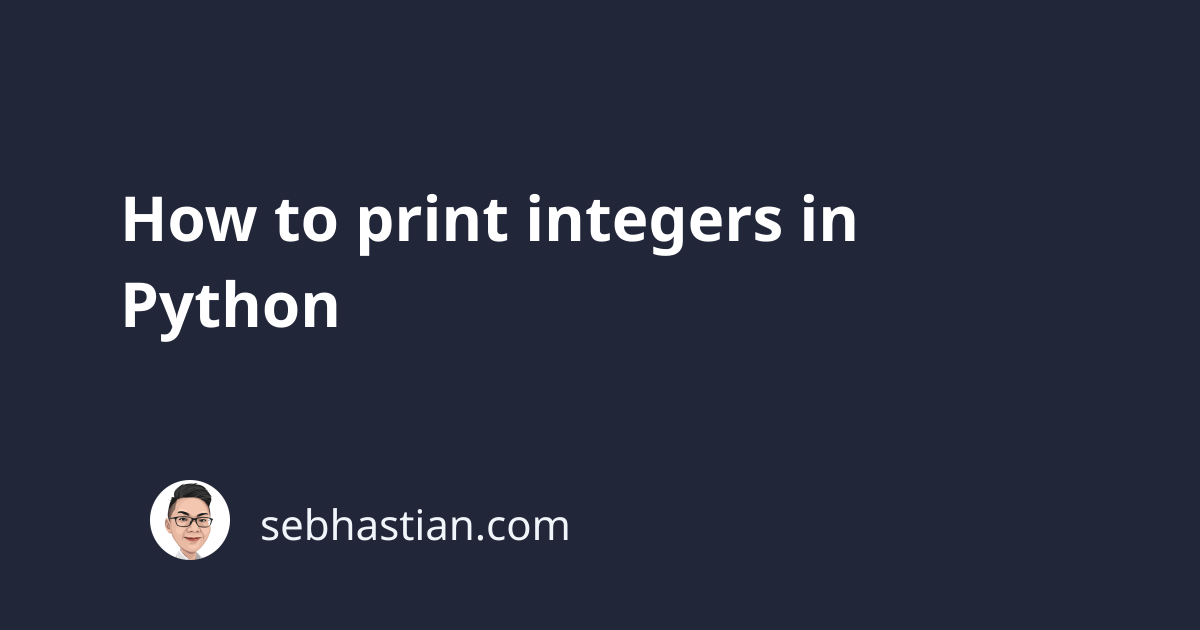
There are 4 ways you can print integers in Python:
- Using the
print()
function - Using the f-string format when you have a string
- Using the
int()
function when you have a string of numbers - Using the
str()
function when you need to print alongside a string
Let’s see how to use these 4 solutions in practice next.
1. Using the print()
function
When you have integers in your code, you can print them using the print()
function just like strings:
my_int = 899
print(my_int) # 899
2. Using the f-string format when you have a string
When you need to print an integer alongside a string, you can use the f-string format to get them printed without any error.
You add a literal f
before the string, then wrap the integer inside curly brackets {}
as shown below:
my_int = 899
print(f'The value is: {my_int}')
# The value is: 899
The f-string format automatically converts the integer into a string, allowing the print function to work.
3. Using the int()
function when you have a string of numbers
When you have a string of numbers, you can call the int()
function to convert that value:
my_number = '899'
print(int(my_number)) # 899
The variable my_number
will be printed as an integer because you called the int()
function inside print()
.
4. Using the str()
function when you need to print alongside a string
If you want to print the integer with some strings, you need to convert the integer value to a string so that Python can concatenate the values:
my_int = 899
print('The value is: ' + str(my_int))
# The value is: 899
Without calling the str()
function, you’ll get an error saying ‘can only concatenate str (not “int”) to str’.
And those are the 4 solutions you can use to print integers in Python. I hope this tutorial is useful.