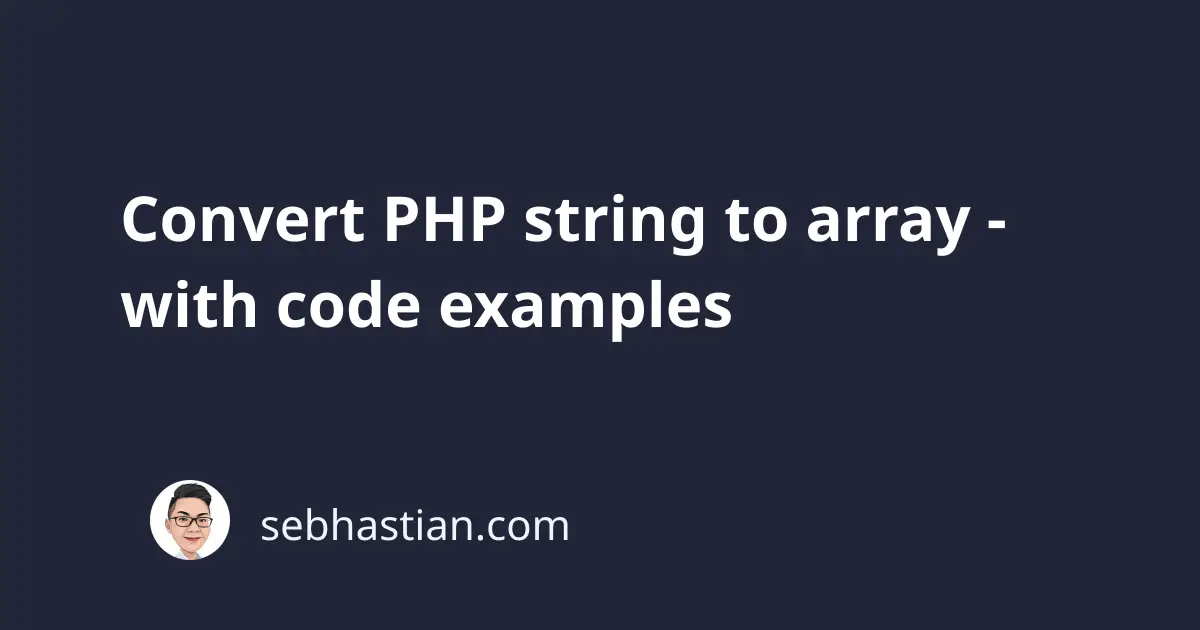
Converting a PHP string to an array can be a useful way to manipulate and process data stored in a string.
PHP provides six functions that make it easy to convert a string to an array:
In this article, you will see how these functions can be used to convert a string to an array in PHP.
explode() function
One of the most common methods of converting a PHP string to an array is to use the explode()
function.
This function takes a string and a delimiter (separator) as its arguments.
It then returns an array containing the substrings of the original string that are delimited by the specified delimiter.
For example, the following code converts a string that contains a list of names separated by commas to an array containing each individual name as an element:
$names = "John, Jane, Bob, Alice";
$name_array = explode(", ", $names);
print_r($name_array);
The print_r
result will be:
Array
(
[0] => John
[1] => Jane
[2] => Bob
[3] => Alice
)
The explode()
function is easy to use as you only need to pass the string and the delimiter where the string will be split into the function.
Note that in the delimiter argument, the white space after the comma is also added. This is done so that the returned array elements do not contain any white space.
str_split() function
Another function that can be used to convert a PHP string to an array is the str_split()
function.
str_split(string $string, int $length = 1): array
This function takes a string and an optional length
argument. The return value is an array containing the individual characters of the string as elements.
Consider the example below:
$word = "hello";
$word_array = str_split($word);
print_r($word_array);
Output:
Array
(
[0] => h
[1] => e
[2] => l
[3] => l
[4] => o
)
In the example above, the length
argument is not passed to the str_split()
function, so it returned an array of individual characters.
If the optional length
argument is provided, each value of the array will contain the specified number of characters.
The last value may be shorter if the given string can’t be divided evenly:
$word = "hello";
$word_array = str_split($word, 2);
print_r($word_array);
Output:
Array
(
[0] => he
[1] => ll
[2] => o
)
Depending on the result you want to achieve, you can adjust the length
argument as required.
preg_split() function
The preg_split()
function takes a regular expression and a string as its arguments.
