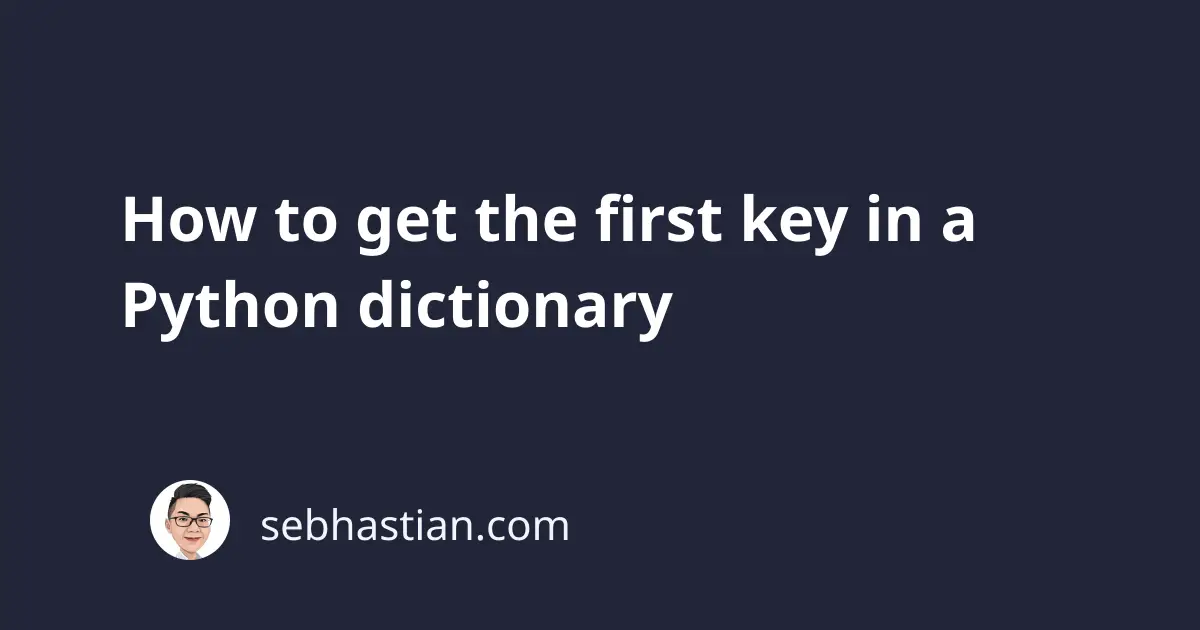
Unlike a list
object, the Python dict
object isn’t indexed, so you can’t use the subscript operator []
to access the first key of a dictionary.
Depending on the Python version you used, there are two ways to get the first key of a Python dict
object:
- Use the combination of
next()
anditer()
to get the first key. - Use the
list()
constructor and thedict.keys()
method
This tutorial shows how you can use the two solutions in practice
1. Get the first key in a dictionary with next() and iter()
To get the first key of a dictionary, you can use the next()
function in combination with the iter()
function as follows:
my_dict = { "as": 1, "bz": 2, "cx": 3 }
first_key = next(iter(my_dict))
print(first_key) # as
The iter()
function returns an iterator object of an iterable (a dictionary in this case) and the next()
function is used to return the next item in the iterator.
These two functions allow you to get the first key of a dictionary quickly, but this solution doesn’t work when you need to get the key in other positions besides the first.
2. Use the list() and dict.keys() functions
Another way to get the first key of a dictionary in Python is to use the list()
and dict_keys()
functions to get the dictionary keys as a list.
Consider the following example:
my_dict = {"as": 1, "bz": 2, "cx": 3}
dict_keys = list(my_dict.keys())
# Get the first key in the dict
print(dict_keys[0]) # as
# Get the last key in the dict
print(dict_keys[-1]) # cx
The dict.keys()
method returns a dict_keys
object containing the keys of your dictionary.
After that, the list()
constructor is called to convert the dict_keys
object into a list
object.
By storing the dictionary keys as a list, you’ll be free to get the key in any position and not just the first.
Note for Python version below 3.7
Since Python version 3.7, the dictionary object order is guaranteed to be insertion order, so the first key-value pair you put in the object is fixed.
If you’re using an older Python version, then the order of the key-value pair might not be the same as the insertion order.
You need to initialize the dictionary using the OrderedDict()
constructor from the collections
module as follows:
import collections
my_dict = collections.OrderedDict([
("as", 1),
("bz", 2),
("cs", 3)
])
first_key = next(iter(my_dict))
print(first_key) # as
By using the OrderedDict()
constructor, you created an instance of OrderedDict
that keeps the order of your items.
Conclusion
Python doesn’t memorize the positions of dictionary keys, so you can’t use the subscript operator []
to access a dictionary key.
To get the first key of a dictionary, you can use the next()
and iter()
functions.
If you need to get the keys in other positions, then you need to use the list()
constructor and the dict.keys()
method to get a list of dictionary keys.
Also, keep in mind that you need to use the OrderedDict()
constructor for Python 3.6 and below to guarantee the insertion order of the dictionary object.
I hope this tutorial is helpful. Happy coding! 🙌