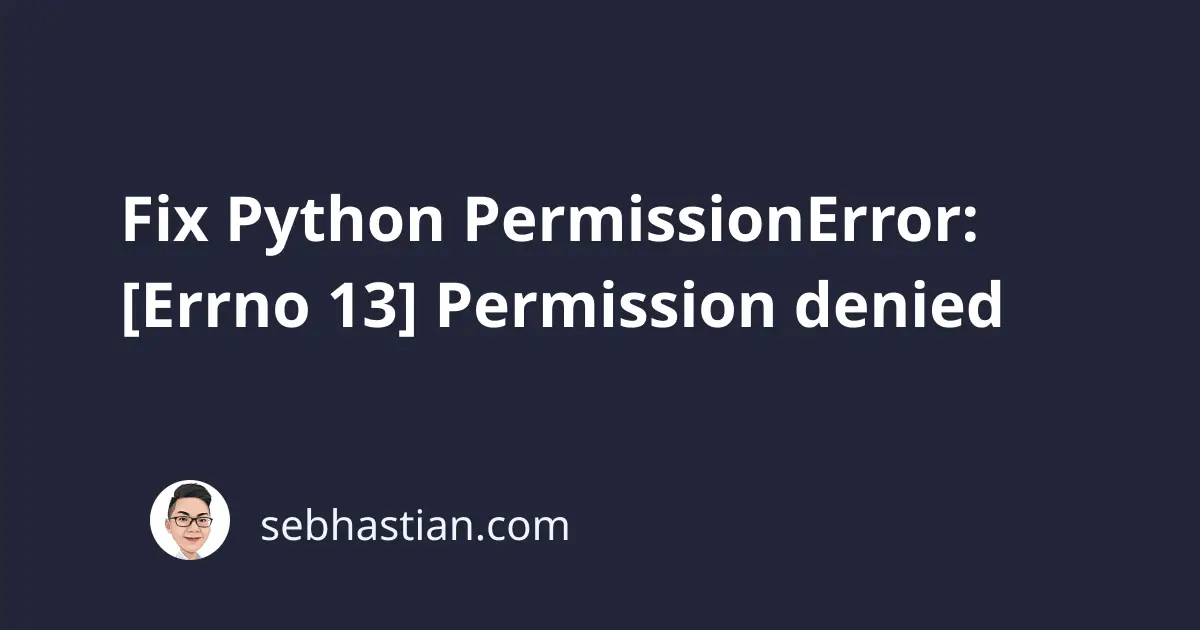
Python responds with PermissionError: [Errno 13] Permission denied message when you try to open a file with the following exceptions:
This article will help you to resolve the issues above and fix the PermissionError message.
You specify a path to a directory instead of a file
When you call the open()
function, Python will try to open a file so that you can edit that file.
The following code shows how to open the output.txt
file using Python:
with open('text_files/output.txt', 'w') as file_obj:
file_obj.write('Python is awesome')
While opening a file works fine, the open()
function can’t open a directory.
When you forget to specify the file name as the first argument of the open()
function, Python responds with a PermissionError.
The code below:
with open('text_files', 'w') as file_obj:
Gives the following output:
Because text_files
is a name of a folder, the open()
function can’t process it. You need to specify a path to one file that you want to open.
You can write a relative or absolute path as the open()
function argument.
An absolute path is a complete path to the file location starting from the root directory and ending at the file name.
Here’s an example of an absolute path for my output.txt
file below:
abs_path = "/Users/nsebhastian/Desktop/DEV/python/text_files/output.txt"
When specifying a Windows path, you need to add the r
prefix to your string to create a raw string.
This is because the Windows path system uses the backslash symbol \
to separate directories, and Python treats a backslash as an escape character:
# Define a Windows OS path
abs_path = r"\Desktop\DEV\python\text_files\output.txt"
Once you fixed the path to the file, the PermissionError message should be resolved.
The file is already opened elsewhere (in MS Word or Excel, .etc)
Microsoft Office programs like Word and Excel usually locked a file as long as it was opened by the program.
When the file you want to open in Python is opened by these programs, you will get the permission error as well.
See the screenshot below for an example:
To resolve this error, you need to close the file you opened using Word or Excel.
Python should be able to open the file when it’s not locked by Microsoft Office programs.
You don’t have the required permissions to open the file
Finally, you will see the permission denied error when you are trying to open a file created by root or administrator-level users.
For example, suppose you create a file named get.txt
using the sudo
command:
sudo touch get.txt
The get.txt
file will be created using the root user, and a non-root user won’t be able to open or edit that file.
To resolve this issue, you need to run the Python script using the root-level user privilege as well.
On Mac or Linux systems, you can use the sudo
command. For example:
sudo python script.py
On Windows, you need to run the command prompt or terminal as administrator.
Open the Start menu and search for “command”, then select the Run as administrator menu as shown below:
Run the Python script using the command prompt, and you should be able to open and write to the file.
Conclusion
To conclude, the error “PermissionError: [Errno 13] Permission denied” in Python can be caused by several issues, such as: opening a directory instead of a file, opening a file that is already open in another program, or opening a file for which you do not have the required permissions.
To fix this error, you need to check the following steps:
- Make sure that you are specifying the path to a file instead of a directory
- Close the file if it is open in another program
- Run your Python script with the necessary permissions.
By following these steps, you can fix the “PermissionError: [Errno 13] Permission denied” error and successfully open and edit a file in Python.