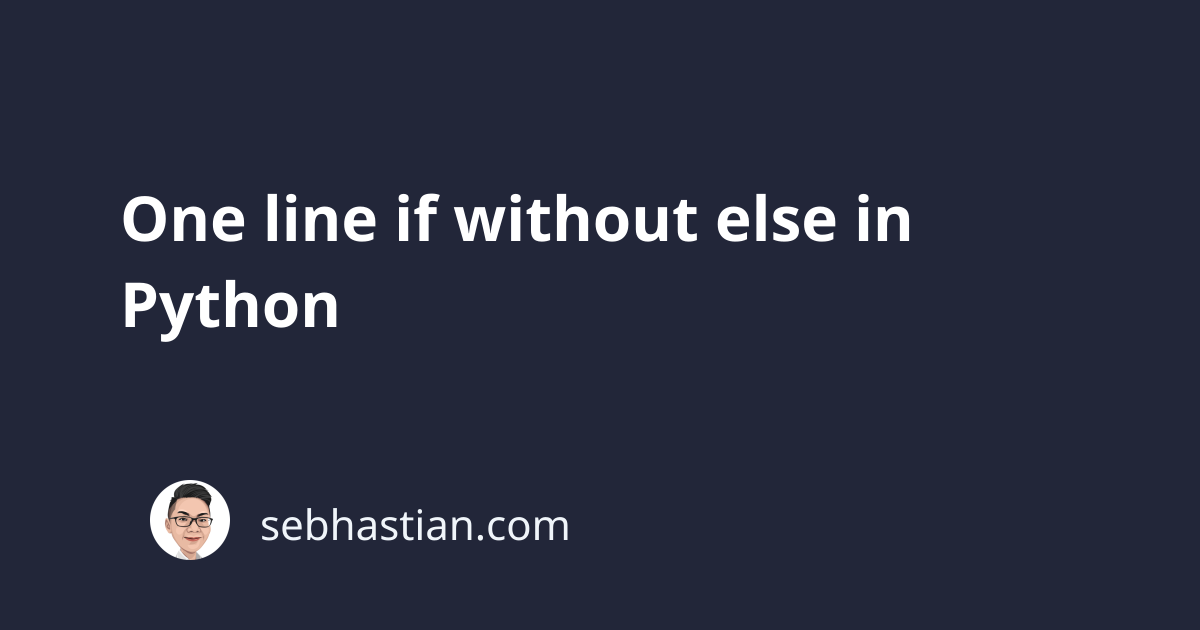
If you don’t have an else
statement, then you can write a one line if
statement in Python by removing the line break as follows:
if condition: a
There are 3 different ways you can create a one line if
conditional without an else
statement:
- The regular
if
statement in one line - Using the ternary operator syntax
- Shorthand syntax with the
and
operator
This tutorial shows you examples of writing intuitive one line if
statements in practice.
1. One line if statement
In the following example, the if
statement prints something if x
value is greater than 10
:
x = 20
if x > 10:
print("More than 10!")
Now here’s how you convert the code into one line if
statement:
x = 20
if x > 10: print("More than 10!")
Yup! Just remove the line break and you’re good to go.
If you have more than one line in the if
body, you can use a semicolon to mark the end of the statements. See the example below:
x = 20
if x > 10:
print("More than 10!")
print("Process finished")
# Equivalent one liner:
x = 20
if x > 10: print("More than 10!"); print("Process finished")
But keep in mind that this code violates Python coding conventions by defining multiple statements in one line.
It’s also harder to read when you have complex statements inside the if
body. Still, the code works well when you have simple conditionals.
2. Ternary operator syntax without else
The Python ternary operator is used to create a one line if-else
statement.
It comes in handy when you need to write a short and simple if-else
statement as follows:
x = 4
print("Good!") if x > 10 else print("Bad!")
You can read this article to learn more about how ternary operators work in Python.
You can use the ternary operator to write a one line if
statement without an else
as follows:
x = 20
print("Good!") if x > 10 else None
By returning None
, Python will do nothing when the else
statement is executed.
Although this one line if
statement works, it’s not recommended when you have an assignment in your if
body as follows:
x = 20
y = 5
if x > 10:
y = 50
Using the ternary operator, you still need to assign the original value of y
in the else
statement as follows:
x = 20
y = 5
y = 50 if x > 10 else y
The ternary operator doesn’t allow you to discard the else
statement, so this code redundantly assigns the value of y
to y
in the else
statement.
It’s better to use a regular if
statement as follows:
x = 20
y = 5
if x > 10: y = 50
Using a regular if
statement has less repetition and easier to read.
3. Shorthand syntax with the and
operator
The and
operator can be used to shorthand an if
statement because this operator executes the second operand only when the first operand returns True
.
Suppose you have an if
statement as follows:
x = 20
if x > 10:
print("More than 10!")
You can use the and
operator to rewrite the code above as follows:
x = 20
x > 10 and print("More than 10!")
The print()
function above is only executed when the expression on the left side of the and
operator returns True
.
But this method also falls short when you need to do an assignment as it will raise an error:
x = 20
y = 5
x > 10 and y = 50 # ❗️SyntaxError: cannot assign to expression
If your conditional involves an assignment, then you need to use the regular if
statement.
Conclusion
This tutorial has shown you examples of writing a one line if
without else
statement in Python.
In practice, writing a one line if
statement is discouraged as it means you’re writing at least two statements in one line: the condition and the code to run when that condition is True
.
Just because you can, doesn’t mean you should. Writing a regular if
statement with line breaks and indents provides you with a clean and consistent way to define conditionals in your code.
I hope this tutorial helps. Happy coding! 👍