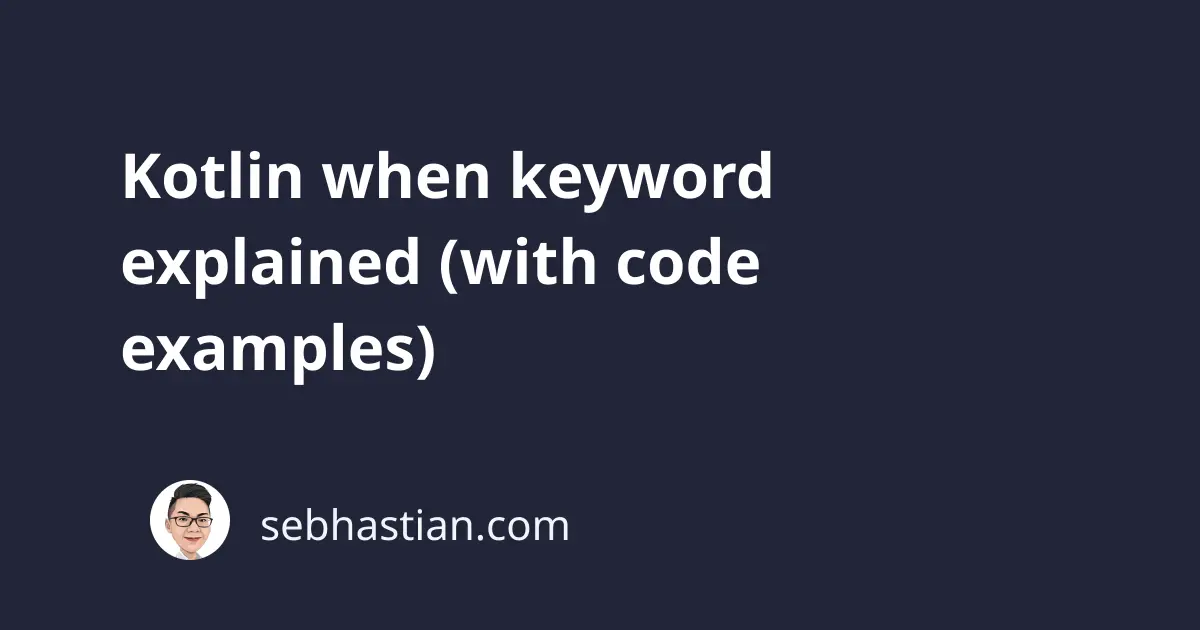
The Kotlin when
keyword is a modern replacement for the switch
statement in traditional programming languages.
The when
expression allows you to create several condition branches that run different pieces of code.
The when
keyword can be used as a statement or an expression, depending on how you write your Kotlin code.
Let’s see how the when
keyword works as a statement first.
Kotlin when keyword as a statement
In the code example below, the when
statement will print
a specific string based on the value of myInteger
variable:
var myInteger: Int = 2
when (myInteger){
1 -> print("Value is one")
2 -> print("Value is two")
}
Because the value of myInteger
variable above is 2
, then the "Value is two"
string will be printed.
You can also combine the else
statement with when
to create a default piece of code to run when none of the other branch conditions are satisfied.
Here’s an example of the else
statement inside a when
expression:
var myInteger: Int = 10
when (myInteger){
1 -> print("Value is one")
2 -> print("Value is two")
else -> print("another number")
}
In the above example, the string "another number"
will be printed as long as the value of myInteger
variable is not 1
or 2
.
When you need to write multiple lines of code for the else
statement, you can use the curly brackets to contain the code as shown below:
else -> {
println("another number")
println("The number is not one or two")
}
You can omit the else
statement when you’re using the when
keyword as a statement.
When there’s no matching conditional branch without an else
statement, Kotlin will skip your when
statement without executing any branch at all.
Now that you’ve learned how when
works as a statement, let’s see how it works as an expression next.
Using when keyword as an expression
The when
keyword can also be used as an expression, which means you can assign the result of when
to a variable or return it from a function.
The example below will assign a String
value to the variable myString
based on the value of myInteger
:
var myInteger: Int = 10
var myString: String = when (myInteger) {
1 -> "one"
2 -> "two"
else -> "another number"
}
print(myString) // "another number"
When you’re using the when
keyword as an expression, you must include the else
statement or Kotlin won’t compile your code.
You can also return a when
expression from a function as shown below:
var myInteger: Int = 1
var myString: String = checkMyInt(myInteger)
fun checkMyInt(myInt: Int): String {
// return a when expression
return when (myInt) {
1 -> "one"
2 -> "two"
else -> "another number"
}
}
print(myString) // "one"
And that’s how you can use the Kotlin when
keyword as an expression.
Specify multiple conditions for a branch
You can specify multiple conditions as the requirements of one specific branch inside the when
keyword.
To do so, you need to separate the conditions using a comma.
The following example shows multiple conditions for the two branches of the when
statement:
var myInteger: Int = 2
when (myInteger){
1, 2 -> print("Value is either one or two")
3, 4 -> print("Value is either three or four")
}
Creating a conditional branch with in keyword
You can use the in
or !in
keyword to check if the when
argument is inside or outside of a range as follows:
when (myInteger){
in 1..5 -> print("Value is between one to five")
!in 6..10 -> print("Value is not between six to ten")
}
The when
keyword evaluates the conditional branches from top to bottom. Kotlin will stop evaluating the next branch after it found the first matching condition.
So although it’s possible for myInteger
to fulfill both conditional branches above, only the first matching condition will be executed.
Checking value type with the is keyword
You can also use the is
and !is
keywords to check the type of the argument passed to the when
keyword.
Take a look at the example below:
var myVar: Any = true
when (myVar){
is Int -> print("Value is an integer")
!is String -> print("Value is NOT a string")
}
You’ve learned how the when
keyword works in Kotlin. Nice work! 👍
For more information, you can check out the Kotlin documentation for the when
keyword.