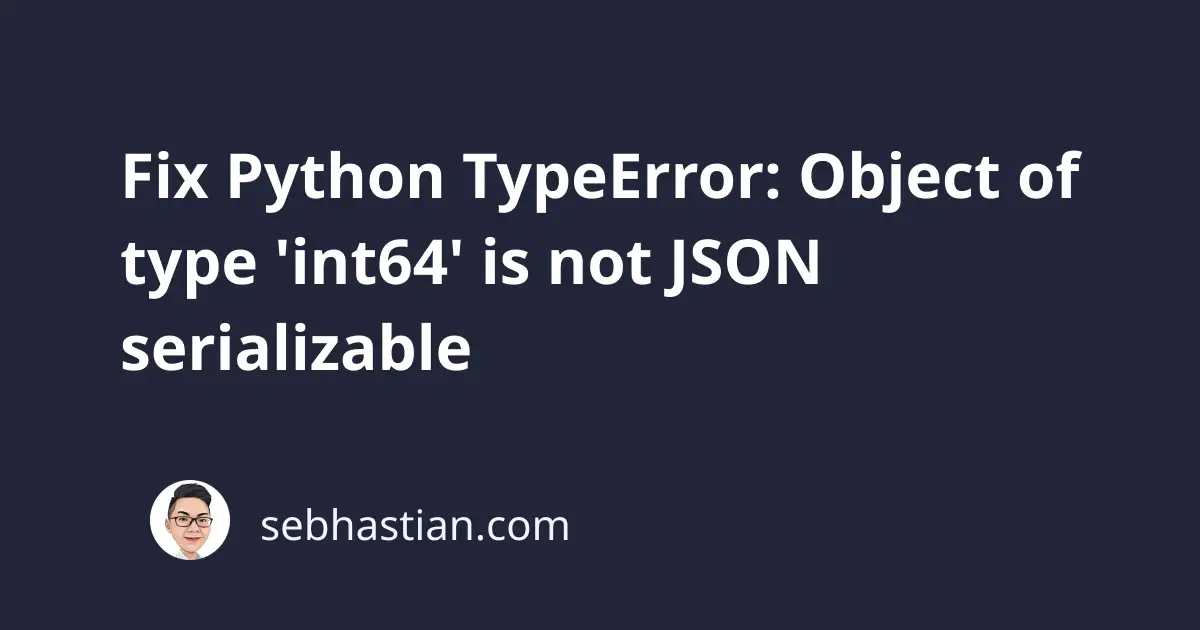
The Python TypeError: Object of type 'int64' is not JSON serializable
error message occurs when you try to convert an int64
object to a JSON string.
To fix this error, you need to convert the int64
type to a JSON-serializable type(such as int
).
This error commonly happens when you use the NumPy module in your Python code.
Suppose you call the json.dumps()
function on a NumPy int64
object as shown below:
import numpy as np
import json
score = np.int64(90)
json_str = json.dumps(score) # ❌
The JSON dump()
and dumps()
methods can only convert Python native data types, such as:
- dict
- list
- str
- int
- float
- bool
- None
Since int64
is a data type created by NumPy, the json
module doesn’t know how to handle it.
This is why Python returns the following error:
Traceback (most recent call last):
File ...
TypeError: Object of type int64 is not JSON serializable
To solve this error, you can convert the int64
object into a native Python int
object using the int()
construct.
See the example below:
import numpy as np
import json
score = np.int64(90)
int_value = int(score)
json_str = json.dumps(int_value) # ✅
print(json_str) # 90
If you have lots of Numpy int64
variables in your code, then converting each of the variables into int
before calling json.dumps()
can be tedious.
Instead of manually converting the int64
values, you can extend the JSONEncoder
class and instruct the class on how to convert NumPy custom objects into serializable objects.
Here’s how you create a custom JSON encoder in Python:
import json
import numpy as np
# Extend the JSONEncoder class
class NpEncoder(json.JSONEncoder):
def default(self, obj):
if isinstance(obj, np.integer):
return int(obj)
if isinstance(obj, np.floating):
return float(obj)
if isinstance(obj, np.ndarray):
return obj.tolist()
return json.JSONEncoder.default(self, obj)
The NpEncoder
class above extends the JSONEncoder
class from the json
module.
The default()
method is called whenever the encoder encounters an object that it does not know how to serialize. Otherwise, the encoder will run without calling this method.
Because you want to handle NumPy objects, you need to check whether the object passed is an instance of np.integer
, np.floating
, or np.ndarray
object.
The object is then converted into an int
, float
, or list
so that it can be processed by the encoder.
To use this extended encoder, you need to pass it as the cls
argument into json.dumps()
method.
See the highlighted line below:
score = np.int64(90)
json_str = json.dumps(score, cls=NpEncoder) # ✅
print(json_str)
As you can see, using the NpEncoder
class enables the json.dumps()
method to convert an int64
object.
The extended if
check also allows you to convert a NumPy ndarray
type, avoiding the ndarray is not JSON serializable error.
Conclusion
To summarize, you encounter the Python TypeError: Object of type 'int64' is not JSON serializable
error message when you try to convert an int64
object into a JSON string.
To fix this error, you can convert the int64
data type to an int
, or you can create a custom class that extends the JSON.encoder
class.
If you’re only dealing with a small amount of NumPy data, then converting the type directly is enough.
Otherwise, extending the JSON encoder class is a great solution as you can avoid repeating yourself.