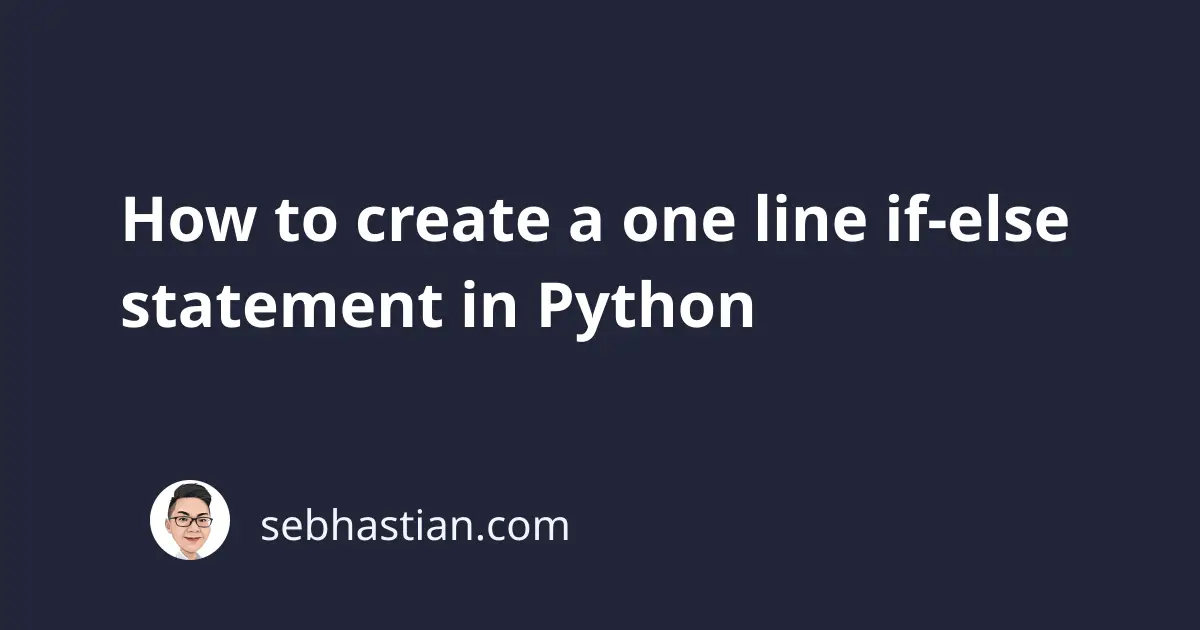
To create a one line if-else
statement in Python, you need to use the ternary operator syntax as follows:
a if condition else b
The ternary operator in Python is used to return an expression a
or b
based on the condition
that’s defined in your code.
The following tutorial shows you examples of creating one line if
statements in practice.
Writing a one line if-else statement
Let’s see an example that’s easy to understand. Suppose you create a program for a bank, and you want to determine if a person is old enough to apply for a bank account.
In most countries, a person needs to be 18 years or older, so you can use an if-else
statement as follows:
age = 19
if age >= 18:
print("Welcome!")
else:
print("Sorry. Not allowed!")
The above code prints a different message based on the value of the age
variable.
Next, let’s rewrite the same code using the ternary operator:
age = 19
message = "Welcome!" if age >= 18 else "Sorry. Not allowed!"
print(message)
In the above example, the if
statement checks if the value of age
is greater than or equal to 18
. If it is, the expression evaluates to the string “Welcome!”. If it’s not, the expression evaluates to the string “Sorry. Not allowed!”.
While a regular if
statement puts the condition first and then the expression after, a ternary condition requires you to put the expression first before the if
keyword.
The ternary operator also supports an elif
condition, but the syntax is hard to read as follows:
a if condition_1 else b if condition_2 else c
Again, let’s see an example with a regular if-elif-else
statement first.
Suppose you have a program that determines whether the day is Saturday, Sunday, or a weekday and assigns a different activity to each day:
day = "Monday"
if day == "Sunday":
activity = "Go to church"
elif day == "Saturday":
activity = "Go to mall"
else:
activity = "Go to office"
print(activity)
You can rewrite the code above using ternary operator as follows:
day = "Sunday"
activity = "Go to church" if day == "Sunday" else "Go to mall" if day == "Saturday" else "Go to office"
print(activity)
I’m sure you’re perplexed by the ternary conditions above. While the ternary operator can handle multiple conditions, it’s not recommended because it sacrifices readability.
You can separate the ternary conditions by wrapping them in parentheses as follows:
day = "Sunday"
activity = (
"Go to church" if day == "Sunday"
else "Go to mall" if day == "Saturday"
else "Go to office"
)
print(activity)
But I believe the regular if-elif-else
statement is far easier to read than the ternary statement shown above.
You should only use ternary operators for simple statements that have one condition, ideally with no mathematical operations.
Suppose you have conditions that involve numbers like this:
x = 7
if x > 10:
y = x / 2
else:
y = x * 2
If you convert the conditional above using ternary, here’s the result:
x = 7
y = x / 2 if x > 10 else x * 2
Oops.. that’s just nauseating to read. As the saying goes, “With great power comes great responsibility.”
Even though you can create a one liner if-else
statement in Python, you need to exercise caution when using it.
If you write a one line if-else
statement that has many conditionals with complex rules, your code will be hard to read and understand.
Conclusion
Python allows you to write a one line if-else
statement by using the ternary operator syntax. But you need to be careful as writing complex conditionals using the ternary operator makes your code confusing.
I hope this tutorial is helpful. See you in other tutorials! 👋