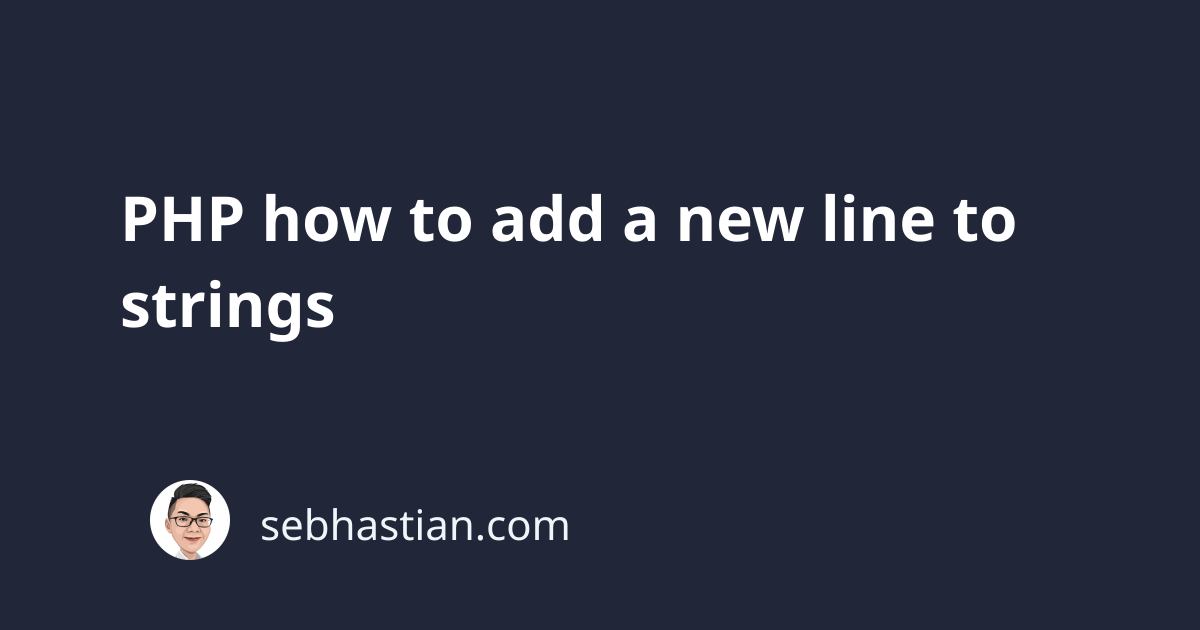
To create a new line in PHP strings, you need to add the new line character \n
or \r\n
in your source code.
The \n
is the new line character for UNIX operating systems, while \r\n
is for Windows operating systems.
The code example below shows how you use the new line characters:
<?php
echo "Hello! \nHow are you? \r\n I'm fine, thanks!";
The output in the console will be as follows:
Hello!
How are you?
I'm fine, thanks!
Notice how there’s a whitespace character before the I'm
word on the third line. This is because there’s a space right after the \r\n
characters in the source code.
You need to mind the whitespace after the new line characters because it will be interpreted literally by PHP.
The \r\n
characters work for both UNIX and Windows systems, so you can use \r\n
characters for all your line breaks to keep your code consistent.
PHP new line on the browser
Please note that the new line characters won’t work when you run the PHP script from the browser.
To make it work, you need to add the nl2br()
function to the echo
statement:
echo nl2br("Hello! \nHow are you? \r\n I'm fine, thanks!", false);
The output string from the code above will be as follows:
Hello! <br>
How are you? <br>
I'm fine, thanks!
The nl2br()
function has 2 parameters:
$input
is thestring
you want to operate on$use_xhtml
is aboolean
value determining whether to use XHTML compatible line break or not.
By default, nl2br()
will use the <br />
tag. You can tell the function to use <br>
instead by passing false
as the second argument of the function.
// 👇 nl2br syntax
nl2br(string $string, boolean $use_xhtml = true): string
While you can add the <br>
tag to the string yourself, using nl2br()
allows your string to be compatible with both the browser and the console.
And that’s how you create new lines in PHP. Nice! 👍