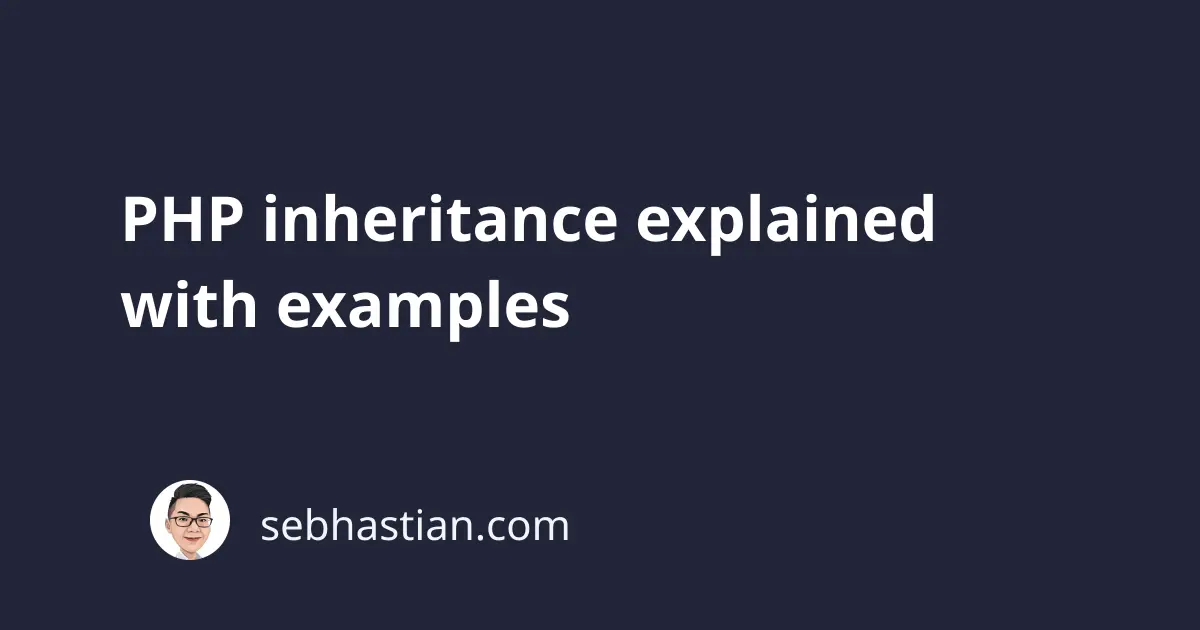
Inheritance is a concept in object-oriented programming that allows a class to inherit the properties and methods of another class.
The inheritor is commonly called a child class, while the original class is called a parent class.
Inheritance allows child classes to reuse and extend the functionality of parent classes, allowing you to write more concise and flexible code.
This article will help you learn the basics of inheritance in PHP so that you can create and extend classes.
PHP inheritance code example
To create a child class that inherits from a parent class in PHP, you need to use the extends
keyword.
Consider the example below:
// Create a class
class Vehicle {
public $speed;
function __construct($speed) {
$this->speed = $speed;
}
function move() {
echo "Moving " . $this->speed . " miles per hour.\n";
}
}
// this class inherits the Vehicle class
class Car extends Vehicle {
public $brand;
function __construct($speed, $brand) {
// initialize properties
$this->speed = $speed;
$this->brand = $brand;
}
}
In this example, the Car
class inherits from the Vehicle
class and extends its functionality by adding a brand
property.
The Car
class is also able to initialize the speed
property in its constructor class, even when there’s no speed
property defined in the class.
To create an object of the Car
class, we call the class constructor and pass the required parameters:
$car = new Car(40, "Tesla");
The code above creates a Car
object with the given speed
and brand
properties.
An object of the Car
class is also an instance of the Vehicle
class, even though it has additional properties and behavior.
We can access the inherited properties and methods of the Vehicle
class on the Car
object, as well as the brand
property of the Car
class:
echo $car->speed . "\n"; // Output: 40
echo $car->brand . "\n"; // Output: Tesla
$car->move();
// Output: Moving 40 miles per hour.
And that’s how you do basic inheritance in PHP.
Next, let’s see how you can override the methods of the parent class.
Overriding methods in PHP
In PHP, a child class can override a method of a parent class by defining a method with the same name and signature in the child class.
When a method is overridden, the implementation of the method in the child class will take precedence over the implementation of the method in the parent class.
For example, let’s override the move()
method of the Vehicle
class in the Car
class like this:
class Vehicle {
function move() {
echo "The vehicle is moving fast!";
}
}
class Car extends Vehicle {
// also define the move() method here
function move() {
echo "The car is moving very slow...";
}
}
// create an object of the Car class
$car = new Car();
// call the move() method
$car->move();
// output: The car is moving very slow...
In the example above, the Car
class overrides the move()
method of the Vehicle
class by providing a different implementation.
When you call the move()
method on the Car
object, PHP will call the overridden method instead of the original implementation.
Inheritance with abstract classes
Aside from a normal class, PHP allows you to create a special type of class called an abstract class.
An abstract class is like an interface, but it can contain abstract and non-abstract methods.
To learn more, see my PHP abstract class explained article.
Disable inheritance with the final modifier
When you want to prevent a class from being inherited, you can add the final
modifier keyword to that class.
See the example below:
// define a final class
final class Vehicle {}
// try to extend the final class
class Car extends Vehicle {}
The example above will cause a fatal error: Class Car may not inherit from final class (Vehicle)
.
You can also put the final
modifier in a method to prevent that method from being overridden:
// define a class with a final method
class Vehicle {
// declare a final method
final function move() {
echo "The vehicle is moving!";
}
}
class Car extends Vehicle {
// 👇 this causes error
function move() {
echo "The new car can move!";
}
}
The code above will cause a Fatal error: Cannot override final method Vehicle::move()
.
Depending on your requirements, you can use the final
modifier to disable a class from being extended.
Conclusion
Inheritance is a powerful concept in object-oriented programming that helps you write extendable code.
In PHP, we can use the extends
keyword to create a child class that inherits from a parent class.
The child class will have access to the properties and methods of the parent class. It can also override the methods already implemented in the parent class.
Now you’ve learned how PHP inheritance works with several code examples. Good work!