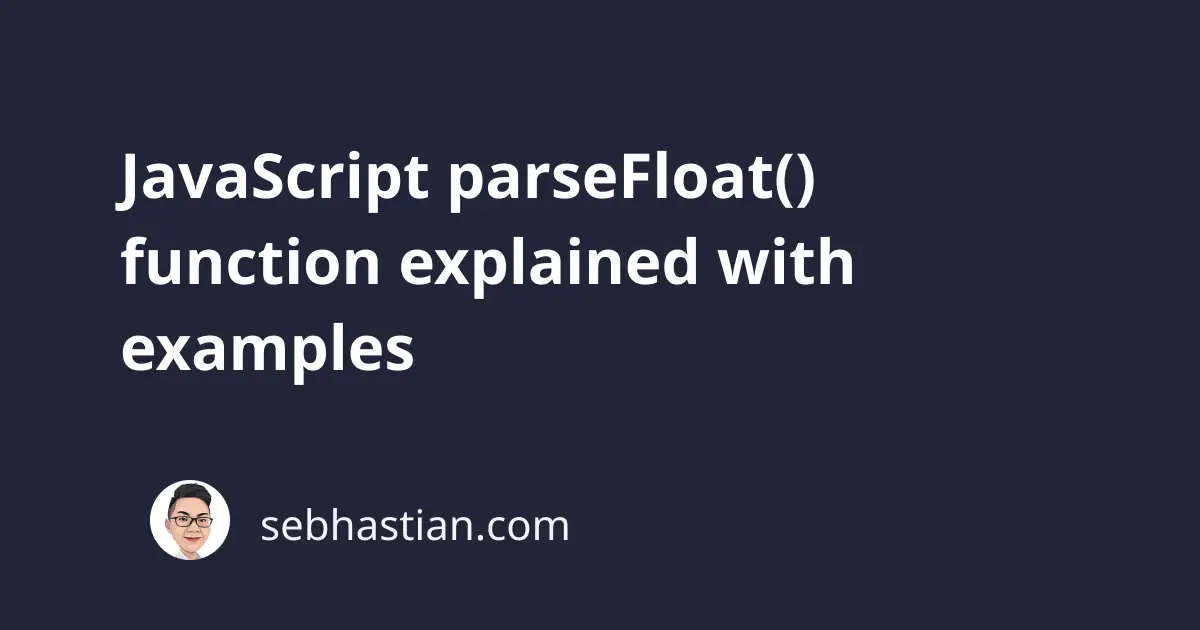
The JavaScript parseFloat()
function is a built-in function that returns a floating-point number from the given string
argument.
The function allows you to extract a number
from a string
as shown below:
console.log(parseFloat("12.57.a ")); // 12.57
When a number can’t be parsed from the argument, a NaN
will be returned by the function:
console.log(parseFloat("abc ")); // NaN
The parseFloat()
function can’t adjust the number of digits for the decimal point.
When you need a certain amount of decimal point digits, you need to use the toFixed()
method.
See also: Rounding numbers with toFixed() method
For example, suppose you only want 2 decimal point digits out of the string
number. Here’s how you do it:
let string = "37.5930"
let float = parseFloat(string);
let fixedFloatString = float.toFixed(2); // "37.59"
let fixedFloat = parseFloat(fixedFloatString);
console.log(fixedFloat); // 37.59
The parseFloat()
function is called two times because the toFixed()
method returns a string
representing the number where you call the function from.
If you don’t need a number
type representation of the number, you can omit the last parseFloat()
function call.
Use isNaN() to check the returned value of parseFloat()
Finally, the parseFloat()
function returns a NaN
when the given argument has no valid number representation.
Since a NaN
isn’t a useful value, you might want to replace it with 0
in some cases.
Consider the following example code:
function multiplyByTwo(x) {
if (isNaN(parseFloat(x))) {
x = 0
}
return parseFloat(x) * 2;
}
console.log(multiplyByTwo("abc")) // 0
console.log(multiplyByTwo("3.21`")) // 6.42
When the value of parseFloat(x)
is NaN
, the x
variable will be replaced with 0
instead.
This way, the calculation of parseFloat(x) * 2
will always return a number 0
in place of NaN
.
Now you’ve learned how the parseFloat()
function works in JavaScript. Good work! 😉