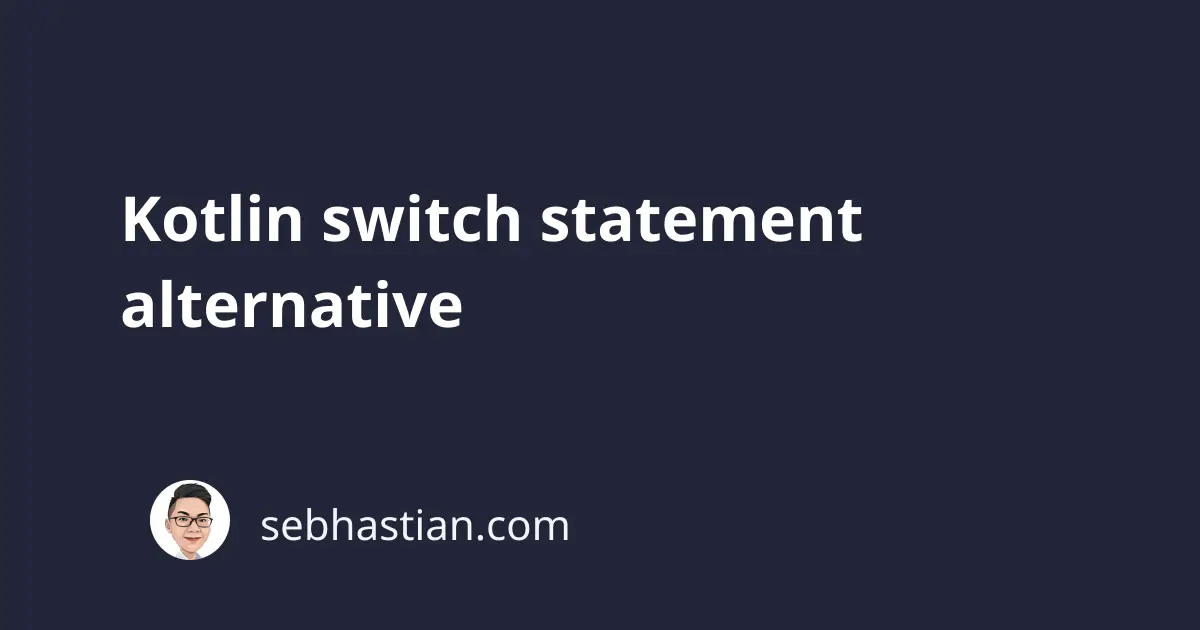
In Kotlin, there’s no switch
-case
statement like the one you might find in the Java programming language.
Instead, Kotlin replaces a switch
statement with the when
keyword.
Consider the following switch
statement in Java:
int myInt = 2;
switch (myInt) {
case 1:
System.out.println("number is one");
break;
case 2:
System.out.println("number is two");
break;
default:
System.out.println("number is unknown");
}
The code above is equivalent to the following Kotlin code:
var myInt = 2
when (myInt) {
1 -> println("number is one")
2 -> println("number is two")
else -> println("number is unknown")
}
The when
keyword brings some improvement to the old switch
keyword of Java.
You don’t need to add the break
keyword for each case, and the default
case is replaced with the else
case.
The when
keyword also allows you to define a common result for multiple cases. You only need to separate the cases that produce the same result with a comma.
For example, the following code in Java has both 1
and 2
produce the same result:
int myInt = 2;
switch (myInt) {
case 1:
case 2:
System.out.println("number is either one or two");
break;
default:
System.out.println("number is unknown");
}
The Kotlin equivalent of the code above will be as follows:
var myInt = 2
when (myInt) {
1, 2 -> println("number is either one or two")
else -> println("number is unknown")
}
Finally, the when
keyword can be used as an expression or a statement.
It means that you can assign the result of the when
keyword into a variable as follows:
var myInt = 2
var myString = when (myInt) {
1, 2 -> "number is either one or two"
else -> "number is unknown"
}
print(myString) // number is either one or two
In Java, the use of switch
keyword is limited to a statement or as a return value of a function.
To assign a value to a variable, you need to create a work-around as follows:
String myString;
switch (myInt) {
case 1:
case 2:
myString = "number is either one or two";
break;
default:
myString = "number is unknown";
break;
}
System.out.println(myString);
In Java JDK 12, improvements are made with the switch
keyword that allows you to use it as an expression as shown below:
int myInt = 2;
var myString = switch (myInt) {
case 1, 2 -> "number is either one or two";
default ->"number is unknown";
};
System.out.println(myString);
But since Android Studio uses JDK 11 by default, using the above code for Android application would likely produce illegal start of expression
error.
You can learn more about the when
keyword here.