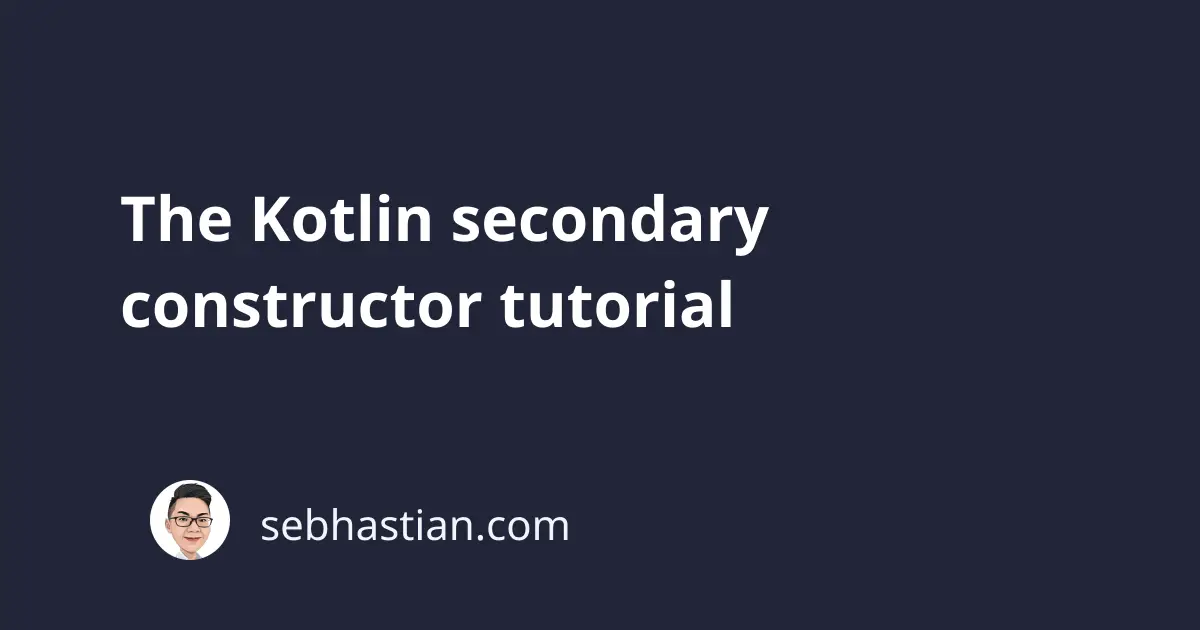
In Kotlin, there are two ways you can create constructors in a class
.
One way is to use a primary constructor, where the class
properties are initialized in the class
header as shown below:
class Person(
val name: String
)
In the above example, the name: String
property of the Person
class is initialized in the primary constructor.
The other way to create a constructor is by defining a secondary constructor.
While a class
primary constructor only allows you to initialize the properties of the class, a secondary constructor allows you to write some logic to execute when an object of the class is initialized.
Consider the following example:
class Person {
constructor(name: String) {
println("Hello, my name is $name")
}
}
Person("Nathan")
// Hello, my name is Nathan
In the above example, a secondary constructor is declared using the keyword constructor()
followed by the constructor body.
It’s similar to how you create a regular function, but this function uses the keyword constructor
instead of fun
and doesn’t have a name (anonymous).
Here’s a Kotlin class
with a primary constructor that’s equal to the example above:
class Person(name: String) {
init {
println("Hello, my name is $name")
}
}
But while a secondary constructor can execute code logic without the init
block, you can’t declare class
properties in a secondary constructor.
The following code will trigger an error:
class Person {
constructor(val name: String, var age: Int) {
println("Hello, my name is $name")
}
}
// Error: 'val' on secondary constructor parameter is not allowed
// Error: 'var' on secondary constructor parameter is not allowed
Without creating a primary constructor, you need to declare the class
properties in the body:
class Person {
var name: String
constructor(name: String) {
this.name = name
println("Hello, my name is $name")
}
}
val person = Person("Nathan")
person.name = "Joy"
The example above shows how it’s quite redundant when you create a secondary constructor without a primary constructor.
That’s why most often, you only need to use the primary constructor and run some logic from the init
block.
You can also add multiple secondary constructors to a single class
as shown below:
class Person {
constructor(name: String) {
println("1st secondary constructor called")
println("Hello, my name is $name")
}
constructor(name: String, age: Int) {
println("2nd secondary constructor called")
println("Hello, my name is $name")
println("I'm $age years old")
}
}
Person("Nathan")
// 1st secondary constructor called...
Person("Joy", 23)
// 2nd secondary constructor called...
Kotlin will decide which constructor
to execute based on the arguments that you send to the class
with multiple secondary constructors.
Finally, you can have a Kotlin class
with both primary and secondary constructors, but with a condition that you call the primary constructor from the secondary constructor.
See the following example:
class Person(val name: String) {
constructor(name: String, age: Int) : this(name) {
println("Secondary constructor called")
}
}
Person("Nathan")
// prints nothing
Person("Naomi", 25)
// Secondary constructor called
The secondary constructor calls the primary constructor through the use of : this(name)
syntax after the secondary constructor’s parameters.
Also, you can’t have two constructors with identical parameters, so the secondary constructor needs to have different parameters.
If this looks confusing to you, then it’s because it is 😄
Creating secondary constructors in a class
gives you more flexibility to define what object
can be created from the class
.
But the tradeoff is that you will increase the complexity of your code, making it harder to maintain and develop.
I’d recommend you use a primary constructor until you have a strong reason to use secondary constructors.
Great work on learning the Kotlin class
secondary constructors! 👍