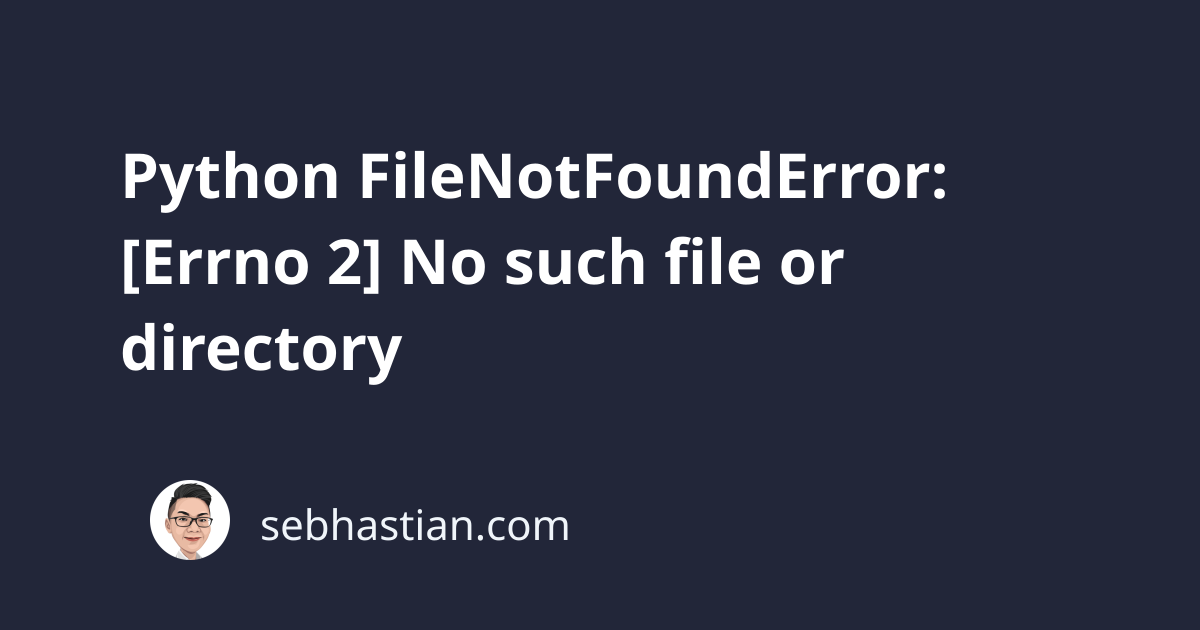
The error FileNotFoundError: [Errno 2] No such file or directory
means that Python can’t find the file or directory you are trying to access.
This error can come from calling the open()
function or the os
module functions that deal with files and directories.
To fix this error, you need to specify the correct path to an existing file.
Let’s see real-world examples that trigger this error and how to fix them.
Error from open() function
Suppose you use Python to open and read a file named output.txt
as follows:
with open('output.txt', 'r') as f:
data = f.read()
Python will look for the output.txt
file in the current directory where the code is executed.
If not found, then Python responds with the following error:
Traceback (most recent call last):
File ...
with open('output.txt', 'r') as f:
FileNotFoundError: [Errno 2] No such file or directory: 'output.txt'
If the file exists but in a different directory, then you need to specify the correct path to that file.
Maybe the output.txt
file exists in a subdirectory like this:
.
├── logs
│ └── output.txt
└── script.py
To access the file, you need to specify the directory as follows:
with open('logs/output.txt', 'r') as f:
data = f.read()
This way, Python will look for the output.txt
file inside the logs
folder.
Sometimes, you might also have a file located in a sibling directory of the script location as follows:
.
├── code
│ └── script.py
└── logs
└── output.txt
In this case, the script.py
file is located inside the code
folder, and the output.txt
file is located inside the logs
folder.
To access output.txt
, you need to go up one level from as follows:
with open('../logs/output.txt', 'r') as f:
data = f.read()
The path ../
tells Python to go up one level from the working directory (where the script is executed)
This way, Python will be able to access the logs
directory, which is a sibling of the code
directory.
Alternatively, you can also specify the absolute path to the file instead of a relative path.
The absolute path shows the full path to your file from the root directory of your system. It should look something like this:
# Windows absolute path
C:\Users\sebhastian\documents\logs
# Mac or Linux
/home/user/sebhastian/documents/logs
Pass the absolute path and the file name as an argument to your open()
function:
with open('C:\Users\sebhastian\documents\logs\output.txt', 'r') as f:
data = f.read()
You can add a try/except
statement to let Python create the file when it doesn’t exist.
try:
with open('output.txt', 'r') as f:
data = f.read()
except FileNotFoundError:
print("The file output.txt is not found")
print("Creating a new file")
open('output.txt', 'w') as f:
f.write("Hello World!")
The code above will try to open and read the output.txt
file.
When the file is not found, it will create a new file with the same name and write some strings into it.
Also, make sure that the filename or the path is not misspelled or mistyped, as this is a common cause of this error as well.
Error from os.listdir() function
You can also have this error when using a function from the os
module that deals with files and directories.
For example, the os.listdir()
is used to get a list of all the files and directories in a given directory.
It takes one argument, which is the path of the directory you want to scan:
import os
files = os.listdir("assets")
print(files)
The above code tries to access the assets
directory and retrieve the names of all files and directories in that directory.
If the assets
directory doesn’t exist, Python responds with an error:
Traceback (most recent call last):
File ...
files = os.listdir("assets")
FileNotFoundError: [Errno 2] No such file or directory: 'assets'
The solution for this error is the same. You need to specify the correct path to an existing directory.
If the directory exists on your system but empty, then Python won’t raise this error. It will return an empty list instead.
Also, make sure that your Python interpreter has the necessary permission to open the file. Otherwise, you will see a PermissionError message.
Conclusion
Python shows the FileNotFoundError: [Errno 2] No such file or directory
message when it can’t find the file or directory you specified.
To solve this error, make sure you the file exists and the path is correct.
Alternatively, you can also use the try/except
block to let Python handle the error without stopping the code execution.