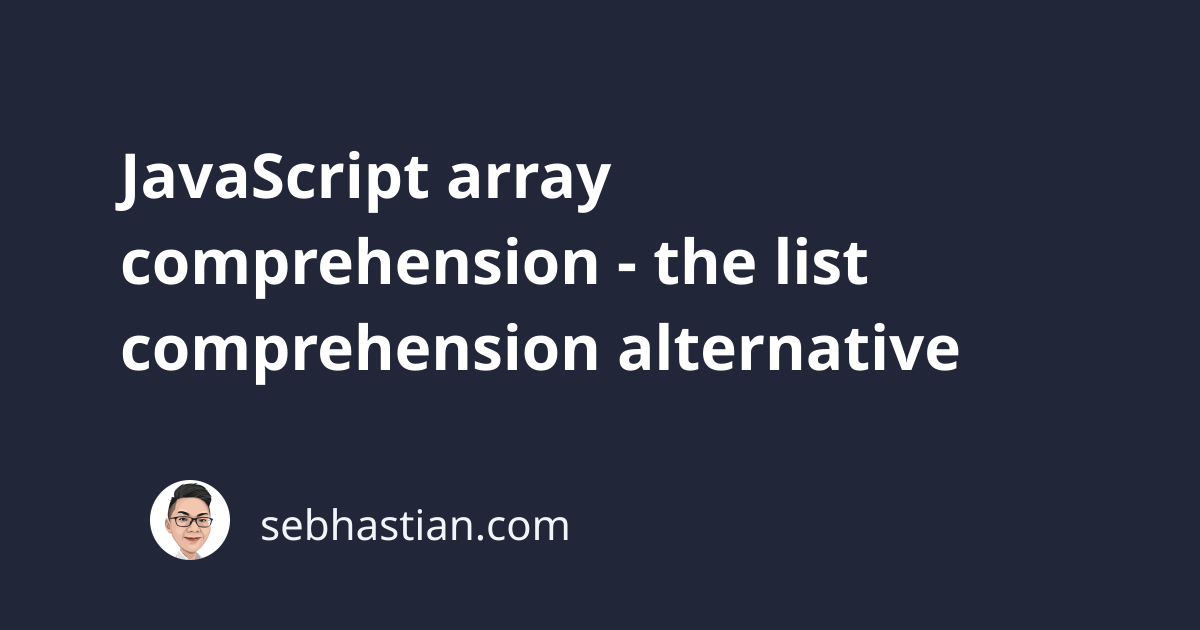
A list comprehension is a certain language syntax available in many programming languages. It is used for creating a new list from an already existing list.
You can think of list comprehension as an elegant way to filter a list.
For example, Python allows you to create a new list from an already existing list. The list is array based as shown below:
animals = ["cow", "bird", "fish", "horse", "tiger"]
newList = [animal for animal in animals if "i" in animal]
print(newList) # ["bird", "fish", "tiger"]
The syntax assigned to the newList
variable is the list comprehension syntax of Python.
It enables you to filter the animals
array and add the element into the newList
variable only when the element has the letter "i"
inside it.
The syntax creates a new list, so the original list is not modified. It’s elegant because you are filtering the list with only one line of code.
If you’re wondering if JavaScript supports a list comprehension syntax like Python, the answer is unfortunately no.
The JavaScript Committee TC39 once considered adding list comprehension to JavaScript, but it was canceled in favor of other JavaScript array methods like filter()
and map()
.
As an alternative to the list comprehension syntax, you can use the filter()
or map()
method with an array. This is also known as the array comprehension syntax in JavaScript.
JavaScript array comprehensions syntax explained
An easy way to replicate Python’s list comprehension is to use the Array.filter()
method available in JavaScript.
The Array.filter()
method allows you to create a new array out of an existing array by applying a certain filter to it.
The filter is a JavaScript expression that returns an array element when it passes the test function you specify in the function body.
Getting back to the Python example, here’s how you can create a newList
out of the animals
array in JavaScript:
let animals = ["cow", "bird", "fish", "horse", "tiger"];
let newList = animals.filter(function (animal) {
return animal.includes("i");
});
console.log(newList); // ["bird", "fish", "tiger"]
The filter creates a new array so the original array is not modified, just like in the Python example.
As you can see, the filter()
method is a good alternative to the list comprehension filter.
You can also reduce the method body into only one line with the arrow function syntax as follows:
let newList = animals.filter(animal => animal.includes("i"));
Next, let’s see how you can iterate through a list using the map()
method.
JavaScript array comprehension with map
Another use of list comprehension is to iterate through a list and do an operation to each element repeatedly.
For example, the following Python code adds the letter "i"
to each element in the list:
animals = ["cow", "horse", "tiger"]
newList = [animal + "i" for animal in animals]
print(newList) # ['cowi', 'horsei', 'tigeri']
You can produce the same output as the code above by using JavaScript’s Array.map()
method.
Just like Array.filter()
method, the Array.map()
method allows you to create a new array from an existing array.
But instead of filtering the existing array, the map()
method simply iterate over the array and allows you to freely execute a piece of code inside the callback function.
The following JavaScript code produces the same output as the Python code above:
let animals = ["cow", "horse", "tiger"];
let newList = animals.map(function (animal) {
return animal + "i";
});
console.log(newList); // ["cowi", "horsei", "tigeri"]
You can use the arrow function syntax to reduce the map()
body into only one line:
let newList = animals.map(animal => animal + "i");
With the map()
method, you can iterate through any JavaScript array and execute a provided callback function, just like a Python list comprehension.
Iterate through array with the for of syntax
Alternatively, you can also use the for of
iteration to iterate through an array as follows:
let animals = ["cow", "horse", "tiger"];
let newList = [];
for (const animal of animals) {
newList.push(animal + "i");
}
console.log(newList); // ["cowi", "horsei", "tigeri"]
In the above example, each iteration creates a new animal
variable that holds the element stored in the array.
Inside the for.. of
body, push each animal
element into the newList
array. You can apply an operation for each element as you need.
Conclusion
Now you’ve learned three different array comprehensions syntax in JavaScript:
- Using the filter function
- Using the map function
- Using a
for of
iteration
You are free to use the syntax you want in your code.
I hope this tutorial has helped you to understand array comprehension syntax in JavaScript.
Thanks for reading!