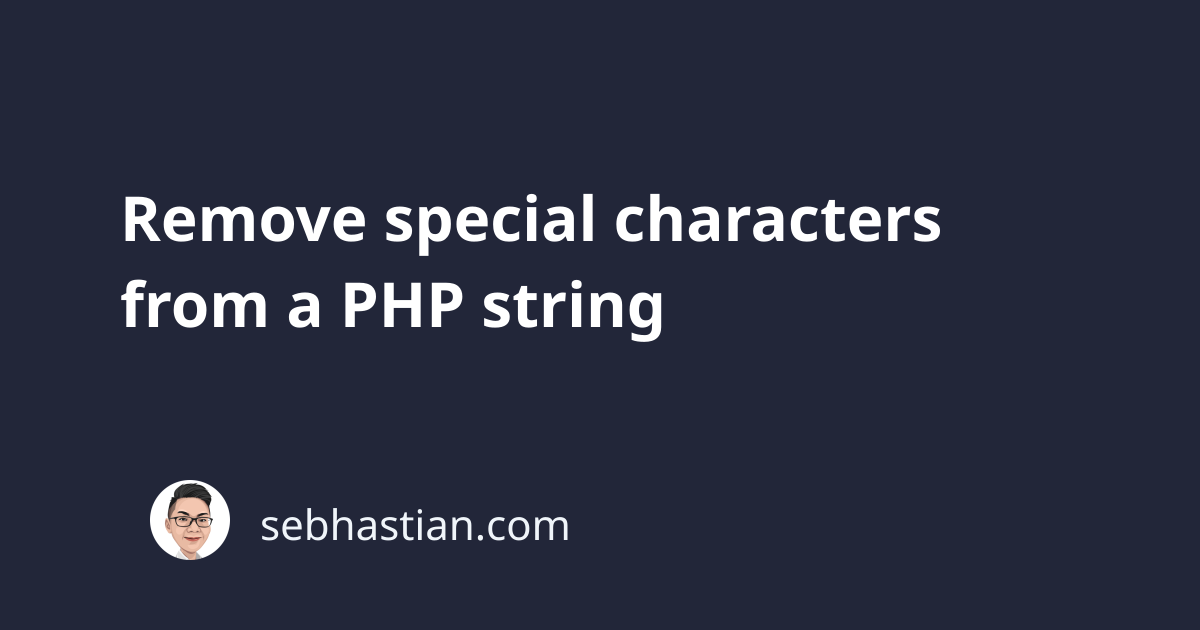
PHP has the str_replace()
and preg_replace()
functions that you can use to remove special characters from a string.
Which function to use depend on the result you want to achieve, as you will see in this tutorial.
Remove special characters using str_replace()
The str_replace()
function allows you to replace all occurrences of specific characters in your string.
You need to pass an array of special characters that you want to remove as the first argument of the function.
Then, pass the replacement for the special characters as the second argument.
Finally, pass the original string as the third argument:
<?php
$string = "<removing> ,special !!characters";
$new_string = str_replace(
[",", "<", ">", "!"], // 1. special chars to remove
"", // 2. replacement for the chars
$string // 3. the original string
);
print $new_string;
// Output: removing special characters
In the example above, the special characters ,<>!
will be replaced with an empty string.
The str_replace()
function also removes multiple occurrences of a character. You can modify the array to fit your requirements.
Remove special characters using preg_replace()
Another way to remove special characters is to use the preg_replace()
function, which involves writing a regular expression.
The regular expression pattern that you need to remove all special characters is /[^a-zA-Z0-9 ]/m
.
Here’s an example of using preg_replace()
:
$string = "<removing> ,special
char_ac-ters!@#$%^&*()-_+=~`[{]}\|;:'\",<.>/?";
$new_string = preg_replace(
'/[^a-zA-Z0-9 ]/m', // 1. regex to apply
'', // 2. replacement for regex matches
$string // 3. the original string
);
print $new_string; // removing special characters
The regex pattern above will match all characters that are not alphabet letters a-z
in upper or lower cases, the numbers 0-9
, and the space character.
Using the regex pattern, you don’t need to define the special characters one by one as in the str_replace()
function.
Conclusion
Now you’ve learned how to remove special characters using str_replace()
and preg_replace()
functions.
If you need to remove only specific special characters, it’s better to use the str_replace()
function.
When you need to remove all special characters, use the preg_replace()
function.
You are free to use the best solution for your project.