It returns an array containing the substrings of the original string that match the specified regular expression.
This function is particularly useful when the delimiter used to separate the elements of the string is a complex pattern, such as a series of multiple characters or a regular expression.
Suppose you want to split a string by three different separators: a comma, a white space, and a semicolon.
You can use the regex /[,\s:]/
as shown below:
$date = "10th December,2022:birthday";
$date_array = preg_split("/[,\s:]/", $date);
print_r($date_array);
The output will be:
Array
(
[0] => 10th
[1] => December
[2] => 2022
[3] => birthday
)
preg_match_all() function
The preg_match_all()
function takes a regular expression and a string as its arguments.
The function then returns a multidimensional array containing all of the matches of the regular expression in the given string.
This function is similar to preg_split()
, but instead of splitting the string and returning a single array, it returns a multidimensional array of matching substrings.
For example, suppose you have a string that contains a list of dates.
To extract all of the dates from the string, we can use the preg_match_all()
function as follows:
$dates = "Birthdays: 3rd January 2022, 15th February 2022";
$pattern = "/\d{1,2}(?:st|nd|rd|th)\s[A-Za-z]+\s\d{4}/";
preg_match_all($pattern, $dates, $matches);
print_r($matches);
Output:
Array
(
[0] => Array
(
[0] => 3rd January 2022
[1] => 15th February 2022
)
)
As you can see, only the string that matches the regex pattern will be extracted by the preg_match_all()
function.
str_getcsv() function
The str_get_csv()
function is used to parse a CSV (Comma-Separated Values) string into an array.
This function is similar to the explode()
function, but it allows for more control over the format of the string and the resulting array.
For example, suppose you have a CSV string that contains a list of names that you want to convert to an array:
$csv = "John,Jane,Bob,Alice";
$name_array = str_getcsv($csv);
print_r($name_array);
Output:
Array
(
[0] => John
[1] => Jane
[2] => Bob
[3] => Alice
)
Since you didn’t provide any arguments to the str_getcsv()
function, it used the default delimiter (a comma) and the default enclosure (double quote) to parse the CSV string.
When your CSV uses a different delimiter, you can specify the delimiter as the second argument.
The example below shows how to split the same string but with a pipe (|
) delimiter:
$csv = "John|Jane|Bob|Alice";
$columns = str_getcsv($string, "|");
Finally, here’s a code example that you can use to load a CSV file and split its content into an array:
$csv = file_get_contents("data.csv");
// split the string into an array of lines
$lines = explode("\n", $csv);
// loop through each line
foreach ($lines as $line) {
// split the string
$fields = str_getcsv($line);
// print the fields array
print_r($fields);
}
The code above will take the data.csv
file you have and process it so that you can use the values in your PHP code.
json_decode() function
The json_decode()
function is used to convert a JSON string to a PHP object or array.
To convert a JSON string to an array in PHP, you can use the following code:
$json = '{"name":"Nathan","age":28,"email":"[email protected]"}';
$array = json_decode($json, true);
print_r($array);
The output will be:
Array
(
[name] => Nathan
[age] => 28
[email] => [email protected]
)
In the example above, a JSON string is defined that contains an object with three properties: name
, age
, and email
.
When calling the json_decode()
function, you need to pass the JSON string and set the second argument to true
.
The second argument is also known as the associative
parameter. When set to true
, the function will return an associative array. By default, the function will return an object.
The resulting array contains the properties of the original JSON object in key-value format.
This allows you to easily access and manipulate the data contained in the JSON string.
In addition to the associative
parameter, the json_decode()
function also accepts several other optional arguments that can be used to customize the behavior of the function.
For example, the depth
parameter can be used to specify the maximum depth of the resulting array, and the options
parameter can be used to specify additional options for parsing the JSON string.
For more information about the json_decode()
function and its arguments, you can refer to the PHP documentation at php.net.
Conclusion
In summary, there are many different ways to convert a PHP string to an array. The appropriate solution to use will depend on the format and contents of the string, as well as the desired outcome of the conversion.
Whether you are working with simple delimiter-separated strings, complex regular expressions, or JSON and other serialized data, there is a PHP function or technique that can be used to convert the string to an array.
By using the built-in functions and techniques discussed in this article, you can easily convert a PHP string to an array and manipulate the resulting data as needed.
Also, you might want to learn how to convert an array to a string using PHP.