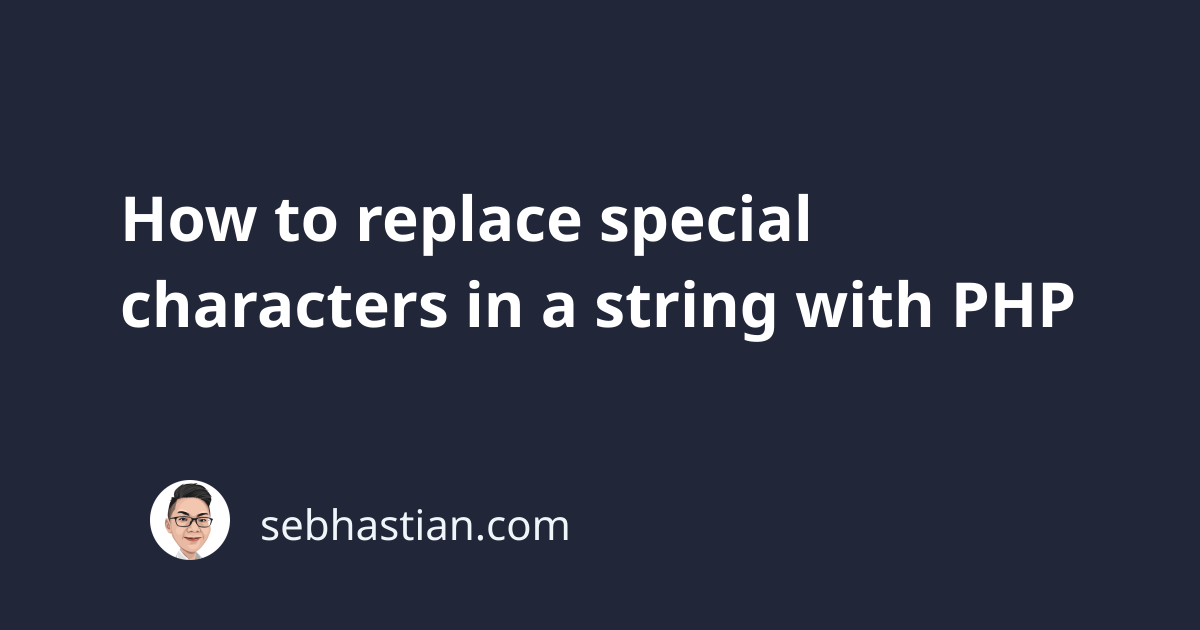
To replace special characters in a string, you can use the str_replace()
function.
The str_replace()
function is used to replace all occurrences of specific characters with a replacement you specified as its parameters.
Replace dash symbols with underscore symbols
For example, suppose you want to replace the special character dash (-
) with an underscore (_
). Here’s how you do it:
<?php
$str = "learning-php-language";
$new = str_replace("-", "_", $str);
print $new;
// Output: learning_php_language
You need to pass three parameters to the function:
- The character to search for
- The replacement character
- The string to search from
Replace asterisk symbols with and symbols
Here’s another example to replace asterisk symbols (*
) with and symbols (&
):
<?php
$str = "john*spider*wick";
$new = str_replace("*", "&", $str);
print $new;
// Output: john&spider&wick
Replace plus and minus with white spaces
You can also replace a special character with a white space.
The example below shows how to remove two special characters +
and -
with white spaces:
$str = "The+Legend-of-Hercules++";
$new = str_replace(["+", "-"], " ", $str);
print $new;
// Output: The Legend of Hercules
Instead of passing a single value, pass an array as the first argument of the str_replace()
function.
The function will then work to replace all occurrences of the values in the array with the second argument.
Finally, you can also pass an array as the second argument:
$str = "The+Legend-of-Hercules++";
$new = str_replace(["+", "-"], ["/", ">"], $str);
print $new;
// Output: The/Legend>of>Hercules//
The str_replace()
function will replace any values found following the replacement array order.
In the above example, +
will be replaced with /
and -
gets replaced with >
.
When your replacement array count is lower than the search array, then PHP will pass an empty string to replace the remaining search values.
Now you’ve learned how to replace special characters with PHP. Nice!
You can also use the str_replace()
function to remove special characters from your string.
I hope this tutorial has been useful for you. 👍