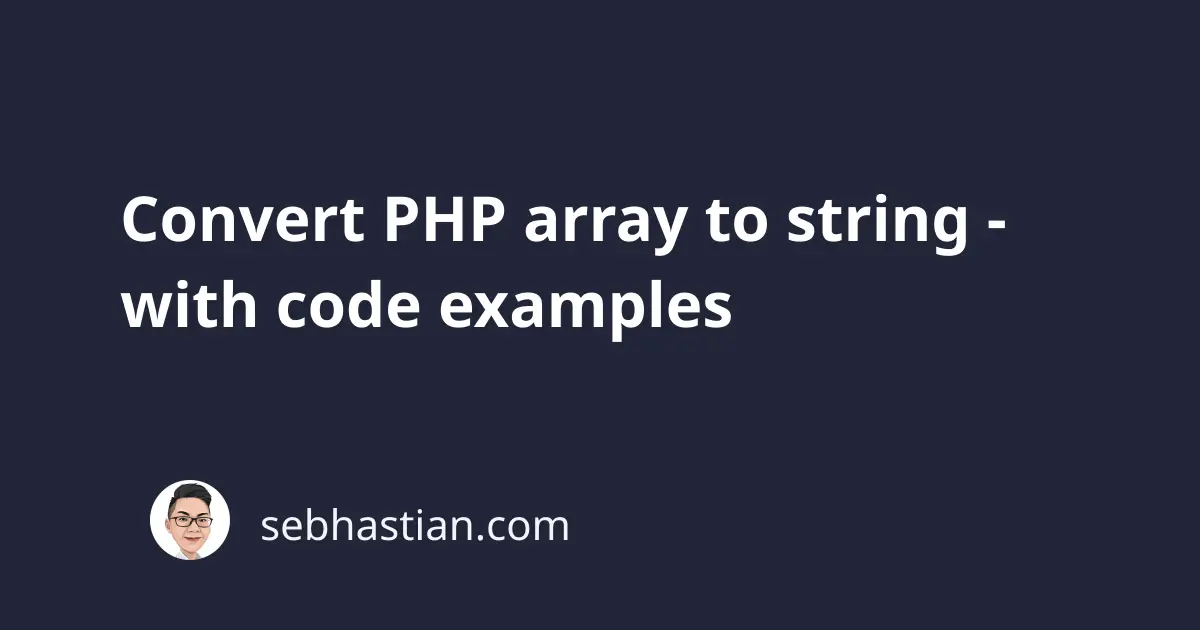
PHP provides a number of functions that you can use to convert arrays to strings.
Using the implode()
function
The implode()
function is a built-in function in PHP that takes an array and converts it into a string by joining the elements together.
It takes two arguments:
- the
delimiter
, which is the character used to separate the elements of the array. - the
array
you want to process.
Here is an example of how to use the implode()
function to convert an array to a string:
$array = array("apple", "banana", "cherry");
$string = implode(",", $array);
echo $string;
// output: "apple,banana,cherry"
You can add a space on the delimiter
so that the words will be separated in the string:
$string = implode(", ", $array);
Using the join() function
The join()
function is another built-in function in PHP that can be used to convert an array to a string.
This function is an alias of the implode()
function, so it accepts the same parameters and does the same thing.
Here is an example of how to use the join()
function to convert an array to a string:
$array = array("apple", "banana", "cherry");
$string = join(",", $array);
echo $string;
// output: "apple,banana,cherry"
Using the json_encode() function
The json_encode()
function is a built-in function in PHP that converts an array or an object to a JSON string.
It takes one argument: the array
or object
that you want to convert to a JSON string.
Here is an example of how to use the json_encode()
function to convert an array to a string:
$array = array("apple", "banana", "cherry");
$string = json_encode($array);
echo $string;
// output: ["apple","banana","cherry"]
When using the json_encode()
function, you will still have the square brackets in the string.
Using a foreach loop
You can also use a loop to manually iterate through the elements of an array and concatenate them into a string.
Here is an example of how to use the foreach
loop to convert an array to a string:
$array = array("apple", "banana", "cherry");
$string = "";
foreach ($array as $element) {
$string .= $element . "-";
}
echo $string;
// output: "apple-banana-cherry-"
Remember to remove the trailing dash from the end of the string if you don’t want it to be included in the final output.
To conclude, there are several ways in which you can convert an array to a string in PHP.
The implode()
and join()
functions are the most straightforward and easiest to use, but you can also use the json_encode()
function or a foreach
loop if you need more control over the process.
In addition, you might also want to know how to convert a string to an array in PHP.