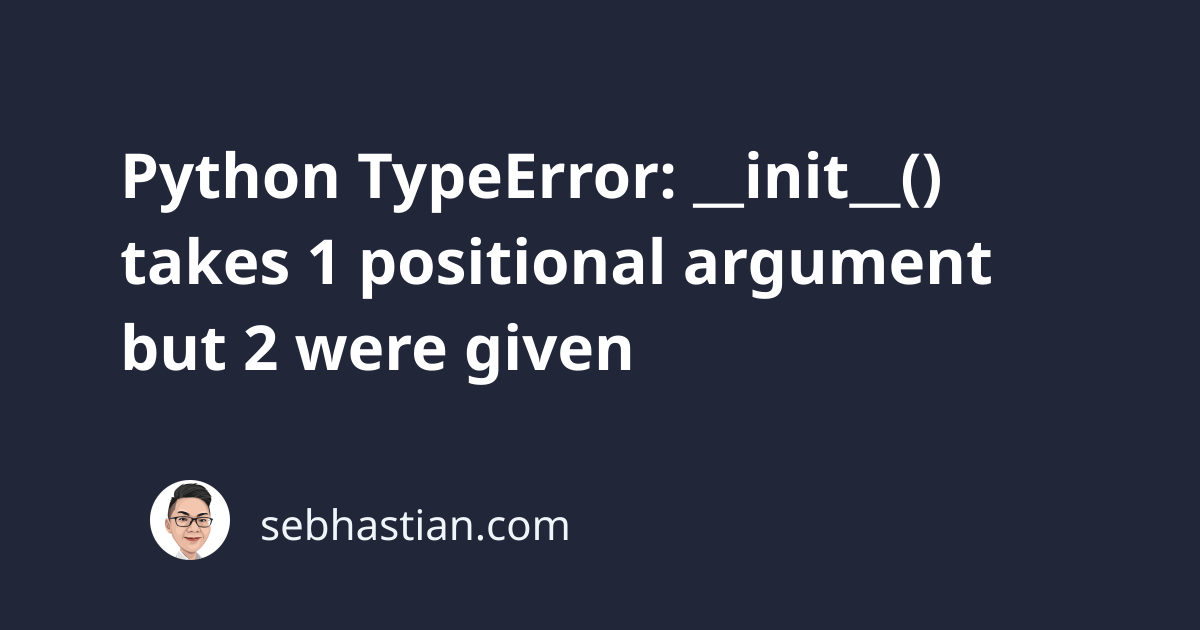
Python shows TypeError: __init__() takes 1 positional argument but 2 were given
when you instantiate an object of a class and pass more arguments to the constructor (__init__()
method) than it is expecting.
In Python, the instance of the class (self
) is automatically passed as the first argument when calling an instance method.
The exception to this rule are:
- class methods, which get the class instead of the instance as the first argument
- static methods, which don’t get a first argument at all
When you define and instantiate a class as follows:
class Car:
def __init__(price):
self.price = price
# create an instance, pass price
my_car = Car(8000)
Python will pass self, 8000
to the __init__
method under the hood.
But because you define only price
as the parameter of the method, Python responds with an error:
Traceback (most recent call last):
File ...
my_car = Car(8000)
TypeError: Car.__init__() takes 1 positional argument but 2 were given
To fix this error, you need to add self
parameter to the __init__
method:
class Car:
def __init__(self, price):
self.price = price
my_car = Car(8000) # ✅
print(my_car.price) # 8000
If you don’t need the self
instance, you can declare a static method as shown below:
class Car():
@staticmethod
def start(message):
print(message)
my_car = Car()
my_car.start("Start engine!")
# or
Car.start("Vroom vroom!")
A static method can be called directly from the class without having to create an instance.
Because static methods don’t receive a first argument, the code above runs without any issue.
Finally, this error can happen to any function you declare in your project. Suppose you have an add()
function defined as follows:
def add(x, y):
return x + y
add(2, 4, 5) # ❌
The add()
function is defined with two arguments, but 3 arguments were passed when calling that function.
As a result, Python raises another error:
Traceback (most recent call last):
File ...
add(2, 4, 5)
TypeError: add() takes 2 positional arguments but 3 were given
Depending on what you want to achieve, you can either add another argument to the function definition, or you can remove the third argument from the function call.
To summarize, the message takes 1 positional argument but 2 were given
means a method or function expects only 1 argument, but you are passing two.
If this error appears when calling the __init__
method, it’s likely that you forget to define the self
argument, which is passed to the method implicitly.
To solve the error, add the self
argument to the __init__
method as shown in this article.