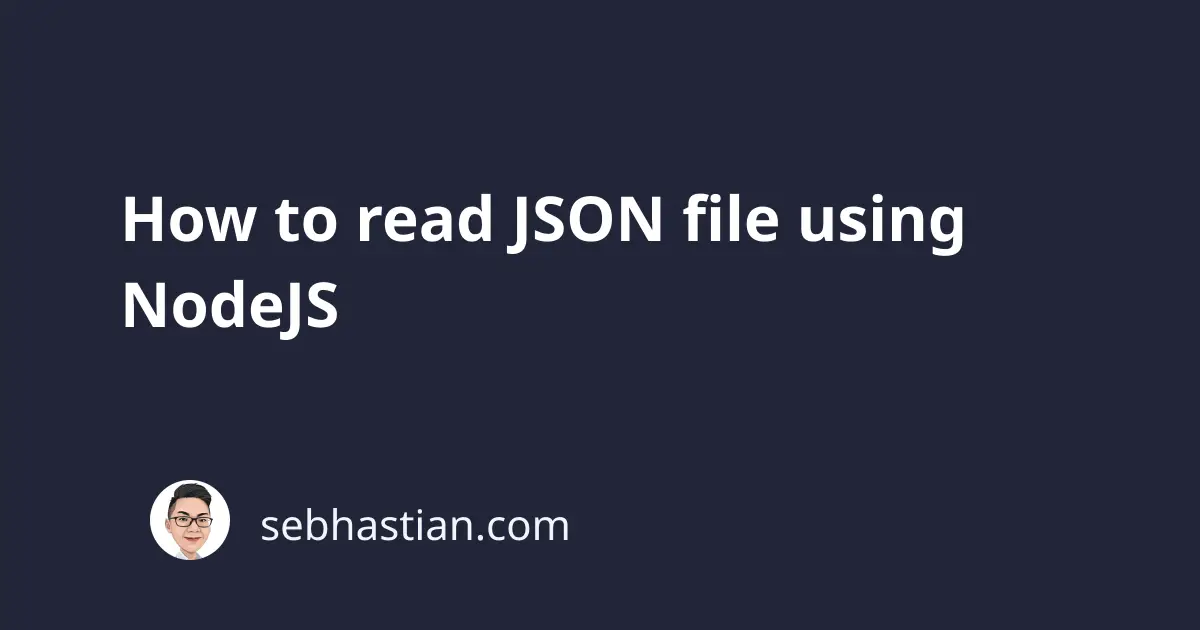
This tutorial will help you to learn two different ways to read JSON data from a .json
file using NodeJS.
The easiest way to read a .json
file content is to import the file using the require()
function.
Suppose you have the following content inside your data.json
file:
{
"firstName": "Nathan",
"lastName": "Sebhastian"
}
You can require()
the .json
file in your JavaScript file and use the object property as follows:
const obj = require("./data.json");
console.log(obj.firstName); // Nathan
console.log(obj.lastName); // Sebhastian
The require()
function automatically use JSON.parse()
method to parse your JSON file content so you can immediately retrieve the property values.
But keep in mind that importing the JSON file using require()
will cache the content of the file. If you change the content of your JSON file, you need to restart your NodeJS runtime.
This method is ideal for loading short config.json
file that you may need for JavaScript build tools like Grunt or Webpack.
For reading a large JSON file (more than 10 MB in size) it’s better to use the fs.readFile()
method because of its asynchronous nature.
Reading JSON file using fs.readFile() method
To read a large JSON file, you can use the readFile()
method from the built-in fs
module.
Here’s an example of reading a large JSON file using the method:
const fs = require("fs");
fs.readFile("./large-file.json", "utf8", (err, response) => {
if (err) {
console.error(err);
return;
}
const data = JSON.parse(response);
console.log(data); // your JSON file content as object
});
Because the readFile()
method is asynchronous, NodeJS will continue to execute your JavaScript code without waiting for the read process to finish.
You can learn more about the readFile()
and readFileSync()
method here:
NodeJS - read file content using the fs module
And that’s how you can read JSON file content using NodeJS 😉