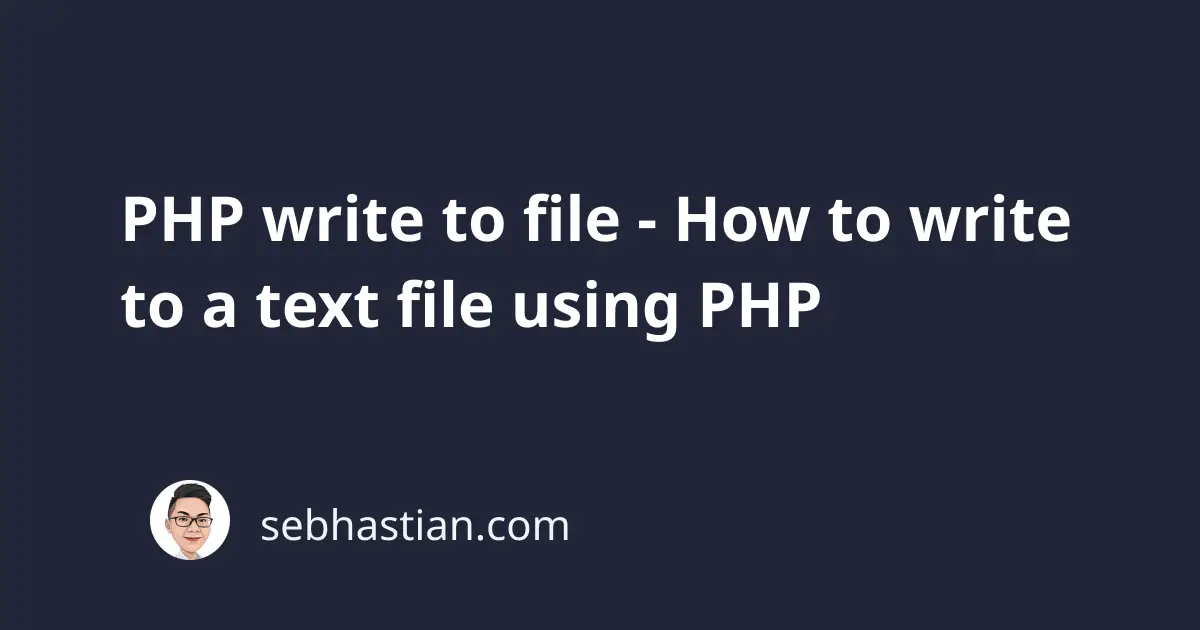
There are two ways you can write text data to a file in PHP:
- Use the
file_put_contents()
function - Use the
fopen()
,fwrite()
, andfclose()
functions
This tutorial will help you learn how to use both methods to write data to a file in PHP.
Let’s start with using the file_put_contents()
function
PHP write to file with file_put_contents()
The file_put_contents()
function is a built-in PHP function used for writing data to a file.
The syntax of the function is as follows:
file_put_contents(
string $filename,
mixed $data,
int $flags = 0,
?resource $context = null
): int|false
The $filename
and $data
parameters are required while $flags
and $context
are optional.
The function returns the int
number of bytes written to the file. When the write operation fails, it returns false
or falsy numbers.
The code example below shows how to write a text to the example.txt
file:
file_put_contents("example.txt", "Hello World!");
The code above will produce the following result:
The file_put_contents()
function will look for the file you passed as the $filename
parameter.
When the file isn’t found, PHP will create the file. It will then write the data you passed as its $data
parameter.
Once the write operation is finished, the function will automatically close the file from access.
The $flags
parameter can be used to modify the behavior of the function. Here are the possible values:
FILE_USE_INCLUDE_PATH
- Search for the file in the include path, which is set from the PHP configurationFILE_APPEND
- Append data to an existing file instead of overwriting itLOCK_EX
- Lock the file while you access it so others can’t edit
The flags can be joined with the binary OR operator (|
) as follows:
file_put_contents(
"example.txt",
" Good Morning!",
FILE_APPEND | LOCK_EX
);
By default, file_put_contents()
will overwrite the file data on write.
The FILE_APPEND
flag will cause the function to append the data instead of overwriting it.
The $context
flag is used to add a stream context for the operation. It’s can be useful when you’re writing to a remote file using an FTP connection.
And that’s how you use the file_put_contents()
function to write data to a file in PHP.
Using fopen(), fwrite(), fclose() functions
The PHP fopen()
, fwrite()
, and fclose()
functions are used to write data to a file.
Under the hood, these functions are similar to calling the file_put_contents()
function, only in three separate steps:
Step #1 - Open the file using fopen()
Call the fopen()
function and pass two arguments into it:
- The
$filename
for the file name - The
$mode
to specify the access mode to the file. Passw
for write anda
for append
The code example below will cause PHP to look for the test.txt
file:
$file = fopen("text.txt", "w");
The function returns a resource stream that you can use to write data into.
Step #2 - Write to the file using fwrite() and close it with fclose()
The fwrite()
function is used to write to a file in PHP.
You need to pass two arguments to the function:
- The
$stream
resource created usingfopen()
- The
$data
string to write to the stream
Here’s an example of writing to the stream:
// 👇 open a stream
$file = fopen("text.txt", "w");
// 👇 write to the stream
fwrite($file, "Somewhere over the rainbow");
fwrite($file, "\n Skies are blue");
// 👇 close the stream
fclose($file);
When your text has multiple lines, you can add the \n
symbol to create a line break in your text.
Although PHP will close the file before exiting, you should close it manually using the fclose()
function.
Closing a file using fclose()
is considered good practice to avoid corrupting a file.
You can also crash the server when you open many files without closing them.
Conclusion
Now you’ve learned how to write data to a file in PHP.
You can use either file_put_contents()
or the combination of fopen()
, fwrite()
, and fclose()
depending on your requirements.
Thanks for reading! May this tutorial be useful for you 🙏