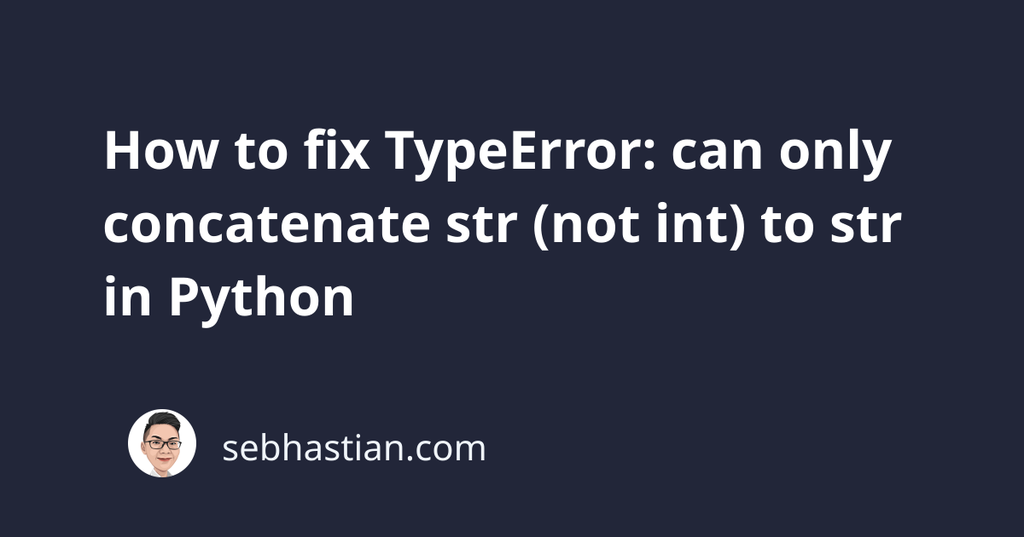
One error you might get when running Python code is:
TypeError: can only concatenate str (not "int") to str
This error occurs when you try to add (concatenate) a string type str
object with an integer type int
object.
This tutorial will show you an example that causes this error and how I fix it in practice.
How to reproduce this error
Suppose you try to print an integer and a string in one print()
function as shown below:
result = 5 + 10
print("The result is: " + result)
Output:
Traceback (most recent call last):
File "main.py", line 3, in <module>
print("The result is: " + result)
TypeError: can only concatenate str (not "int") to str
Python doesn’t allow joining a string with an integer. If you want to join values of different types, you need to make them the same type first.
The error can also occur when you have a string of number and and integer as follows:
result = 5 + 10
print("3" + result)
Output:
Traceback (most recent call last):
File "main.py", line 3, in <module>
print("3" + result)
TypeError: can only concatenate str (not "int") to str
The number ‘3’ in the example above is an str
type, so it can’t be joined with an int
type.
How to fix this error
To resolve this error, you need to either convert the string to integer, or the integer to string.
This example converts the integer to string:
result = 5 + 10
print("The result is: " + str(result))
Output:
The result is: 15
Here’s another example that converts the string to integer:
result = 5 + 10
print(int("3") + result)
Output:
18
When you’re printing the values of a dictionary, you can also get this error when you have integer values as either keys or values.
item_price = {
"Apple": 2,
"Banana": 3,
"Watermelon": 6.3,
}
for item, price in item_price.items():
print("Name: " + item + ", Price: $" + price)
Output:
Traceback (most recent call last):
File "main.py", line 8, in <module>
print("Name: " + item + ", Price: $" + price)
TypeError: can only concatenate str (not "int") to str
To resolve this error, you can use the str()
function to convert price to string:
print("Name: " + item + ", Price: $" + str(price))
Or you can also use the f-string format like this:
item_price = {
"Apple": 2,
"Banana": 3,
"Watermelon": 6.3,
}
for item, price in item_price.items():
print(f"Name: {item}, Price: ${price}")
The f-string format automatically converts values of another type to string when you run the code.
Conclusion
The TypeError: can only concatenate str (not "int") to str
occurs when you attempt to add a string and an integer.
Python doesn’t allow adding values of different types together, so you need to convert one of the values to make them the same type.
I hope this tutorial helps. See you in other tutorials!