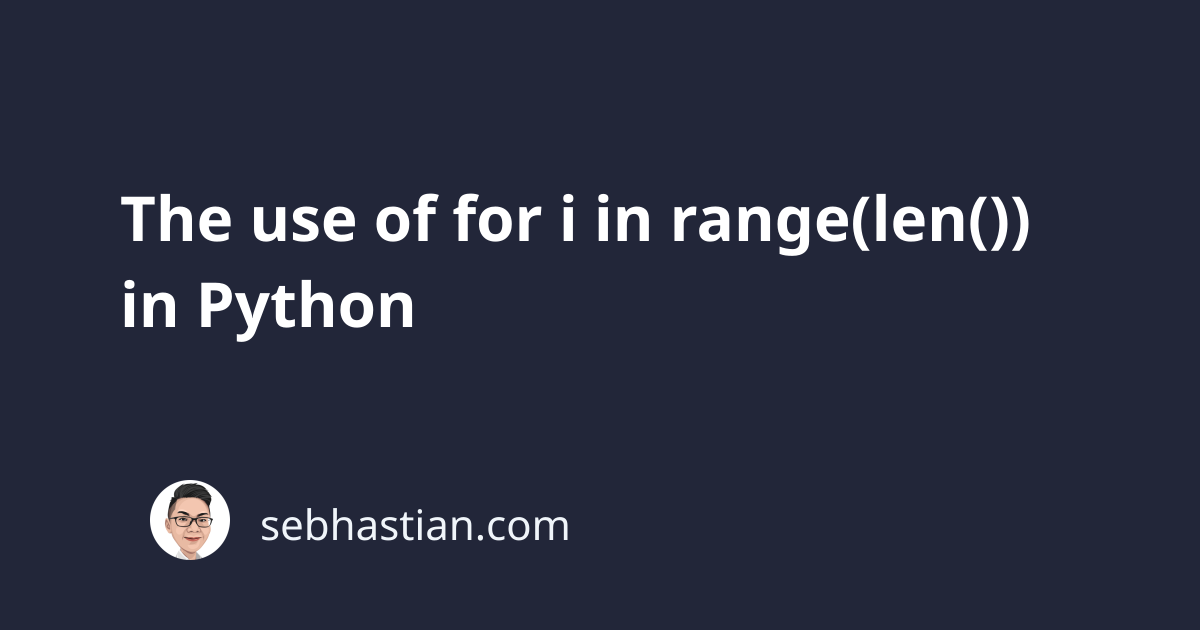
When iterating over an iterable object such as a list, you’ve probably seen the use of for i in x
and the for i in range(len(x))
syntax.
You might wonder, why some people use for i in range(len(x))
instead of for i in x
?
The answer is that the syntax that use the range()
and len()
functions are used when you need to access the indices of a sequence.
As you might know, the iterable object such as a list stores the index position of the items using integers that starts from 0.
Using the range(len(x))
syntax allows you to access these index numbers as shown below:
x = ['a', 'b', 'c']
print("Items in x:")
for item in x:
print(item)
print("Indices in x:")
for index in range(len(x)):
print(index)
Output:
Items in x:
a
b
c
Indices in x:
0
1
2
This brings up a further question: Why do you need to access the indices of a list?
This depends on what you want to achieve with your program, but generally there are two reasons:
- You need to modify the original list
- You need to access more than just the current item inside the iteration
Let’s see an example that requires the use of range(len(x))
in practice.
1. You want to modify the original list
When you need to modify the original list, you need to access the list using the index numbers.
In the example below, we attempt to modify the original list by adding the character z
to each item:
x = ['a', 'b', 'c']
for item in x:
item = item + "z"
print(x) # ['a', 'b', 'c']
At first glance, the for
loop in the example above seems to correctly modify the item
in the list x
, but the operation simply re-assigns the item
variable without modifying the original list.
To modify the original list, you need to get the indices of the list by using the range()
and len()
functions like this:
x = ['a', 'b', 'c']
for index in range(len(x)):
x[index] = x[index] + "z"
print(x) # ['az', 'bz', 'cz']
To modify the list x
, you need to access the list inside the for
loop. But if you iterate over the items with for i in x
, then there’s no way you can specify the index numbers in the loop body.
By using the range(len(x))
syntax, the original list x
can be modified inside the for
loop correctly.
There’s also another way to access the item and index in a list, which is to use the enumerate()
function as follows:
x = ['a', 'b', 'c']
for index, _ in enumerate(x):
x[index] = x[index] + "z"
print(x) # ['az', 'bz', 'cz']
The enumerate()
function returns a pair containing the index and the item of the list. Since we don’t use the item, we assigned the _
to the second pair.
You’re free to use the syntax you liked best.
2. You want to access more than just the current item inside the iteration
Sometimes, you want to access more than just the current item inside the for
loop.
For example, suppose you want to get numbers that are less than the next number in a list:
numbers = [1, 5, 2, 8, 3, 9, 4]
result = []
for i in range(len(numbers)-1):
if numbers[i] < numbers[i+1]:
result.append(numbers[i])
print(result) # [1, 2, 3]
In this example, we need to iterate over the list except for the last item, so we set the iteration range to len(numbers)-1
.
Next, we created an if
statement that checks if the current item is less than the next item. The next item is accessed using the numbers[i+1]
syntax.
When the if
statement returns True
, we append the current item to the result
list.
As you can see, we won’t be able to compare the item inside the iteration with the next one if we didn’t use the range()
and len()
functions.
Of course, you can also use the enumerate()
function as an alternative like this:
numbers = [1, 5, 2, 8, 3, 9, 4]
result = []
for index, _ in enumerate(numbers[0:-1]):
if numbers[index] < numbers[index+1]:
result.append(numbers[index])
print(result) # [1, 2, 3]
Note that the enumerate()
function requires you to slice the list to exclude the last item with [0:-1]
, But both solutions worked just fine.
Conclusion
The for i in range(len(x))
syntax is used when you encounter unique cases that require you to modify the original list or you want to access more than just the current item in the iteration.
Most of the time, the syntax can be replaced with the enumerate()
function, which also handles cases where you need more than just the item inside the iteration.
Personally, the use of range(len(x))
gives me more clarity, so I usually use that instead of enumerate()
.
But that’s just my preference, so you can use whichever you like.
I hope this tutorial is helpful. Until next time! 👋