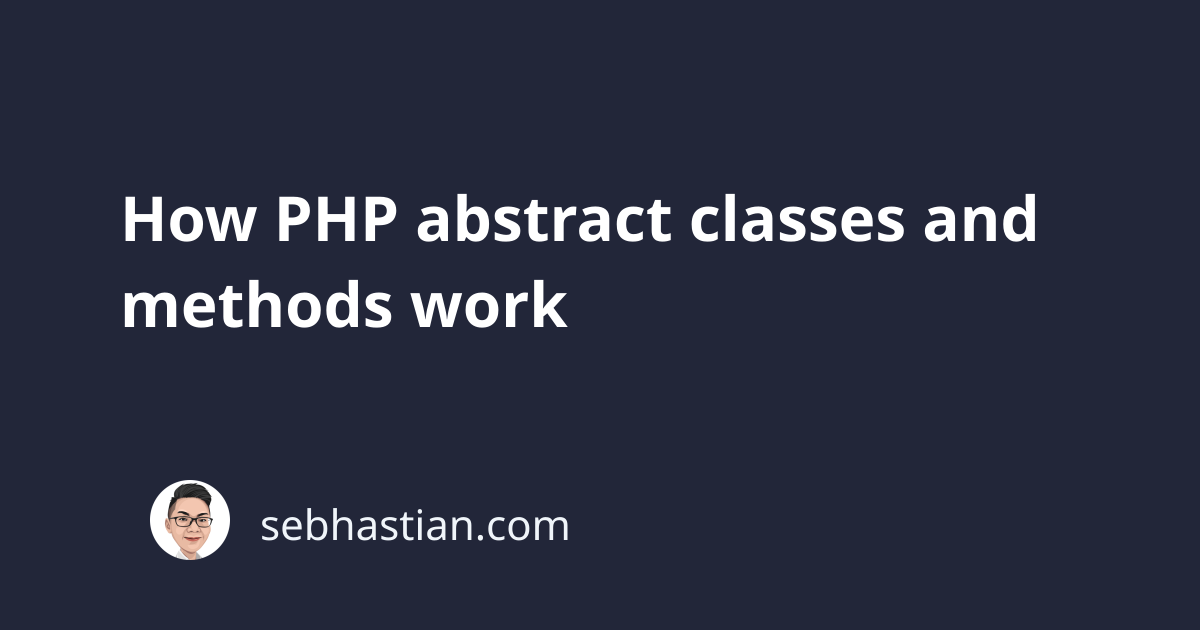
An abstract class is a special type of class that cannot be instantiated on its own. Instead, it serves as a base class for other classes to inherit from.
An abstract class can contain abstract methods. These are methods that are declared without implementation.
Any child class that inherits a class with abstract methods must provide its own implementation.
Let’s see how you can create and inherit an abstract class in this tutorial.
Creating an abstract class
To create an abstract class in PHP, add the abstract
keyword before the class
keyword in the class declaration. For example:
// define an abstract class
abstract class Vehicle {
// declare an abstract method
abstract public function move();
}
In this example, the Vehicle
class is declared as an abstract class and contains an abstract method called move()
.
This means that the Vehicle
class cannot be instantiated on its own. You need to create a child class to instantiate a Vehicle
object.
Inheriting an abstract class
To create a child class that inherits from an abstract class, you need to use the extends
keyword.
If the abstract class has one or more abstract methods, you need to provide an implementation for the abstract method(s).
See the example below:
// define an abstract class
abstract class Vehicle {
public $speed;
public function __construct($speed) {
$this->speed = $speed;
}
// declare an abstract method
abstract public function move();
// declare a normal method
public function start() {
echo "Start the engine..\n";
}
}
// define a class that inherits from Vehicle
class Car extends Vehicle {
public function __construct($speed) {
$this->speed = $speed;
}
// implement move() method
public function move() {
echo "Moving " . $this->speed . " miles per hour.\n";
}
}
In the above example, the Car
class extends the Vehicle
class and provides an implementation for the abstract move()
method.
Meanwhile, the start()
method is a non-abstract method so you don’t need to provide a definition in the Car
class.
When you create a Car
object, you can call both move()
and start()
methods as shown below:
$car = new Car(40);
$car->move(); // Output: Moving 40 miles per hour.
$car->start(); // Output: Start the engine..
In this example, we created an object of the Car
class and called the move()
and start()
methods on the object.
The move()
method is inherited from the abstract Vehicle
class, but the implementation is provided by the Car
class.
Meanwhile, the start()
method is already implemented.
Abstract class vs interface
While they are quite similar, an abstract class is different from an interface.
An abstract class can contain both abstract and non-abstract methods. You can write method implementation in an abstract class.
On the other hand, an interface only contains abstract methods. You will trigger an error when you write an implementation of a method in an interface.
This means that an abstract class can provide some default behavior for its child classes, while an interface cannot.
Conclusion
Abstract classes can be useful in situations where you want to define common methods that characterize a group of related classes.
Unlike an interface, an abstract class allows you to write abstract and normal methods.
This gives you more flexibility in designing a series of classes that are similar but has different purposes.
Now you’ve learned how abstract classes work in PHP. Great!