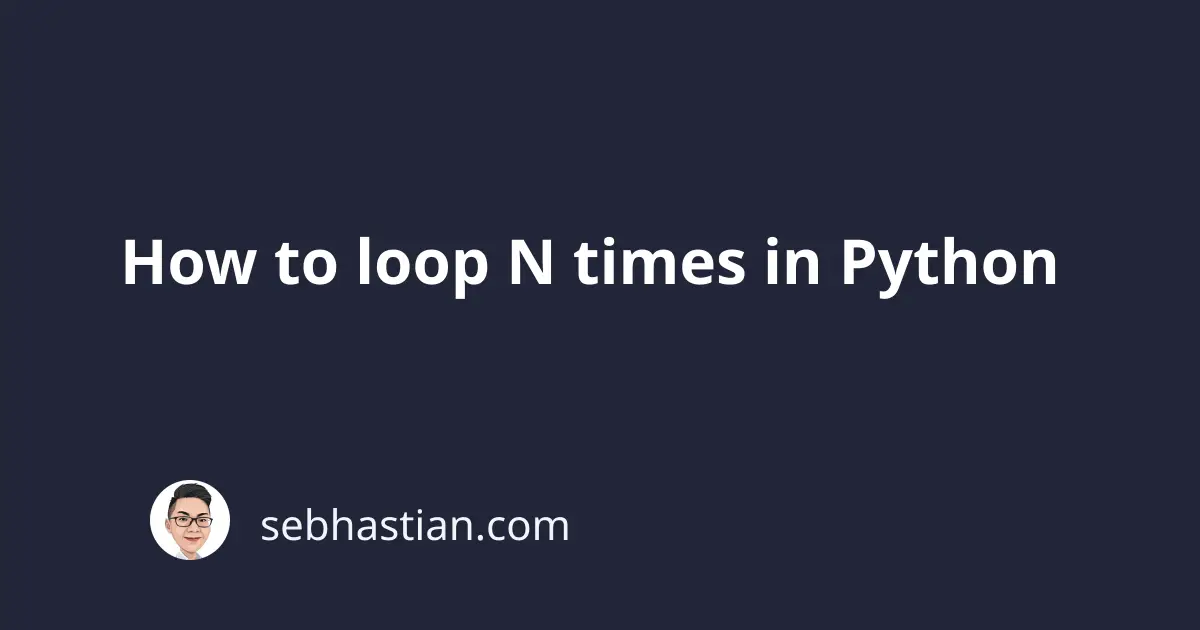
Looping for N times in Python can be done using both the for
and while
loops.
This tutorial will show you how to use both loops in practice.
1. Loop N times using the for loop
The most preferred method to loop N times is to use the for
loop and range()
function.
The range()
function allows you to specify a range of numbers stored as a range object, which you can iterate over using a for
loop.
For example, here’s how to loop 3 times in Python:
for i in range(3):
print(i, "Hello")
Output:
0 Hello
1 Hello
2 Hello
Note that the i
variable becomes the number 0
, 1
, and 2
in each iteration because it’s the number inside the range object.
If you want to loop 5 times, just pass 5
to the range()
function:
for i in range(5):
print(i, "Howdy!")
0 Howdy!
1 Howdy!
2 Howdy!
3 Howdy!
4 Howdy!
Next, let’s see how you can loop for N times using the while
loop.
2. Loop N times using the while loop
When you need to loop for N times using the while
loop, you need to set a counter
variable that keeps track of the iteration in your loop.
You can set the counter from 0
, and increment it by 1 each time the iteration is finished.
To repeat the while
loop N times, just specify counter < N
as the condition for the loop. For example, here’s how to loop 3 times using the while
loop:
counter = 0
while counter < 3:
print(counter, "Good morning!")
counter += 1
Output:
0 Good morning!
1 Good morning!
2 Good morning!
And here’s how to repeat for 5 times:
counter = 0
while counter < 5:
print(counter, "Good!")
counter += 1
Output:
0 Good!
1 Good!
2 Good!
3 Good!
4 Good!
Now you’ve learned how to loop for N times using the while
loop.
Conclusion
You can use both the for
and while
loops to loop for N times in Python.
If you need to loop as many times as the length of a list, you can use the range(len())
syntax as shown in this article.
I hope this tutorial is helpful. Happy coding! 👍