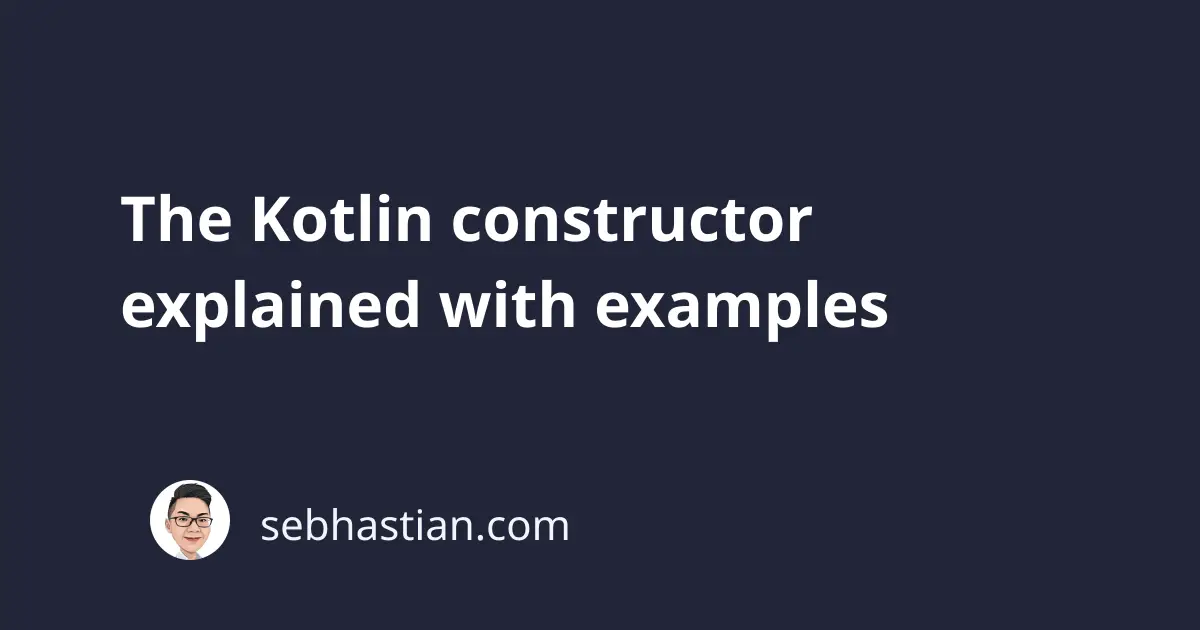
A constructor is a feature of Kotlin class
member that allows you to initialize a class properties in a clear and easy way.
A class
member in Kotlin can have a primary constructor and many secondary constructors. This tutorial will focus on the primary constructor first.
The primary constructor in Kotlin class
is defined in parentheses as a part of the class header.
In the following example, the Person
class has a primary constructor of a string
property called name
:
class Person(val name: String)
When you initialize an object of a Person
class, you need to pass a string
as the name
property of that object.
Consider the following example:
class Person(val name: String)
val person = Person("Jack")
println(person.name) // Jack
The primary constructor can have as many properties as you need according to the class
requirements.
You can add multiple properties in the constructor separated by a comma as follows:
class Person(val name: String, var age: Int, var isAdult: Boolean)
In the above example, the age
and isAdult
properties are initialized using the var
keyword.
A property defined using the val
keyword is a read-only property, while a var
property value can be mutated by the object.
The constructor property can always be called from the initialized object using the property name:
val person = Person("Jack", 27, true)
// modifying the properties
person.age = 14
person.isAdult = false
// calling the properties
println(person.age) // 14
You can also define default values for the constructor properties as shown below:
class Person(val name: String = "Nathan", var age: Int = 29)
When you want to apply access modifiers to the constructor, you need to add the constructor
keyword before the parentheses.
For example, the following class
has a private constructor
:
class Person private constructor(val name: String)
But the most common use of the constructor is to omit the constructor
keyword as in previous examples.
When you have a long constructor, you can move each property definition in its own line.
Consider the following class
example:
class Person(
val name: String = "Nathan",
var age: Int = 29,
var isAdult: Boolean,
var profession: String,
var citizenship: String,
)
This way, the properties will be easier to read than a single long line beside the class name.
Properties initialized in the constructor can be used to initialize variables in the class body:
class Person(
val name: String = "Nathan",
var age: Int = 29,
) {
val customerId = name.uppercase()
}
Finally, a Kotlin primary constructor can only initialize property values without running any statement.
If you want to run any code after the object is initialized, you need to define an initializer block inside the class body using the init
keyword.
Consider the following example:
class Person(
var name: String,
var age: Int,
) {
init {
println("Hello! My name is $name")
println("I am $age years old")
}
}
val person = Person("Nathan", 29)
// Hello! My name is Nathan
// I am 29 years old
person.name = "Jack"
person.age = 27
The init
block above will be called right after the object is initialized, so even when you change the properties of the class, only the initial values are used for the init
code block.
And that’s how the class
constructor works in Kotlin.
Next, let’s learn about Kotlin secondary constructors.