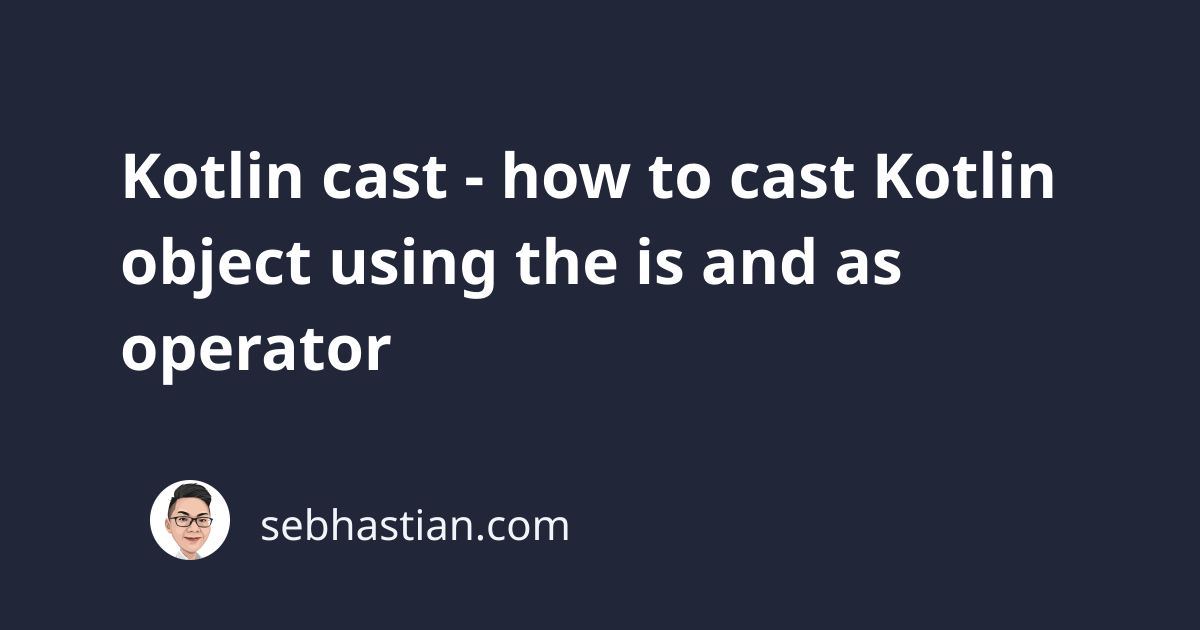
To cast Kotlin objects from one type to another, you can use the is
and as
operator.
The is
operator is used to cast types implicitly while the as
operator is used to cast explicitly.
Let’s learn the differences in this tutorial.
Kotlin implicit casting with the is
operator
The is
operator allows you to check if a variable is of a certain object type, but it also performs an implicit casting so that you can access the properties and methods of the object class that you checked on.
For example, you may have an Any
type variable called myVar
that can accept a String
or an Int
.
You can use the is
operator to create a conditional if
block based on type myVar
value as shown below:
val myVar: Any = "Hello World"
if (myVar is String) {
print(myVar.length)
}
if (myVar is Int) {
print(myVar.plus(5))
}
The call to the .length
property and the .plus()
method above is possible because the is
operator performs a smart-casting when you use it to check on a value type.
Because the "Hello World"
is a String
type, the is
operator implicitly converts the Any
value to String
value, enabling you to access String
class properties and methods.
When you put an integer number as the value of myVar
above, a conversion to Int
happens when you use the is
operator to check whether the variable value is of Int
type.
You can also call the new object type properties and methods when you create multiple conditions inside your if
block.
For example, suppose you need the variable length
property to be greater than 0
.
You can check on the length
property in the same line where you add the is
operator as shown below:
val myVar: Any = "Hello World"
if (myVar is String && myVar.length != 0) {
print(myVar.length)
}
The Kotlin is
operator behavior allows you to reduce the amount of code you need to write when compared with its Java equivalent as shown below:
// Java
Object myVar = "Hello World";
if (myVar instanceof String && ((String) myVar).length() != 0) {
System.out.println(((String) myVar).length());
}
The Java version is longer and requires you to cast the Object
type variable twice: when you check the length
property value and when you print it.
And that’s how the is
operator allows you to implicitly cast Kotlin types. Let’s learn about explicit casting next.
Kotlin explicit casting with the as
operator
The as
operator is used to explicitly convert an object to another type when possible.
For example, you may explicitly cast an Any
type variable to a String
type as shown below:
val myVar: Any = "Hello World"
var myStr: String = myVar as String
print(myStr.length) // 11
But since as
operator is an explicit convert, Kotlin will throw a ClassCastException
error when you try to cast an Int
type as a String
type:
val myVar: Any = 9
var myStr: String = myVar as String
// Exception in thread "main" java.lang.ClassCastException:
// class java.lang.Integer cannot be cast to class java.lang.String
To perform a safe explicit cast, you need to use the nullable cast as?
operator.
When the variable can’t be converted to the specified type, Kotlin will return null
instead of throwing an error:
val myVar: Any = 9
var myStr: String? = myVar as? String
print(myStr) // null
And that’s how you can cast object types with Kotlin.
Kotlin also has type conversion methods for type classes that you can use to convert value types.
Learn more: Kotlin type conversion methods explained.
Thank you for reading! 🙏