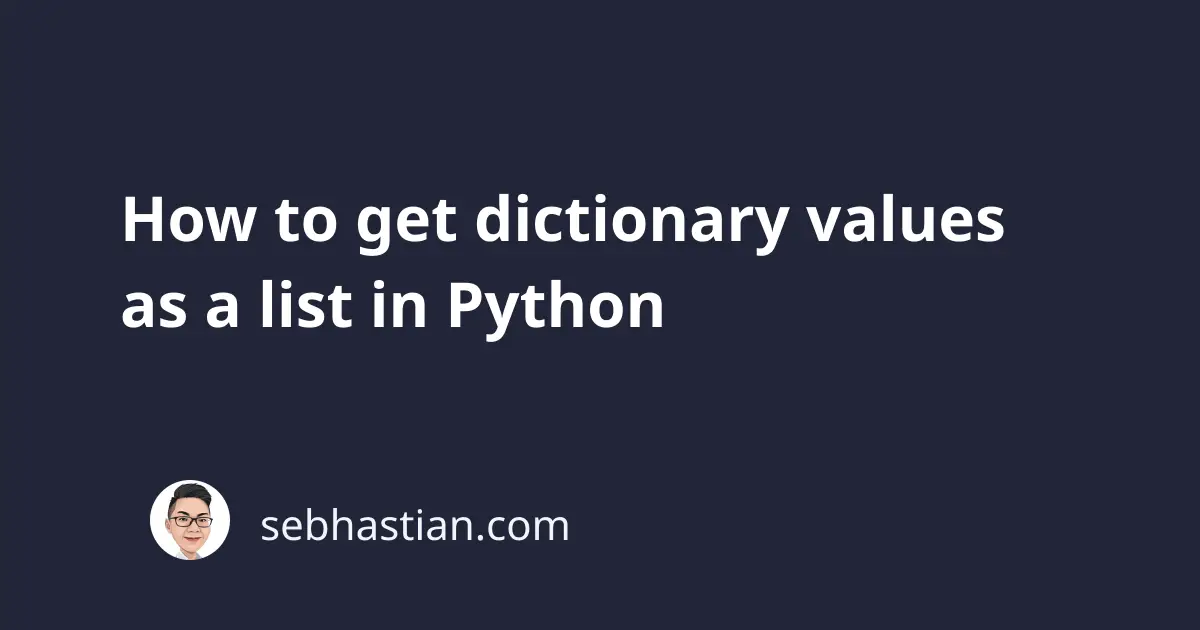
When you need to get the values of a dictionary as a list, there are 4 solutions you can use:
- Using the
list()
function anddict.values()
method - Unpack the values as a list using the asterisk
*
symbol - Using a list comprehension
- Using a
for
loop
I will show you how to use these 4 solutions in practice
1. Using list() and dict.values() method
To extract the values from a dictionary, you can use the dict.values()
method. After that, you only need to call the list()
function to convert the output to a list
Here’s an example:
organization = {
'ceo': 'Nathan',
'coo': 'Andy',
'cfo': 'Johnny'
}
my_list = list(organization.values())
print(my_list)
Output:
['Nathan', 'Andy', 'Johnny']
As you can see, the solution successfully extracted the values from the dictionary.
2. Unpack the values as a list using the asterisk *
symbol
As an alternative, you can also unpack the values as a list using the asterisk *
symbol as follows:
organization = {
'ceo': 'Nathan',
'coo': 'Andy',
'cfo': 'Johnny'
}
my_list = [*organization.values()]
print(my_list)
Output:
['Nathan', 'Andy', 'Johnny']
The starred expression unpacks the values from the dict_value
iterable object, and we used a list []
to store the unpacked values.
3. Using a list comprehension
Another way to extract values from a dictionary is to use a list comprehension and the dict.values()
method:
organization = {
'ceo': 'Nathan',
'coo': 'Andy',
'cfo': 'Johnny'
}
my_list = [i for i in organization.values()]
print(my_list)
Output:
['Nathan', 'Andy', 'Johnny']
Similar to the starred expression, the list comprehension uses a for
loop to extract each item in the dict_values
object to a list.
4. Using a for
loop
You can also use a for
loop to iterate over the dictionary itself:
organization = {
'ceo': 'Nathan',
'coo': 'Andy',
'cfo': 'Johnny'
}
my_list = []
for i in organization.values():
my_list.append(i)
print(my_list)
Output:
['Nathan', 'Andy', 'Johnny']
In this solution, you need to create an empty list before running the for
loop.
And that’s how you get the dictionary values as a list in Python. You might also want to check my other tutorial on getting the first key in a Python dictionary.