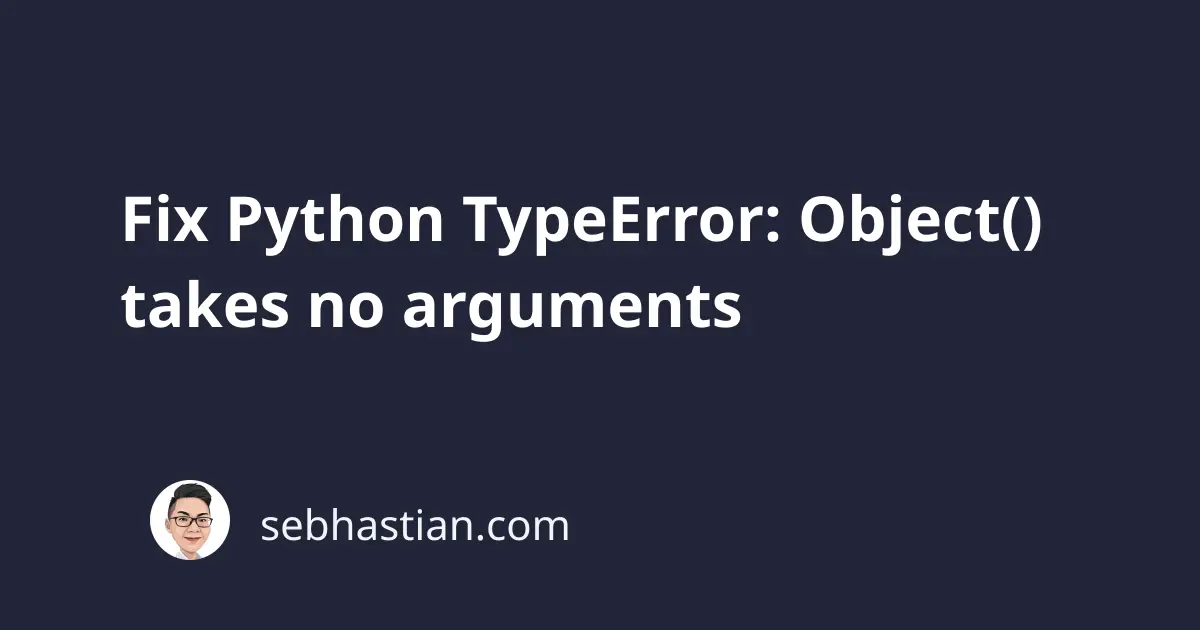
TypeError: Object() takes no arguments
error occurs when you pass one or more arguments to instantiate an object from a class that has no __init__()
method.
When initializing a Python object from a class, you can pass a number of arguments into the object by defining the __init__()
method.
The __init__()
method is the constructor method that will be run when you create a new object.
This article will show you an example that causes this error and how to fix it.
How to reproduce this error
Usually, you get this error when you try to instantiate an object from a class without an __init__()
method.
Suppose you have a class as follows:
class Human:
def walk():
print("Walking")
person = Human("Nathan") # ❌
The Human
class in the example only has walk()
method, but I instantiated a new person
object and passed one string argument to it.
As a result, Python responds with this error message:
Traceback (most recent call last):
File ...
person = Human("Nathan")
TypeError: Human() takes no arguments
You might see the error message worded a bit differently in other versions of Python, such as:
TypeError: this constructor takes no arguments
TypeError: object() takes no parameters
TypeError: class() takes no arguments
But the point of these errors is the same: You’re passing arguments to instantiate an object from a class that has no __init__()
method.
Alright, now that you understand why this error occurs, let’s see how you can fix it.
How to Fix TypeError: Object() takes no arguments
To resolve this error, you need to make sure that the __init__()
method is defined in your class.
The __init__()
method must be defined correctly as shown below:
class Human:
def __init__(self, name):
self.name = name
def walk():
print("Walking")
person = Human("Nathan") # ✅
print(person.name) # Nathan
Please note the indentation and the typings of the __init__()
method carefully.
If you mistyped the method as _init_
with a single underscore, Python doesn’t consider it to be a constructor method, so you’ll get the same error.
The following shows a few common typos when defining the __init__()
method:
init:
_init_:
init():
_init_():
__init():
__int__
The constructor method in a Python class must be exactly named as __init__()
with two underscores. Any slight mistyping will cause the error.
You also need to define the first argument as self
, or you will get the error message init
takes 1 positional argument but 2 were given
The last thing you need to check is the indentation of the __init__()
method.
Make sure that the method is indented by one tab, followed by the function body in two tabs.
Wrong indentation will make Python unable to find this method. The following example has no indent when defining the method:
class Human:
def __init__(self, name): # ❌
self.name = name
Once you have the __init__()
method defined in your class, this error should be resolved.
Conclusion
Python shows TypeError: Object() takes no arguments
when you instantiate an object from a class that doesn’t have an __init__()
method.
To fix this error, you need to check your class definition and make sure that the __init__()
method is typed and indented correctly.
Great work solving this error! I’ll see you again in other articles. 👍