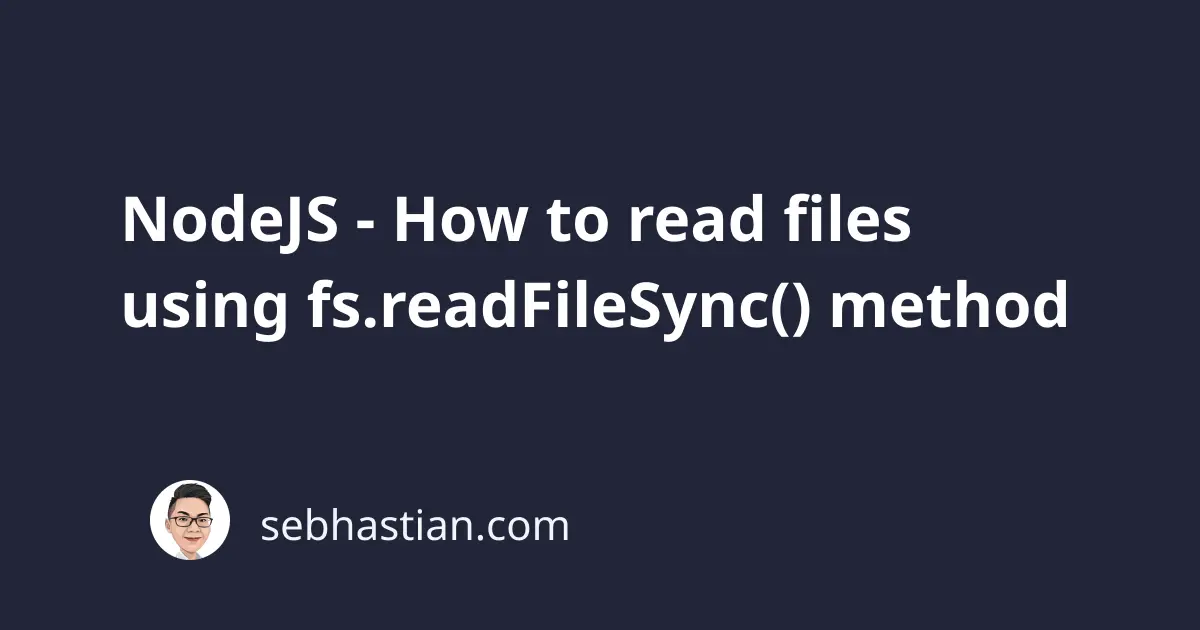
When you need to read the content of a file using NodeJS, you can use the built-in fs
module which contains readFile()
and readFileSync()
methods. This tutorial will show you how to use both.
Read files using readFileSync() method
The readFileSync()
method will read the content of a file synchronously, so your JavaScript code execution will be stopped until the method is finished.
The readFileSync()
method accepts two parameters:
path
- mandatory - which is the relative path to the file you want to read (string
type)options
- optional - the encoding format of the file content to read with (string
orobject
type)
You can also use an object
for the options
parameter to pass both the encoding and the operation flag as follows:
fs.readFileSync("./data.txt", "utf8");
// or
fs.readFileSync("./data.txt", { encoding: "utf8", flag: "r" });
Suppose you have a file named data.txt with the following content:
Hello World
Greetings from data.txt
Hope you have a great day
And a long blessed life
Here’s how you read the file using readFileSync()
method:
const fs = require("fs");
const path = "./data.txt";
try {
const data = fs.readFileSync(path, "utf8");
console.log("File content:", data);
} catch (err) {
console.error(err);
}
The output in the console will be as shown below:
File content: Hello World
Greetings from data.txt
Hope you have a great day
And a long blessed life
Without the "utf8"
encoding, the method will return the raw buffer as follows:
File content: <Buffer 48 65 6c 6c 6f 20 57 6f 72 6c 64
0a 47 72 65 65 74 69 6e 67 73 20 66 72 6f 6d 20 64 61 74
61 2e 74 78 74 0a 48 6f 70 65 20 79 6f 75 20 68 61 76 65
20 ... 35 more bytes>
When you need the output in a human-readable format, don’t forget to include the encoding option.
Next, let’s look at the readFile()
method.
Read files using readFile() method
The fs.readFile()
method enables you to read the content of a file asynchronously, which means the execution of JavaScript code will continue down the line without waiting for the method to finish.
Just like readFileSync()
method, the readFile()
method accepts the path
and options
parameter, along with a callback
function that will be called when the function is finished.
Here’s the syntax of readFile()
method:
fs.readFile("./data.txt", "utf8", function (err, data) {
if (err) {
console.error(err);
return;
}
console.log(data);
});
You’ve just learned how to read the content of a file using NodeJS. Both readFile()
and readFileSync()
method buffers the entire file content in the memory before displaying the output, so it might take a long time to read a large file.
You may also run out of memory when the memory allocated for NodeJS is small, so you may want to read your file using the streaming method instead of buffering.
To stream a file’s content, you can use the createReadStream()
method as show below:
const fs = require("fs");
const readStream = fs.createReadStream("./data.txt", "utf8");
readStream
.on("data", function (chunk) {
console.log(chunk);
})
.on("end", function () {
console.log(">> The stream is finished");
});
With that, the stream will log each file chunk to the console instead of logging all the content at once.