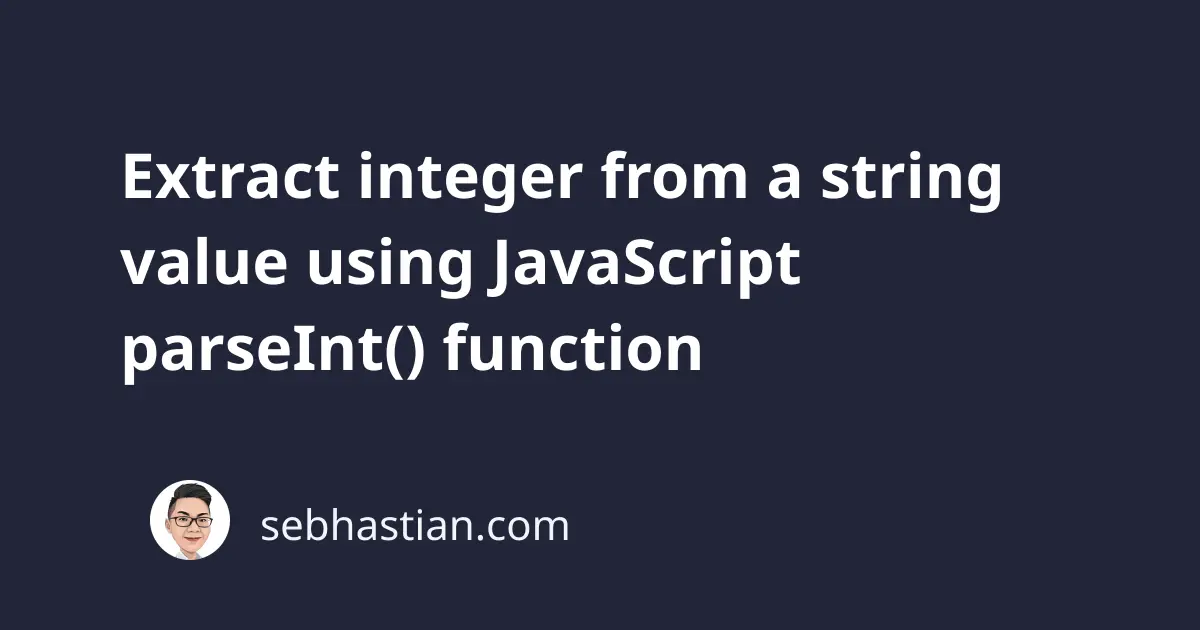
The JavaScript parseInt()
function is used to get an integer value that can be extracted from other value types.
One of the most common uses of the parseInt()
function is to get an integer from a string
value as shown below:
let parsed = parseInt("50");
console.log(parsed); // 50
console.log(typeof parsed); // "number"
As you can see, the type of parsed
variable is a number
instead of a string
.
The parseInt()
method will return a NaN
value when the given argument doesn’t have a valid integer representation.
The example below shows the parseInt()
result of a boolean
value and an empty string:
let parseA = parseInt(true);
let parseB = parseInt("");
console.log(parseA); // NaN
console.log(parseB); // NaN
When you pass an array of numbers, the parseInt()
function will return the first element only.
This is because the function immediately returns the first integer value it can found from your argument:
let parseA = parseInt([28, 37, 61]);
console.log(parseA); // 28
let parseB = parseInt("32 years 8 months old");
console.log(parseB); // 32
The parseInt()
function accepts a second number
argument between 2
and 36
representing the base (or radix
) numeral system used by the first argument.
For example, when you want to convert a binary number string to an integer, you can pass 2
as the function’s second argument:
let parseA = parseInt("1101", 2);
console.log(parseA); // 13
let parseB = parseInt("010", 2);
console.log(parseB); // 2
The binary numbers 1101
and 010
are converted to their base 10 representation with the parseInt()
function.
When you omit the base number argument, the parseInt()
function will consider the strings to be base 10 numbers.
You can also make the function think the argument is a base 16 number by adding 0X
before the string as shown below:
let parseA = parseInt("FE8");
console.log(parseA); // NaN
let parseB = parseInt("0XFE8");
console.log(parseB); // 4072
The hexadecimal number FE8
represents the number 4072
in the base 10 system.
Without 0X
in front of the values, parseInt()
will consider the string to be a base 10 number. And the result is a NaN
value.
Finally, the parseInt()
function always return a whole number from any value you specify as its argument:
let parseA = parseInt("5.962");
console.log(parseA); // 5
let parseB = parseInt("32.07");
console.log(parseB); // 32
If you want a number with decimal points, you need to use the parseFloat()
function instead of parseInt()
.
Consider the example below:
let parseA = parseFloat("5.962");
console.log(parseA); // 5.962
let parseB = parseFloat("21");
console.log(parseB); // 21
Now you’ve learned how to use the JavaScript parseInt()
function. Great job! 😉