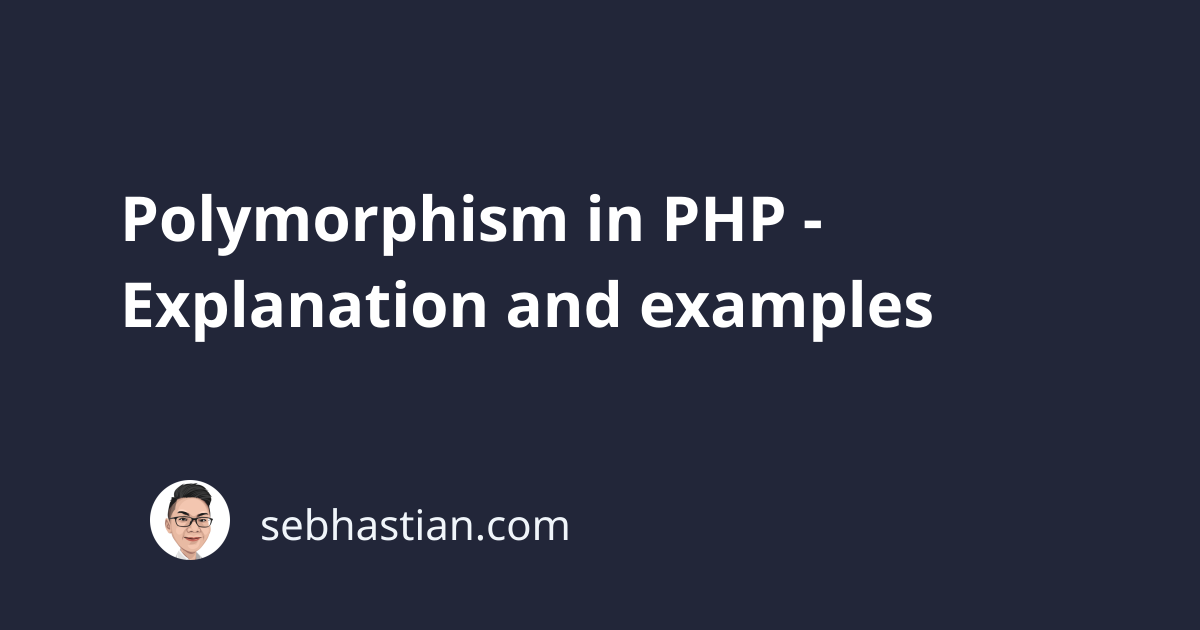
Polymorphism is a concept in object-oriented programming that allows objects of different classes to have properties and methods of a common template.
In PHP, polymorphism is achieved through inheritance and the use of interfaces. The common template used is just another class
or an interface
.
Let’s see some examples of inheritance and interfaces in action.
Example of inheritance in PHP
Inheritance enables a class to inherit the properties and methods of a parent class.
This allows child classes to reuse and extend the functionality of parent classes, allowing for more efficient and extensible code.
In PHP, a child class can inherit from a parent class using the extends
keyword.
Suppose you have a Vehicle
class defined as follows:
class Vehicle {
public $speed = 25;
function move() {
echo "The vehicle is moving at a speed of " .
$this->speed . "mph.\n";
}
}
Next, create a Car
class that inherits the Vehicle
class like this:
class Car extends Vehicle {}
When you create an instance of the Car
class, you can call the methods of the Vehicle
class as follows:
$car = new Car();
$car->move();
// Output: The vehicle is moving at a speed of 25mph.
Because Car
class is an extension of the Vehicle
class, it has access to the move()
method.
This is why the code above works even though the Car
class is an empty class.
This is just a glance at how inheritance works. If you want to learn more about PHP inheritance, check out my PHP inheritance explained article.
Example of an interface in PHP
An interface is a set of rules or a contract that defines the methods that a class should implement.
A class that implements an interface is required to implement all the methods defined in the interface, with the same method signatures (parameters and return values).
Below is a simple example of an interface in PHP:
interface Shape {
function getArea(): float;
}
Once created, the interface can be implemented by a class using the implements
keyword:
class Circle implements Shape {
public $radius;
function __construct($radius) {
$this->radius = $radius;
}
// implement the getArea() method of the Shape interface
function getArea(): float {
return pi() * pow($this->radius, 2);
}
}
// create an object to test
$circle = new Circle(5);
echo $circle->getArea();
// Output: 78.539816339745
In the above example, the Shape
interface defines a getArea()
method with float
data type as its return value.
Because the Circle
class implements the Shape
interface, the getArea()
method must be defined in that class.
This is how an interface works. It provides a set of guidelines that the implementing class should fulfill, or PHP will throw an error.
By using an interface, you can design classes with a clear understanding of the required behavior, which can improve the quality and maintainability of the code.
Conclusion
Polymorphism is a powerful concept that allows for more flexible and extensible code by allowing objects of different classes to be treated interchangeably.
In PHP, polymorphism is achieved through inheritance and interfaces:
Inheritance is a way for classes to inherit the properties and methods of another class, allowing the child classes to reuse and extend the functionality of their parent class.
Interfaces, on the other hand, are used to define a contract: a list of methods that a class should implement.
This allows multiple classes to implement the same set of methods, without requiring those classes to inherit from a common parent class.
The use of polymorphism can make your PHP code more modular, scalable, and maintainable.