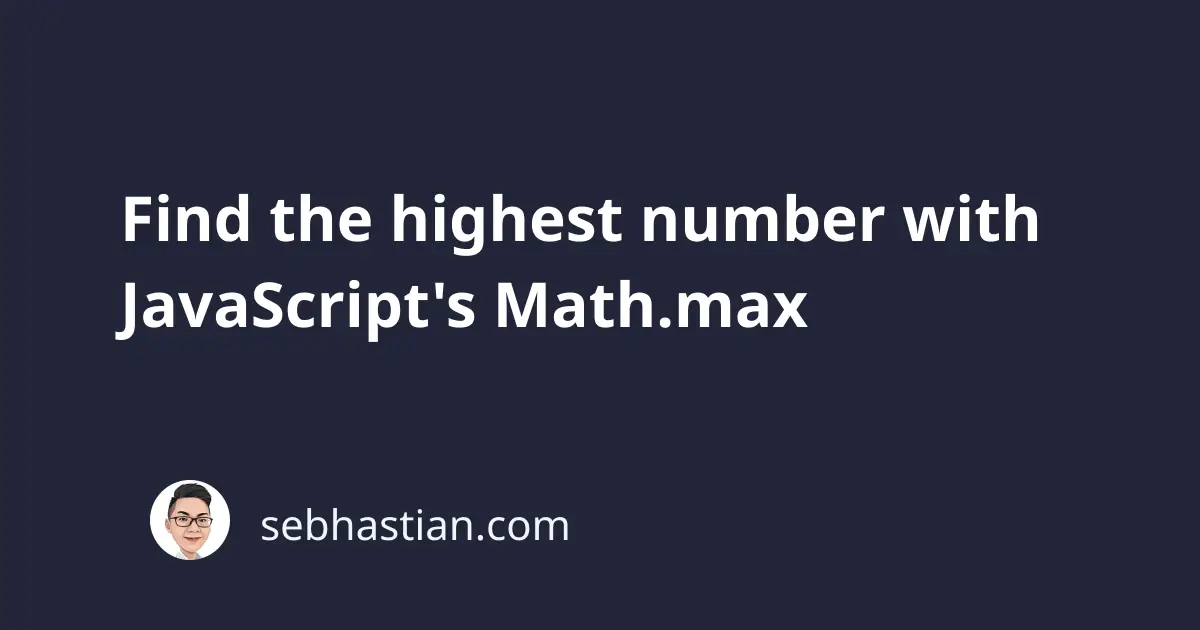
When you need to find the highest value of several numbers, you can use JavaScript’s Math.max
method. The method accepts as many number arguments as you need to pass into it:
Math.max(20, 3, 10, 29);
// returns 29
Passing zero arguments will cause the method to return -Infinity
as value:
Math.max();
// returns -Infinity
Passing any other data type as arguments will cause the method to return NaN
:
Math.max("Hello", 1, 2);
// returns NaN
You can also mix the arguments for the method with negative numbers:
Math.max(7, -9, -25);
// returns 7
Finding the maximum value in an array
When you need to find the maximum value in an array of numbers, you can use the spread
operator (...
) to pull out the numbers and pass them as arguments into the Math.max
method:
let scores = [7, 4, 6, 8];
let maxNumber = Math.max(...scores);
// maxNumber value is 8
Or you can also use the reduce
method to compare two elements at a time:
let scores = [7, 6, 5];
let max = scores.reduce(function(a, b) {
return Math.max(a, b);
});
The reduce
method above will perform two times:
- Compare between
7
and6
, returns7
- Compare between
7
and5
, returns7
And so the max
value is 7